How to use jQuery with Angular?
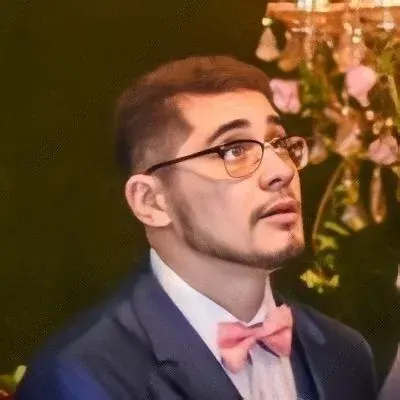
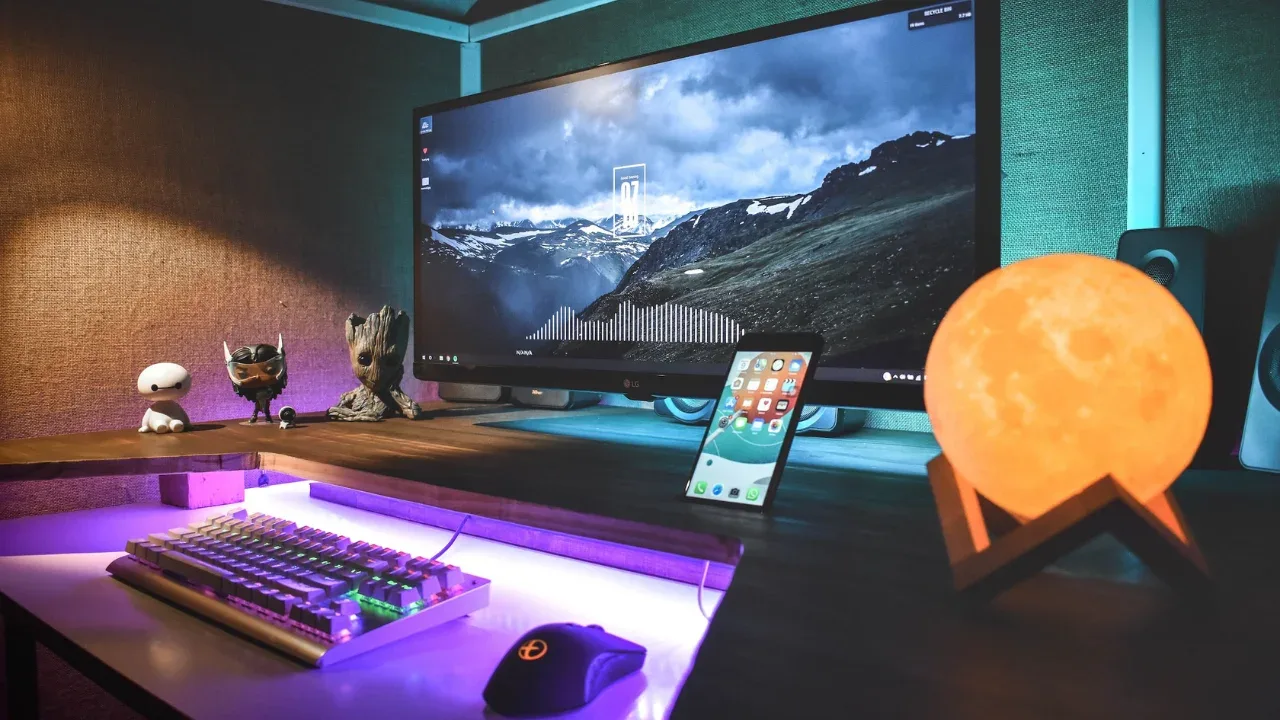
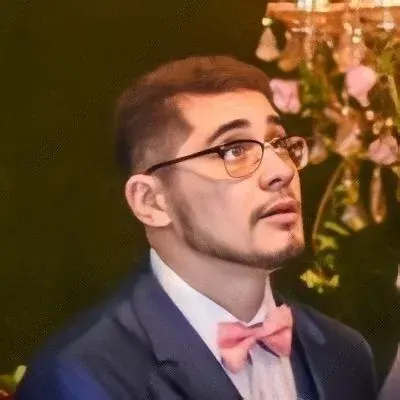
🤔 How to Use jQuery with Angular?
Hey there techheads! Are you scratching your head, trying to figure out how to use jQuery with Angular? Don't worry, we've got you covered! 💪
So, let's dive into the problem statement:
📃 The Problem
A fellow developer asked, "Can anyone tell me, how to use jQuery with Angular?" They provided the following code snippet:
class MyComponent {
constructor() {
// how to query the DOM element from here?
}
}
While some workarounds involve manipulating the class or ID of the DOM element upfront, we're on a mission to discover a cleaner way to tackle this!
💡 The Solution
Now, let's explore a couple of easy solutions to integrate jQuery seamlessly with Angular.
Solution 1: Incorporating jQuery using Angular's ElementRef
One way to leverage jQuery within Angular is by using the ElementRef
class. Let's update our code:
import { ElementRef } from '@angular/core';
class MyComponent {
constructor(private elementRef: ElementRef) {
// how to query the DOM element from here?
const element = this.elementRef.nativeElement; // get the native DOM element
$(element).addClass('myClass'); // use jQuery functions on it
}
}
In this solution, we import ElementRef
from '@angular/core'
. Then, we pass it in the constructor and assign it to a local variable. By calling nativeElement
on the elementRef
, we retrieve the actual DOM element, which can then be manipulated using jQuery's powerful functions!
Solution 2: Utilizing Angular's Renderer2
Another approach involves utilizing Angular's Renderer2
module. Let's modify our code once again:
import { Renderer2, ElementRef } from '@angular/core';
class MyComponent {
constructor(private renderer: Renderer2, private elementRef: ElementRef) {
// how to query the DOM element from here?
const element = this.elementRef.nativeElement;
this.renderer.addClass(element, 'myClass'); // leverage Renderer2 for DOM manipulation
}
}
Here, we import Renderer2
and ElementRef
from '@angular/core'
. Within the constructor, we assign both renderer
and elementRef
to respective class properties. By using the addClass
method from renderer
, we can add our desired class to the element.
🙌 Wrap Up
Congrats, you've learned two simple ways to integrate jQuery with Angular! 🎉
Before wrapping up, it's important to note that using jQuery with Angular should be kept to a minimum. Angular's powerful features such as data binding and event handling should be preferred over jQuery's direct DOM manipulation whenever possible. Remember, Angular's goal is to provide a more structured and scalable framework! 😉
If you have any further questions, feel free to drop them in the comments section below. Happy coding! 💻
🔗 Don't forget to share this post with your fellow developers to help them conquer the jQuery + Angular conundrum!
Stay tuned for more tech-savvy content! 🚀