How do I catch an Ajax query post error?
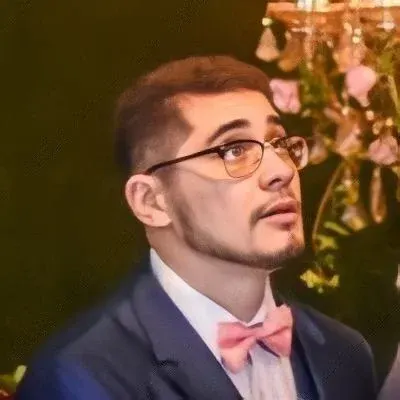
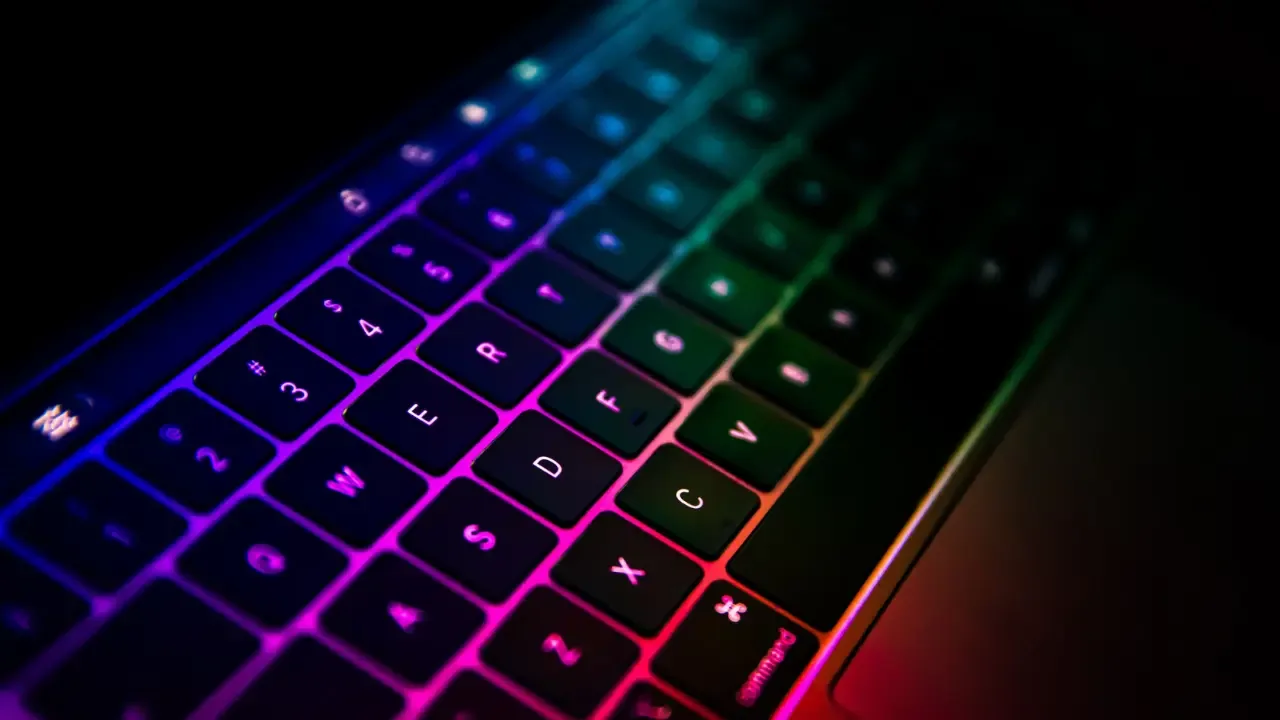
How to Catch an Ajax Query Post Error
So, you're trying to catch those pesky Ajax query post errors, huh? Don't worry, we've got your back! In this guide, we'll walk you through the common issues you might encounter and provide you with easy solutions to catch those errors. 😎
Understanding the Problem
Let's start by looking at the code snippet you provided. It seems like you're making an Ajax post request using jQuery's $.post()
method. You're aiming to handle the situation where the Ajax request fails and display an appropriate error message. However, you mentioned that the "Error in Ajax" code block never gets executed when the request fails.
The Solution – Error Callback
To catch errors in your Ajax request, you need to utilize the $.post()
method's error callback. This callback function is triggered when the request encounters an error. Here's how you can modify your code to handle the error and display the appropriate message:
function getAjaxData(id) {
$.post("status.ajax.php", {deviceId : id}, function(data){
// Code to handle successful response
var tab1;
if (data.length > 0) {
tab1 = data;
} else {
tab1 = "Error in Ajax";
}
// Do something with tab1 (e.g., update UI)
}).fail(function() {
// Code to handle Ajax error
var tab1 = "Error in Ajax";
// Display error message (e.g., show an alert)
alert(tab1);
});
}
By attaching the fail()
method to the $.post()
call, you provide a function that will be executed if the Ajax request encounters an error. In the provided example, the error message "Error in Ajax" is assigned to the tab1
variable, and an alert is shown. You can customize this error handling code to suit your needs, whether it's displaying an error message, logging the error, or performing any other action.
Call to Action
That's it! You have learned how to catch an Ajax query post error like a pro. Go ahead, give it a try, and let us know in the comments how it worked for you. If you have any questions or need further assistance, feel free to ask. Happy coding! 🚀
Have you ever encountered an Ajax query post error? Share your experiences in the comments below!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
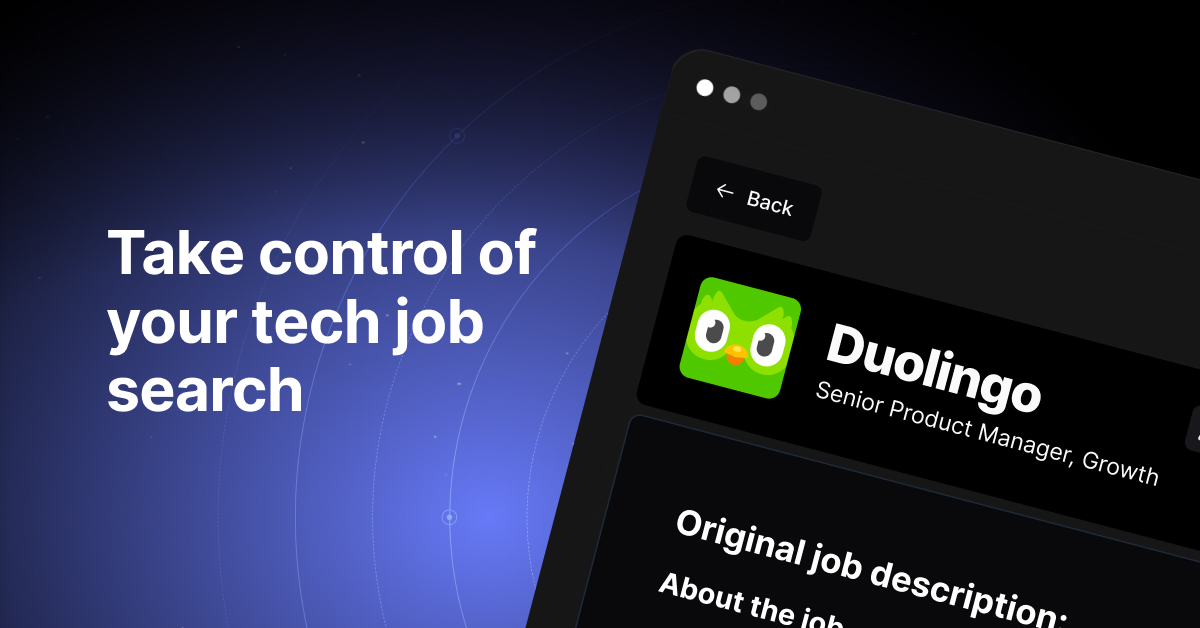