How to remove specific value from array using jQuery
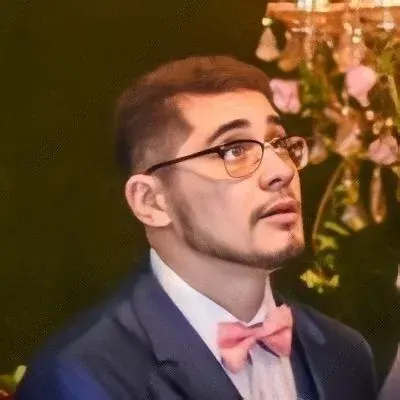
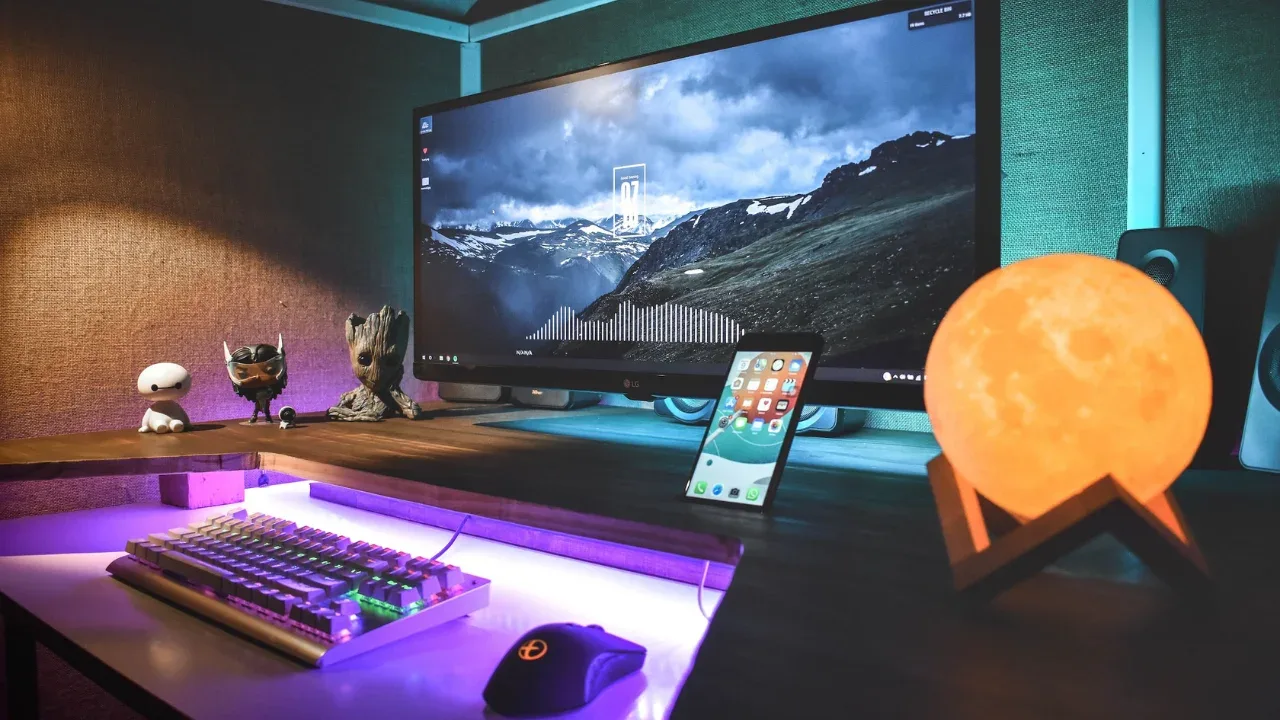
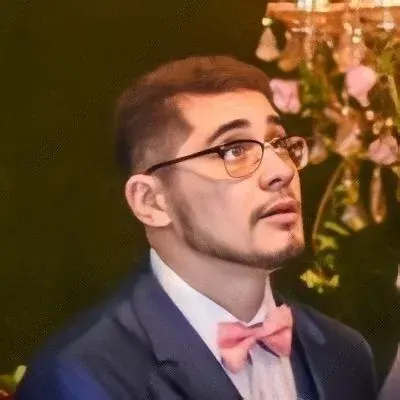
Removing a Specific Value from an Array Using jQuery
So you have an array, and you want to remove a specific value from it using jQuery. You've tried the pop()
method, but that only removes the last element. Don't worry, I've got you covered! 🤩
The Problem
Let's say you have this array:
var y = [1, 2, 3];
And you want to remove the value 2
from it. How can you do that? Well, the good news is that jQuery provides some handy methods to manipulate arrays. 🙌
The Solution
To remove a specific value from an array using jQuery, you can use the $.grep()
function. This function allows you to filter out elements from an array based on a condition.
Here's how you can use $.grep()
to remove 2
from the y
array:
y = $.grep(y, function(value) {
return value != 2;
});
In the above code, $.grep()
takes two arguments: the array y
and a function that defines the condition for filtering. The function checks each element in the array and returns true
if the element should be kept, or false
if it should be removed.
In our case, the condition value != 2
checks if the element is not equal to 2
. If the condition is true
, the element is kept; otherwise, it is removed from the array.
After running the code, the y
array will now look like this:
[1, 3]
And just like that, you have successfully removed the specific value 2
from the array! 🎉
Be Aware
Keep in mind that $.grep()
creates a new array with the filtered elements. If you have other references to the original array, they will not be affected by this operation. So make sure to update the array reference if needed.
Conclusion
Removing specific values from an array using jQuery is made easy with the $.grep()
function. Remember to define the condition in the filtering function and update the array reference accordingly. Now you have a powerful tool in your arsenal for manipulating arrays in JavaScript! 💪
So go ahead and give it a try! And if you have any questions or alternative solutions, I would love to hear from you in the comments below. Let's level up our JavaScript game together! 😊🚀