How can I change CSS display none or block property using jQuery?
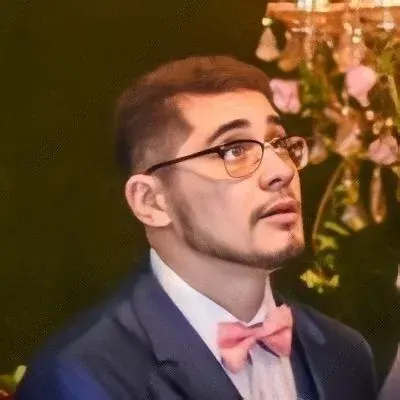
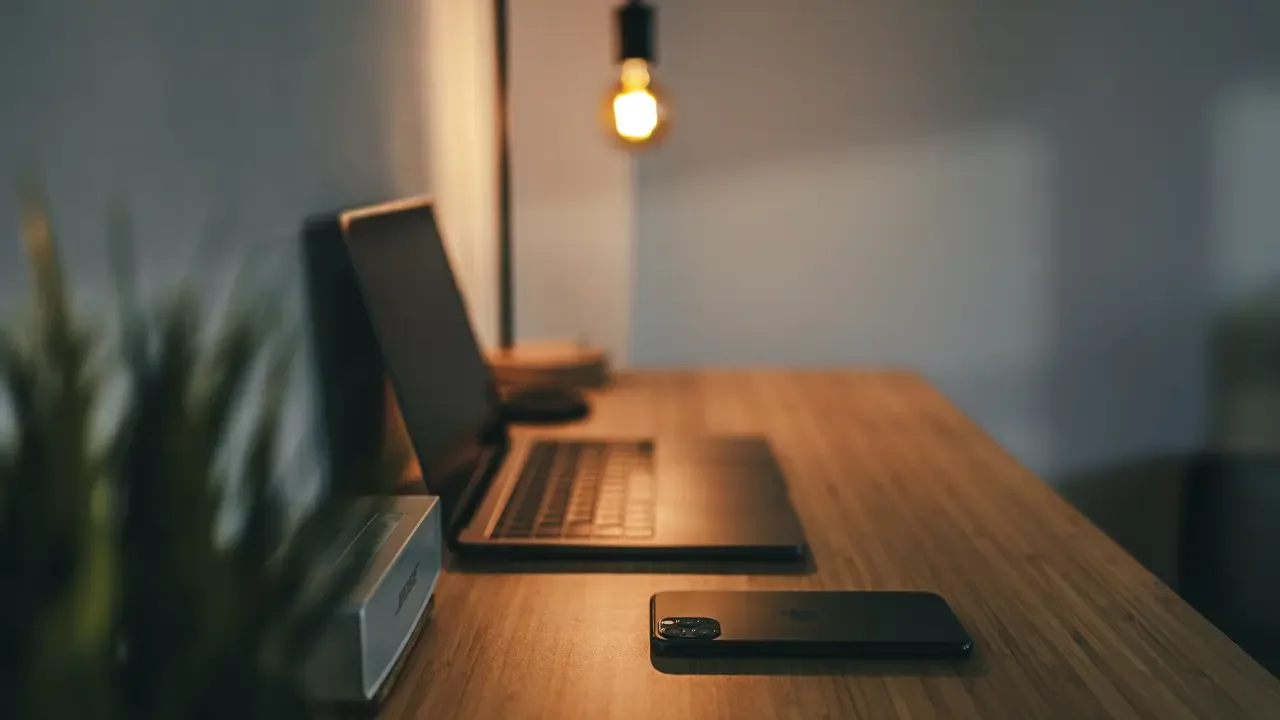
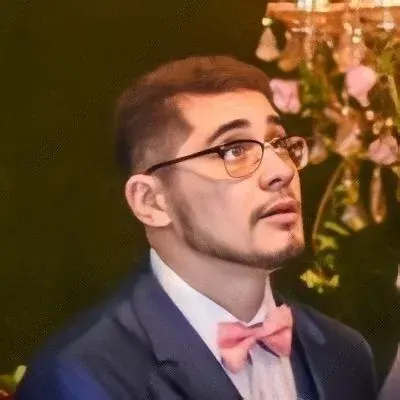
💻 The Ultimate Guide: Changing CSS Display Property with jQuery
Are you struggling to manipulate the CSS display property in your web projects? Well, fear no more! In this blog post, we will dive deep into the world of jQuery and show you how to easily change the "display" property from "none" to "block" and vice versa. 🎉
Identifying the Problem:
So, you have an element on your web page that you want to show or hide dynamically. Maybe it's a dropdown menu, a modal window, or a loading spinner. But how can you achieve this without messing around with complicated CSS classes or inline styles? 🤔
The jQuery Magic:
Here comes the superhero of web development, jQuery, to rescue the day! Using this powerful JavaScript library, you can effortlessly change the CSS "display" property with just a few lines of code.
Solution 1: Toggle between Display States:
The easiest and most common way to change the CSS display property is by using the toggle()
function. This function will toggle between "none" and "block" states each time it is called.
$("#elementID").toggle(); // Replace 'elementID' with the ID of your element
Simply attach this code to an event, such as a button click or a form submission, and watch the magic happen! The targeted element will gracefully appear or disappear with a satisfying animation. 🎩
Solution 2: Show and Hide:
If you prefer a bit more control over how the element appears or disappears, jQuery provides two separate functions: show()
and hide()
.
Show:
$("#elementID").show(); // Replace 'elementID' with the ID of your element
Hide:
$("#elementID").hide(); // Replace 'elementID' with the ID of your element
These functions will instantly show or hide the specified element without any fancy animations. Perfect for those who want simplicity and speed!
Solution 3: CSS Method:
If you're feeling adventurous or need to change the CSS display property to something specific, jQuery also offers the css()
method.
$("#elementID").css("display", "block"); // Replace 'elementID' with the ID of your element
By calling the css()
function and passing in the desired CSS property and value, you have full control over how the element is displayed. 🖌️
💡 Bonus Tips:
Don't forget to include the jQuery library in your HTML file using the
<script>
tag. You can use a local copy or include it from a Content Delivery Network (CDN) like so:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
If you're working with multiple elements, you can use classes instead of IDs. Just replace
#elementID
with.elementClass
.
Get Creative!
Now that you know the secret sauce for changing the CSS display property, go ahead and get creative with your web projects! Show or hide elements dynamically, dazzle your users with sleek animations, and watch as your website becomes a powerhouse of interactivity. 💪
📣 Your Turn!
We hope you found this guide helpful! Now it's your turn to share your thoughts. Have you ever struggled with manipulating the CSS display property? How did jQuery change the game for you? Comment below and let's start a conversation! 👇