jQuery find events handlers registered with an object
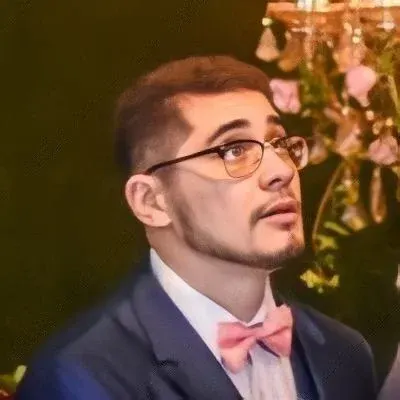
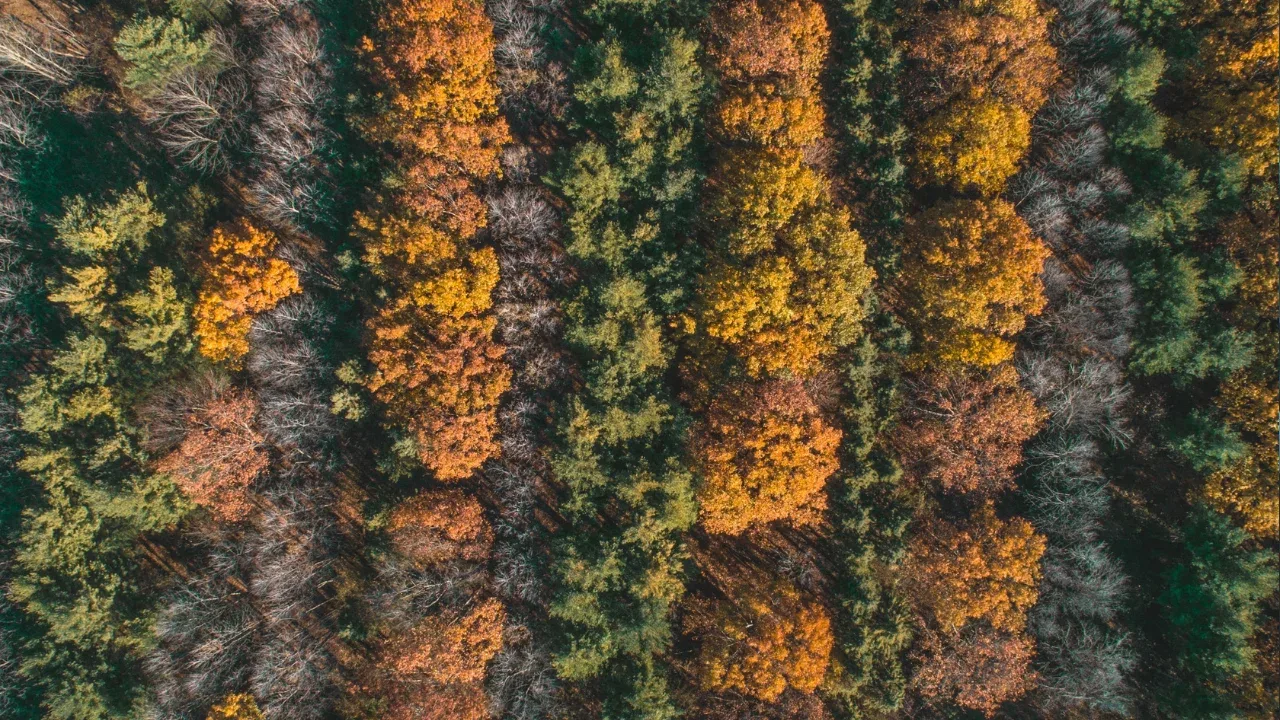
📝 The Ultimate Guide to Finding Event Handlers Registered with jQuery Objects
Introduction
So, you have a jQuery object and you want to find out which event handlers are registered with it? You've come to the right place! In this guide, we'll address this common issue and provide easy solutions to help you achieve your goal.
The Problem
Let's start by understanding the problem at hand. The following code snippet sets up two event handlers, click
and mouseover
, for the #el
element:
$("#el").click(function() {...});
$("#el").mouseover(function() {...});
The question is: How can we find out which event handlers are registered with the $("#el")
object?
Solution: Using jQuery._data()
To find the event handlers registered with a jQuery object, we can utilize the jQuery._data()
method. This method allows you to retrieve the data associated with a DOM element, including event handlers.
Let's look at an example:
const eventHandlers = jQuery._data($("#el")[0], "events");
console.log(eventHandlers);
In the above code, we access the first plain DOM object from the jQuery object $("#el")
using the [0]
index. Then, we pass the DOM object and the string "events"
to the jQuery._data()
method. This will give us an object that contains all the event handlers associated with the element.
Solution: Using the unbind()
Method
Another approach to finding event handlers is to temporarily unbind them using the unbind()
method. This method removes the specified event handlers from the selected elements.
Let's see how it works:
const eventHandlers = {};
$("#el").unbind().each(function() {
const currentHandlers = jQuery._data(this, "events");
if (currentHandlers) {
eventHandlers[$(this).attr("id")] = Object.keys(currentHandlers);
}
});
console.log(eventHandlers);
In the code above, we use the unbind()
method to remove all event handlers from the $("#el")
element. Then, we iterate over the elements using the each()
method. Inside the iteration, we use the jQuery._data()
method to check if there are any remaining event handlers. If so, we store them in the eventHandlers
object, along with the element's ID.
Solution for Plain DOM Objects
But what if you don't have a jQuery object at hand and only have a plain DOM object? No worries! We've got you covered.
You can still use the jQuery._data()
method with plain DOM objects. Here's how:
const element = document.getElementById("el");
const eventHandlers = jQuery._data(element, "events");
console.log(eventHandlers);
In the code snippet above, we use document.getElementById()
to retrieve the DOM element with the ID "el". Then, we pass this element and the string "events" to the jQuery._data()
method to find the associated event handlers.
Conclusion
Congratulations, you've learned how to find event handlers registered with jQuery objects! Whether you choose to use the jQuery._data()
method or the unbind()
method, you now have the tools to solve this problem with ease.
Remember, understanding and manipulating event handlers is an important aspect of web development, and this knowledge can come in handy when debugging or optimizing your code.
So, next time you're faced with the question of finding event handlers, be confident that you can handle it like a pro!
If you found this guide helpful, feel free to share it with your fellow developers. And don't forget to leave a comment below and let us know your thoughts and any questions you may have. Happy coding! 😊✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
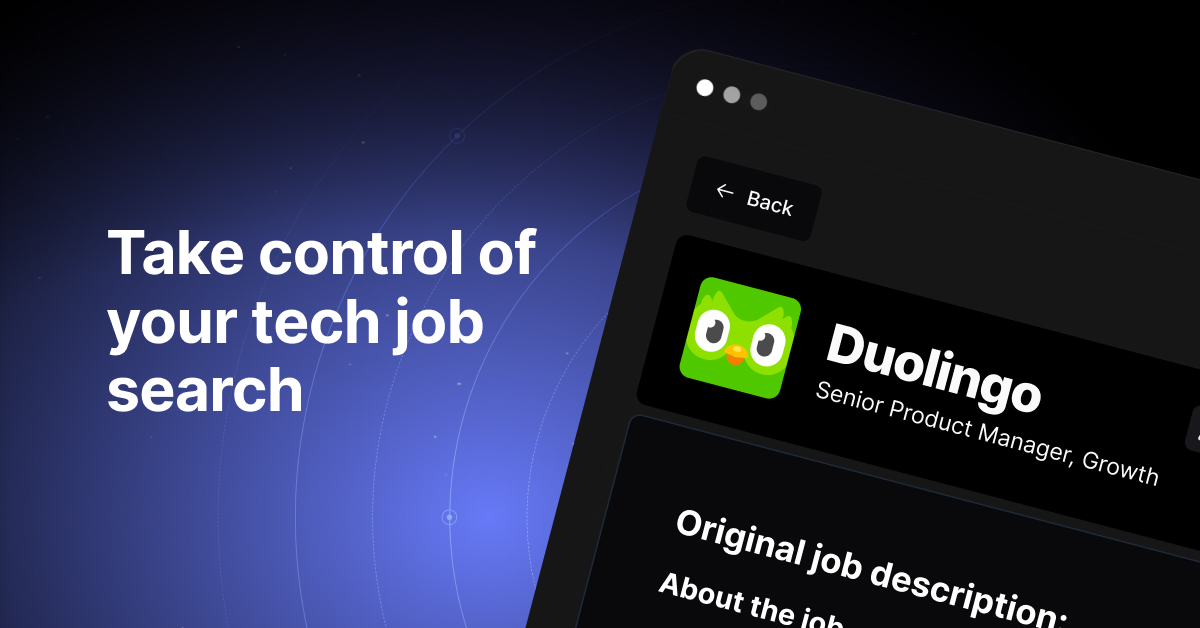