Get selected element"s outer HTML
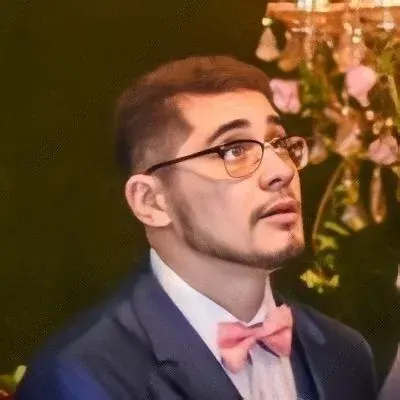
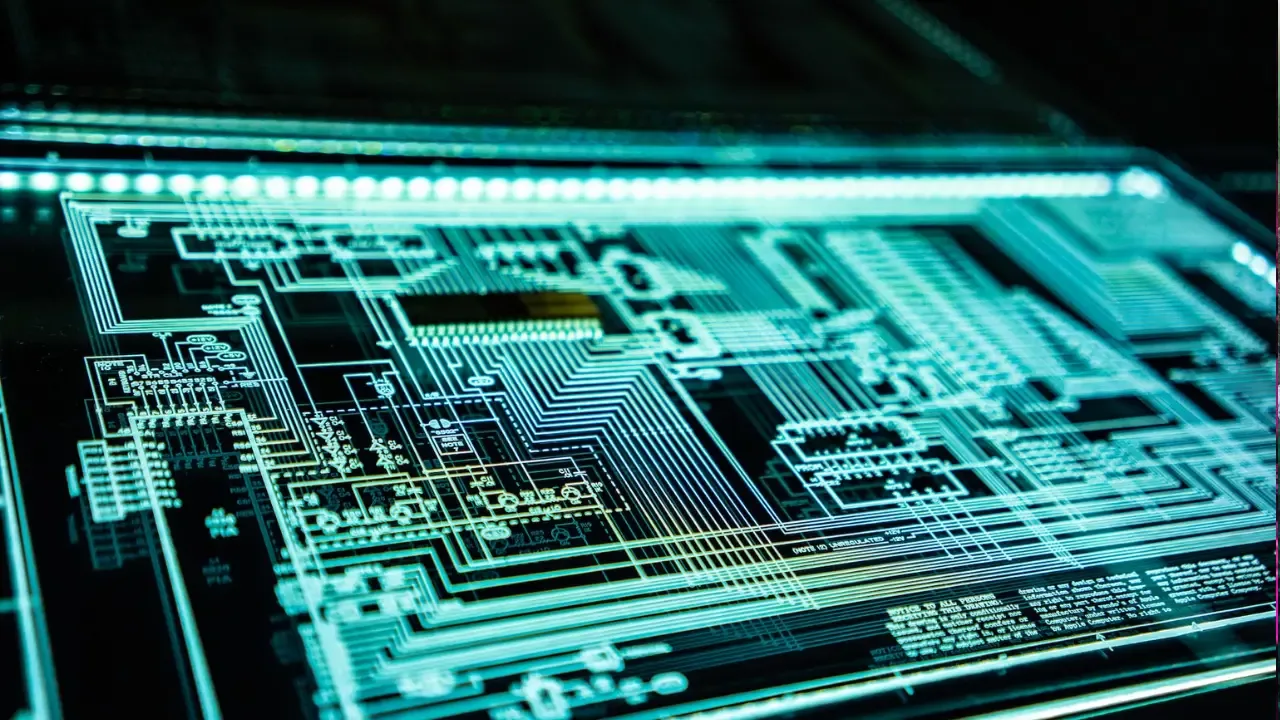
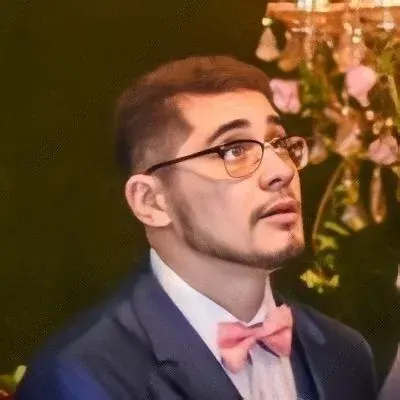
How to Get Selected Element's Outer HTML with jQuery
š Welcome to our tech blog! Today, we're going to tackle a common issue raised by one of our readers: how to get the outer HTML of a selected element using jQuery. We understand that it can be frustrating when the regular .html()
function only returns the inner content, excluding the selected element itself. But fret not, we've got some easy solutions for you!
The Problem: Getting the Outer HTML
In the context shared by our reader, they wanted to obtain the HTML code of a selected object with jQuery. However, the usual .html()
function falls short, as it only retrieves the contents within the selected element, not including the element itself.
The Hackish Methods
They mentioned stumbling upon some 'hackish' methods that involve cloning the object, adding it to a newly created div, and so on. While these methods might work, they can feel overly complicated and dirty. Luckily, there's a better way!
Solution: Using .prop()
and outerHTML
The good news is that starting from jQuery version 1.4.2, there is a prop
function that provides access to an element's properties, including the outerHTML
property. By combining this with the jQuery selector for the selected element, we can easily retrieve the outer HTML.
const selectedElement = $(".selected");
const outerHTML = selectedElement.prop("outerHTML");
console.log(outerHTML);
In the example above, we assume that the selected element has a class of "selected". You can replace this with your own selector based on your specific needs.
Alternative: Using Vanilla JavaScript
For those who prefer a leaner approach without relying on jQuery, you can achieve the same result using plain JavaScript. Here's how:
const selectedElement = document.querySelector(".selected");
const outerHTML = selectedElement.outerHTML;
console.log(outerHTML);
Wrapping Up
Getting the outer HTML of a selected element is now a breeze with jQuery and plain JavaScript. No more hackish solutions or dirty workarounds! š
If you found this guide helpful, be sure to share it with your fellow developers who might be facing the same issue. And don't forget to leave a comment below if you have any questions or suggestions for future blog topics. Happy coding! š»