How to send a PUT/DELETE request in jQuery?
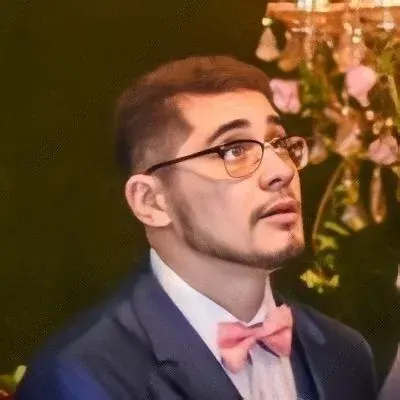
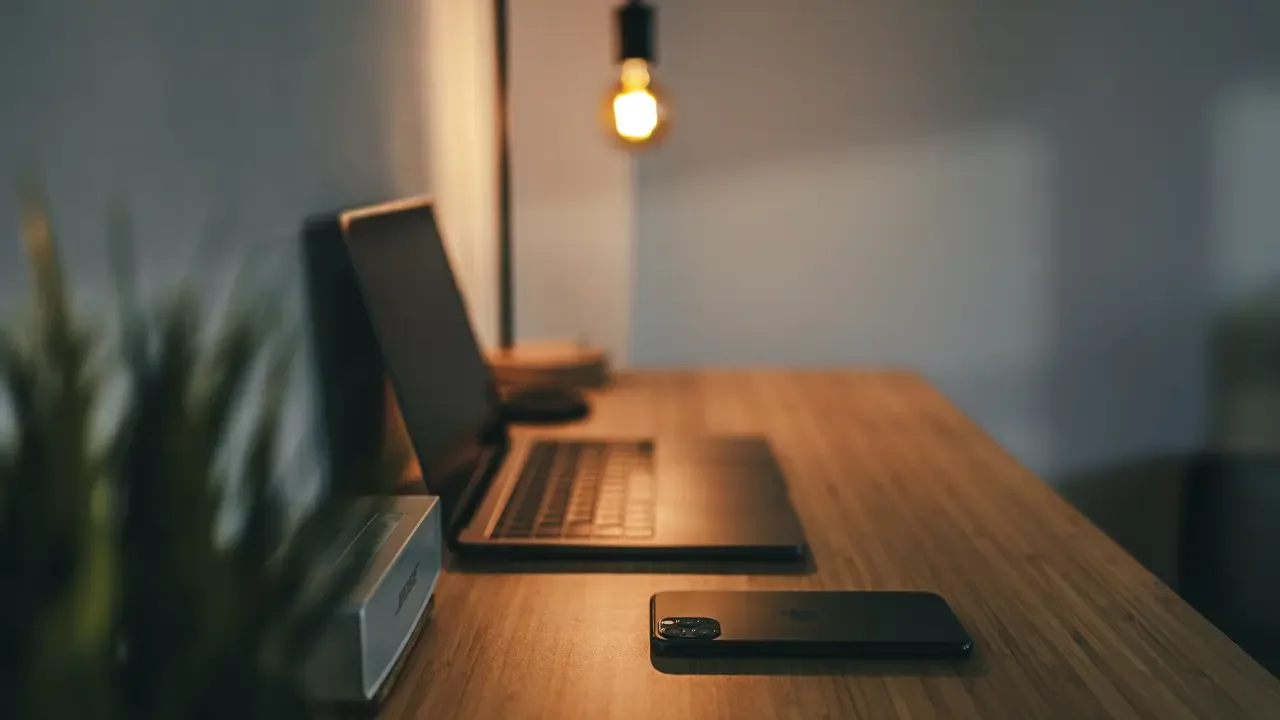
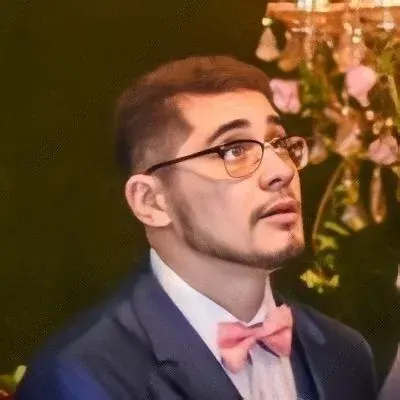
How to Send a PUT/DELETE Request in jQuery? 💪🔀❌
If you've been using jQuery to make AJAX requests, you might be familiar with the $.get()
and $.post()
methods for sending GET and POST requests, respectively. 📥📤
But what about sending PUT and DELETE requests in jQuery? 🤔🤷
It turns out that jQuery doesn't provide dedicated methods for these HTTP methods out of the box. However, fear not! There are easy workarounds to accomplish this. Let's dive in! 🏊♂️💻
The Problem ❌
To send a PUT or DELETE request, you need to set specific headers, provide the request body (if necessary), and handle any potential errors. This can be a bit trickier compared to GET and POST requests with jQuery. 😓
Solution 1: Using $.ajax() ⚙️✉️
One way to send a PUT or DELETE request is by using the versatile $.ajax()
method. This method allows you to customize your AJAX request with the necessary HTTP method, headers, and data. 🛠️👨💻
Here's an example of sending a PUT request using $.ajax()
:
$.ajax({
url: '/api/resource',
method: 'PUT',
data: {
name: 'John Doe',
age: 30
},
success: function(response) {
console.log('PUT request succeeded!', response);
},
error: function(xhr, status, error) {
console.log('PUT request failed!', error);
}
});
Similarly, you can send a DELETE request by setting the method
parameter to 'DELETE'
.
Solution 2: Extending jQuery with $.put() and $.delete() 💪✂️
If you find yourself frequently sending PUT or DELETE requests, you might prefer a more concise approach. We can extend jQuery by creating custom methods to handle these HTTP methods. 💡
Here's an example of how you can extend jQuery to include $.put()
and $.delete()
methods:
$.put = function(url, data, success, dataType) {
return $.ajax({
url: url,
method: 'PUT',
data: data,
success: success,
dataType: dataType
});
};
$.delete = function(url, success, dataType) {
return $.ajax({
url: url,
method: 'DELETE',
success: success,
dataType: dataType
});
};
Now, you can send PUT and DELETE requests in a more straightforward manner:
$.put('/api/resource', { name: 'John Doe', age: 30 })
.done(function(response) {
console.log('PUT request succeeded!', response);
})
.fail(function(xhr, status, error) {
console.log('PUT request failed!', error);
});
$.delete('/api/resource')
.done(function(response) {
console.log('DELETE request succeeded!', response);
})
.fail(function(xhr, status, error) {
console.log('DELETE request failed!', error);
});
Final Thoughts and Call to Action 📝🤝
Sending PUT and DELETE requests in jQuery requires a bit more effort than GET and POST requests. However, with the help of the $.ajax()
method or by extending jQuery with custom methods, you can achieve the desired functionality. 💪🔀❌
Now, you have the power to send PUT and DELETE requests effortlessly in your jQuery projects! Give it a try and let us know your thoughts! 😎🚀
Have you encountered any other challenges with jQuery AJAX requests? Share your experiences and insights in the comments below! Let's level up our AJAX game together! 🙌🎮