How can I post an array of string to ASP.NET MVC Controller without a form?
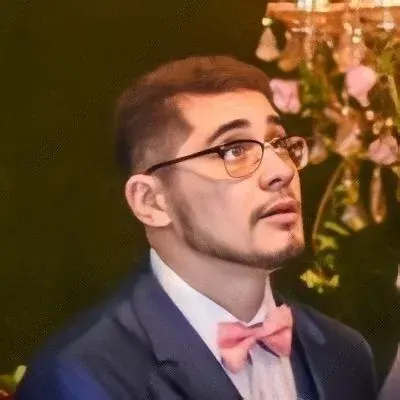
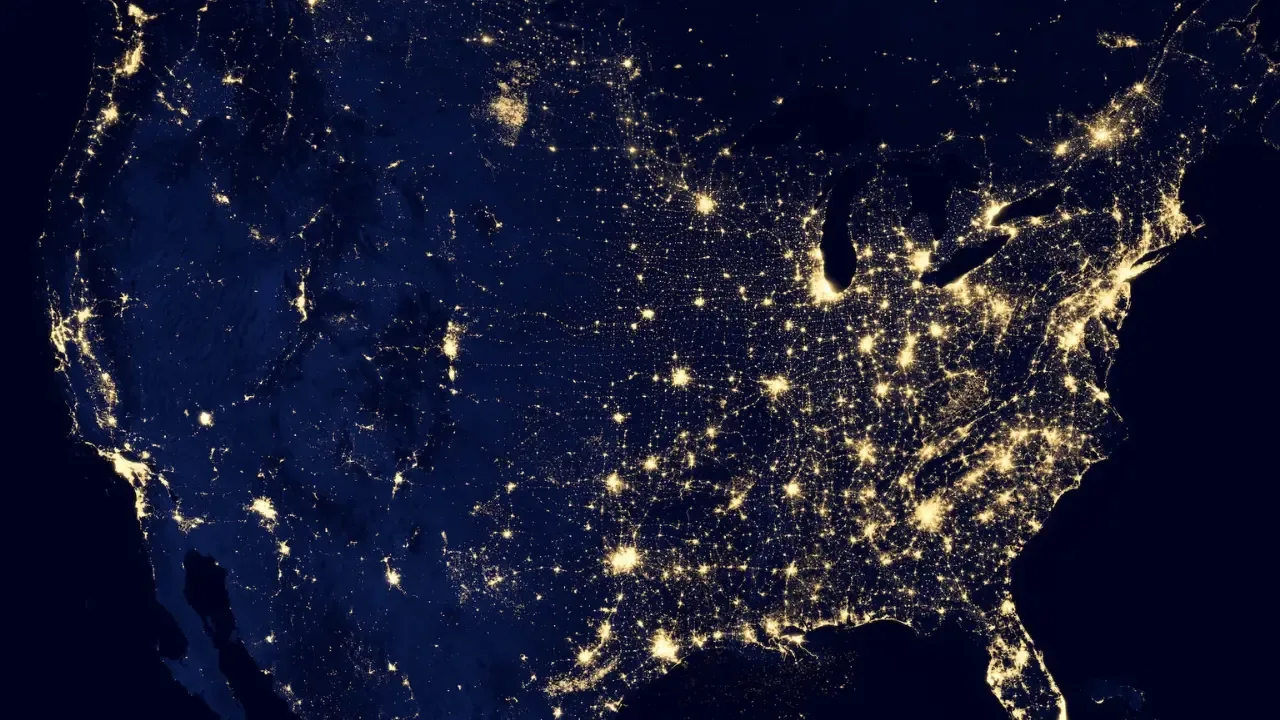
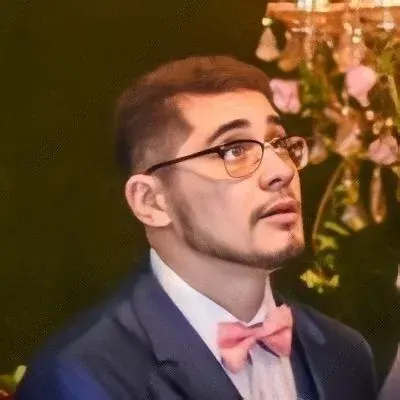
How to Post an Array of Strings to an ASP.NET MVC Controller without a Form
So, you're working on a cool ASP.NET MVC app and you want to learn some JQuery magic to send an array of strings to your controller without using a form. 🚀
You've already managed to grab the array of selected items' ids, and now you're scratching your head trying to figure out how to send them directly to your controller without adding unnecessary HTML code to your page. 🤔
Don't worry, my friend! I've got you covered with some easy solutions that'll make your code clean and efficient. Let's dive in! 💪
The Problem
The issue you're facing is that your controller receives a "null" value when you try to send the array to it using the $.post
function. This can be quite frustrating, but fear not, there's a workaround!
Solution 1: Use JSON Data
One clean way to achieve this is by sending the array as JSON data directly to your controller. You just need to make a small adjustment to your existing code. Here's how it will look:
function generateList(selectedValues) {
var s = {
values: selectedValues // selectedValues is an array of strings
};
$.post("/Home/GenerateList", JSON.stringify(s), function() { alert("back") }, "json");
}
In this updated code, we're using JSON.stringify
to convert the JavaScript object s
into a JSON string. This ensures that our array of strings is sent as expected to the controller.
Solution 2: Specify the Data Type
Another solution is to explicitly specify the data type in your $.post
request. By default, jQuery assumes that the data being sent is in the default format (e.g., URL-encoded). However, since we're sending JSON data, we need to inform jQuery about this. Here's how you can do it:
function generateList(selectedValues) {
var s = {
values: selectedValues // selectedValues is an array of strings
};
$.post({
url: "/Home/GenerateList",
data: JSON.stringify(s),
dataType: "json",
contentType: "application/json"
}).done(function() {
alert("back");
});
}
In this updated code, we're using the contentType
property to inform jQuery that we're sending JSON data. Additionally, we're using the dataType
property to specify that we expect the response from the server to be in JSON format.
Marvelous Result
Congratulations! By using either of these solutions, you will no longer receive a "null" value in your controller parameter. 🎉
Now you can handle the array of strings as desired in your GenerateList
action method within the controller.
The Call-to-Action
I hope this guide has helped you solve the issue of posting an array of strings to your ASP.NET MVC controller without using a form. 🙌
Go ahead and implement these solutions in your code, and don't forget to share your success story in the comments section below! Feel free to ask any further questions as well. Let's learn and grow together! 🤗