How do I add a simple jQuery script to WordPress?
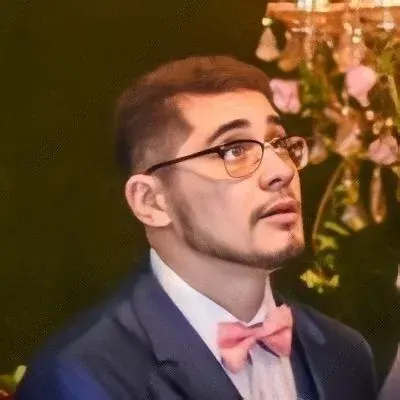
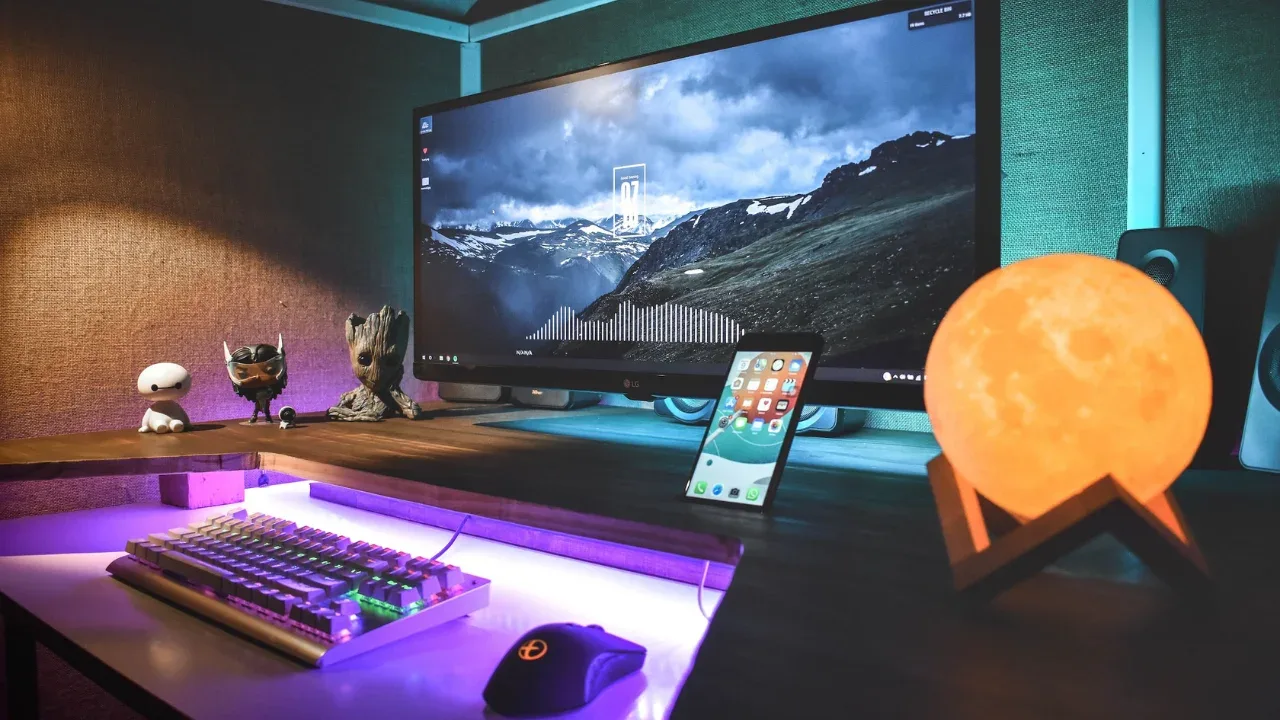
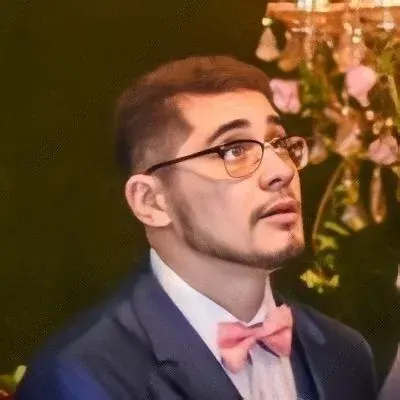
How to Add a Simple jQuery Script to WordPress?
š Have you ever wanted to add a cool jQuery script to your WordPress website, only to feel frustrated by confusing guides and assumptions about your WordPress skills? You're not alone! Adding a jQuery script to WordPress doesn't have to be a headache. In this guide, I will walk you through the process step-by-step, from loading jQuery to adding the script to a single WordPress page. Let's get started! š
Loading jQuery in functions.php
The first step is to load jQuery in your WordPress theme's functions.php
file. This ensures that jQuery is available for use throughout your website. Simply open the functions.php
file in your theme's directory and add the following code:
function mytheme_enqueue_scripts() {
wp_enqueue_script('jquery');
}
add_action('wp_enqueue_scripts', 'mytheme_enqueue_scripts');
š” Pro Tip: Make sure you don't already have this code in your functions.php
file. Duplicate code can cause conflicts and unexpected behavior.
Adding Your jQuery Script
Once you have jQuery loaded, it's time to add your own jQuery script. For the purpose of this guide, let's say you want to add a script that changes the background color of a specific element on a single WordPress page.
Identify the page where you want to add the script.
Open the
functions.php
file in your theme's directory.Add the following code to load your custom jQuery script:
function mytheme_enqueue_custom_script() {
if (is_page('your-page-slug')) { // Replace 'your-page-slug' with the actual slug of your target page
wp_enqueue_script('custom-script', get_template_directory_uri() . '/js/custom-script.js', array('jquery'));
}
}
add_action('wp_enqueue_scripts', 'mytheme_enqueue_custom_script');
š” Pro Tip: You can customize the script's file path and name to match your preferences. Just make sure the file is located in a js
directory within your WordPress theme.
Create a new file called
custom-script.js
in thejs
directory of your theme.Inside
custom-script.js
, add your jQuery code:
jQuery(document).ready(function($) {
// Your jQuery code here
$('element-selector').css('background-color', 'red');
});
š” Pro Tip: Replace 'element-selector'
with the actual selector of the element you want to modify. You can use class, ID, or any other valid selectors.
Testing Your jQuery Script
Once you have added your jQuery script, it's time to test it!
Go to the WordPress page where you added the jQuery script.
Open the browser's developer console (usually by right-clicking and selecting "Inspect" or "Inspect Element").
Navigate to the "Console" tab in the developer console.
If everything is set up correctly, you should see no errors in the console log.
Refresh the page and see the magic happen!
Share Your Success!
Congratulations! š You have successfully added a simple jQuery script to your WordPress website. Now, it's time to show off your new skills and engage with the community. Share your success story or any questions you may have in the comments below. Let's learn and grow together! šāØ
⨠Remember, adding jQuery scripts to WordPress doesn't have to be frustrating. With this step-by-step guide, you can confidently enhance your website with awesome interactive features. Happy coding! šš