How to get URL parameter using jQuery or plain JavaScript?
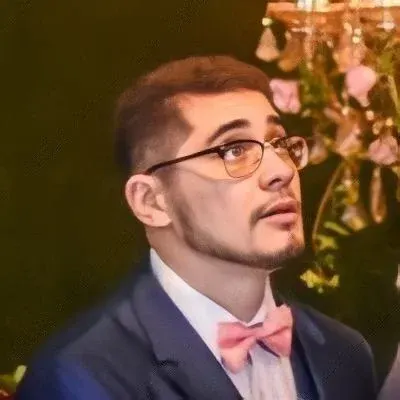
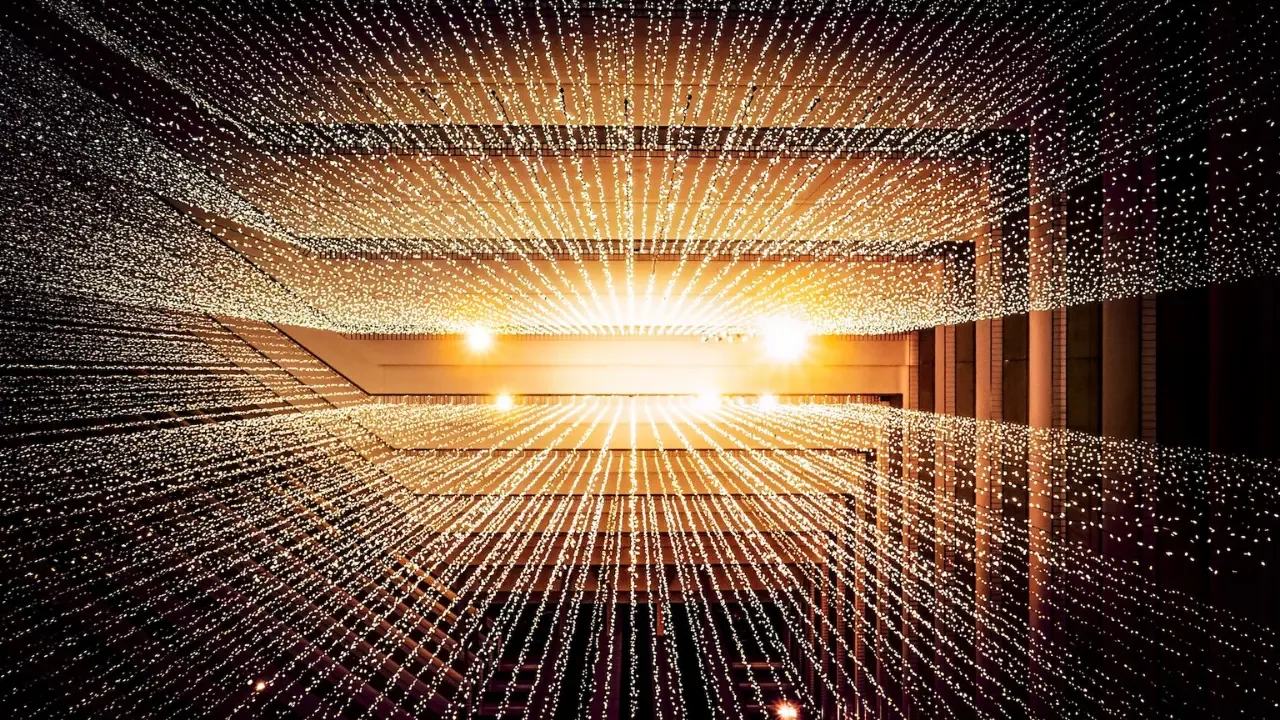
How to Get URL Parameters Using jQuery or Plain JavaScript
Are you struggling to get URL parameters in your web application? Do you want an easy solution to extract specific parameters from a URL using either jQuery or plain JavaScript? Look no further! In this blog post, we'll explore how to detect and extract URL parameters in a simple and efficient way.
The Challenge
One of the common challenges faced by developers is handling URL parameters, especially when their size and names are unknown. To illustrate this, let's consider the following scenario:
You have a URL that looks like this: http://example.com?sent=yes
Your goal is to detect the existence of the sent
parameter and check if its value is equal to "yes".
Using jQuery
If you're using jQuery in your project, you can leverage its built-in functionality to easily extract URL parameters. Here's how you can do it:
// Get URL Parameters in jQuery
function getParameter(url, parameter) {
var urlParams = new URLSearchParams(url.search);
return urlParams.get(parameter);
}
// Usage
var url = new URL("http://example.com?sent=yes");
var sentParam = getParameter(url, "sent");
if (sentParam === "yes") {
// Hooray! The "sent" parameter exists and its value is "yes"
} else {
// Oops! Something went wrong
}
In the above example, we define a getParameter
function that takes the URL object and the parameter name as arguments. We then use the URLSearchParams
constructor to parse the URL's search parameters. Finally, we use the get
method to retrieve the value of the specified parameter.
Using Plain JavaScript
If you prefer using plain JavaScript without any libraries, fret not! You can still achieve the desired result using plain JavaScript methods. Here's how you can do it:
// Get URL Parameters in Plain JavaScript
function getParameter(url, parameter) {
var searchParams = new URLSearchParams(url.search);
return searchParams.get(parameter);
}
// Usage
var url = new URL("http://example.com?sent=yes");
var sentParam = getParameter(url, "sent");
if (sentParam === "yes") {
// Hooray! The "sent" parameter exists and its value is "yes"
} else {
// Oops! Something went wrong
}
Similar to the jQuery approach, we define a getParameter
function that takes the URL object and the parameter name as arguments. We create a URLSearchParams
object to parse the URL's search parameters and use the get
method to retrieve the value of the specified parameter.
Engage with the Blog Post
Did you find this guide helpful in extracting URL parameters using jQuery or plain JavaScript? Are there any other web development challenges you're facing that you'd like us to address? Let us know in the comments below!
Remember, understanding how to work with URL parameters is crucial for building dynamic web applications. By mastering this skill, you'll be able to create personalized and tailored experiences for your users.
So, what are you waiting for? Start implementing these techniques in your projects today, and take your web development skills to the next level!
🚀 Happy Coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
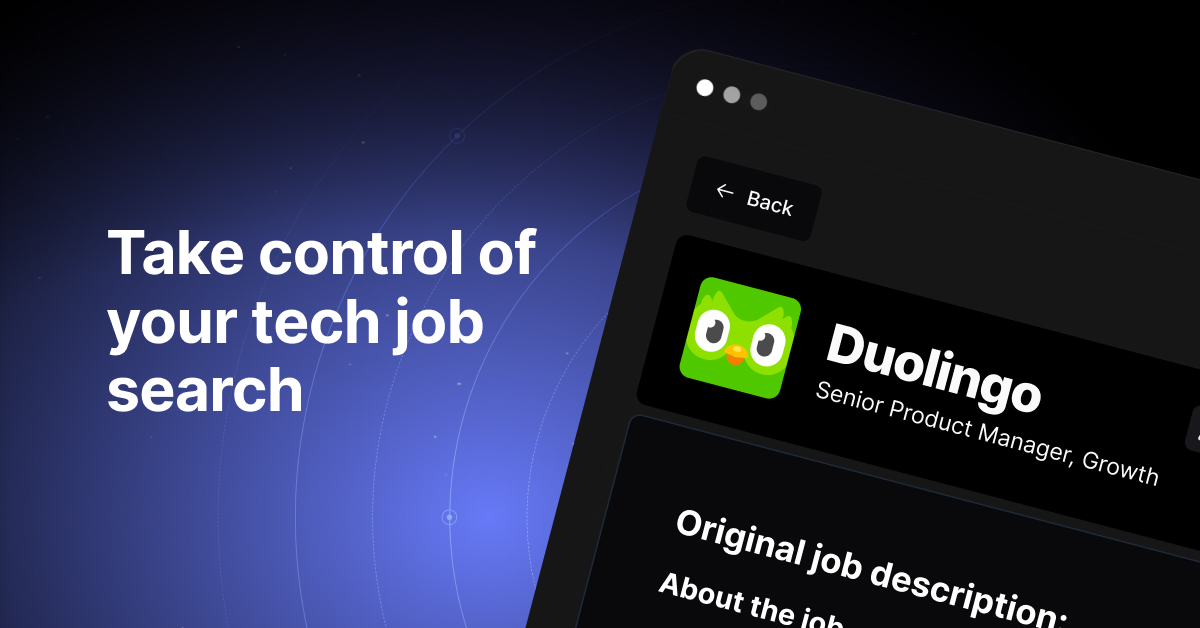