Get checkbox value in jQuery
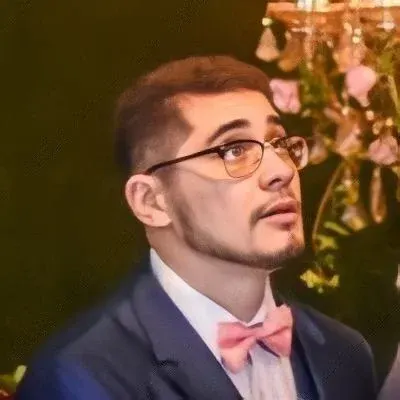
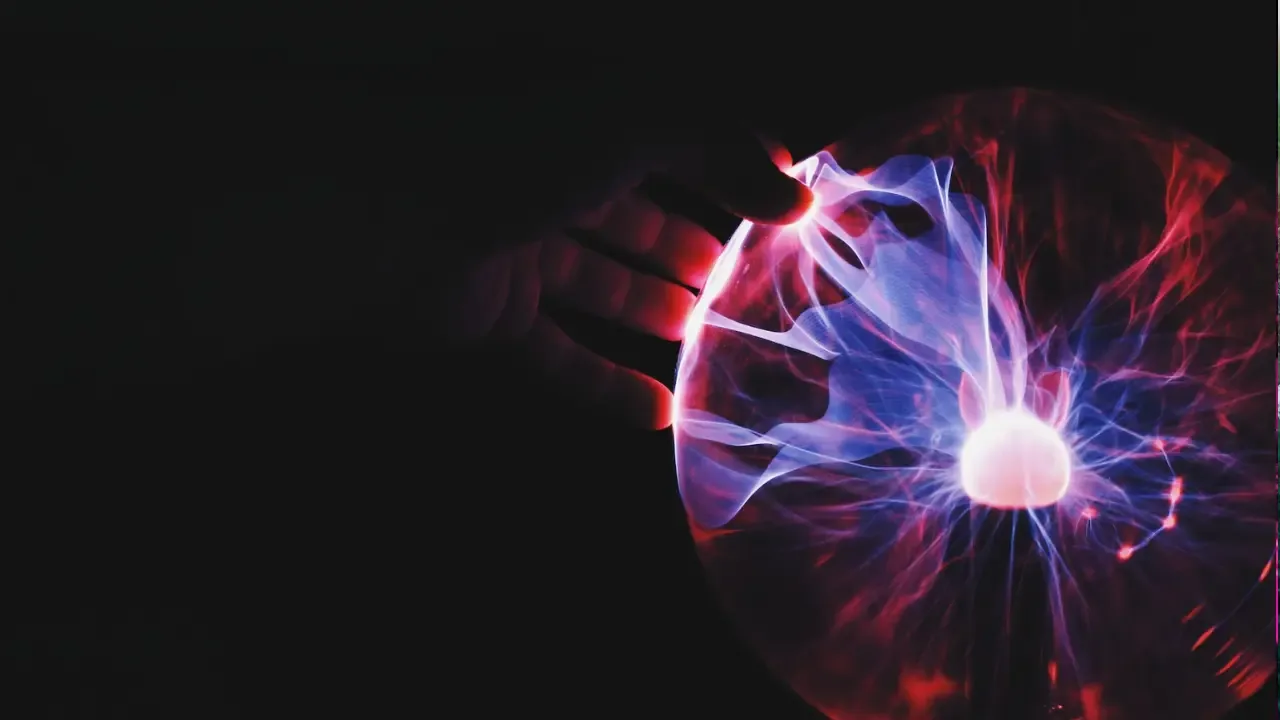
Getting Checkbox Value in jQuery 📝🔍
Are you looking for an easy way to retrieve a checkbox value using jQuery? Look no further! In this blog post, we will discuss a simple and efficient solution to this common problem. 🙌
The Scenario 📝✨
Imagine you have a form with multiple checkboxes, and you need to retrieve the selected checkbox value(s) using jQuery. This might come in handy when you want to perform specific actions based on the user's selection. 📋
<!-- HTML -->
<input type="checkbox" id="checkbox1" value="option1"> Option 1
<input type="checkbox" id="checkbox2" value="option2"> Option 2
The Problem 🤔
The main challenge lies in fetching the value(s) of the selected checkbox(es) using jQuery. Although checkboxes have a value
attribute, you cannot directly retrieve it with jQuery using the val()
function. So, how can we solve this problem? 🤷♂️
The Solution 🌟💡
Here's a simple and effective solution to get the checkbox value in jQuery:
$(document).ready(function() {
$('input[type="checkbox"]').change(function() {
if ($(this).is(':checked')) {
var checkboxValue = $(this).val();
console.log(checkboxValue);
}
});
});
Let's break down the code.
First, we use the
$('input[type="checkbox"]').change()
method to detect changes in the checkbox selection. This event will be triggered whenever a checkbox's state changes.Inside the event handler function, we check if the checkbox is checked using
$(this).is(':checked')
. This ensures that we only get the value of the checked checkbox.Finally, we retrieve the value attribute using
$(this).val()
and store it in thecheckboxValue
variable. You can perform any desired actions here, such as logging the value to the console.
Let's See it in Action! 🎬👀
To see the solution in action, you can copy the provided code into an HTML file and open it in your browser's console. Whenever you check a checkbox, the corresponding value will be logged to the console. 🕵️♂️✅
Take It Further 🚀🔥
Now that you've learned how to get a checkbox's value in jQuery, you can explore additional functionality. For instance, you could:
Use the retrieved value to update other UI elements dynamically.
Store the selected checkbox values in an array for further processing.
Retrieve multiple checkbox values simultaneously by using a class selector.
Get creative! The possibilities are endless! 🌈💡
Conclusion 🎉🔍
Retrieving checkbox values in jQuery doesn't have to be complicated. By using the provided solution, you can easily fetch the selected checkbox value(s) and take dynamic actions based on user input. 🙌
Remember, checkboxes can play an essential role in user interactions, and having the ability to retrieve their values is a valuable skill in web development.
So, what are you waiting for? Start implementing this solution and enhance your website's functionality today! 💪💻
Feel free to share your thoughts and experiences in the comments section below. Happy coding! 🚀👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
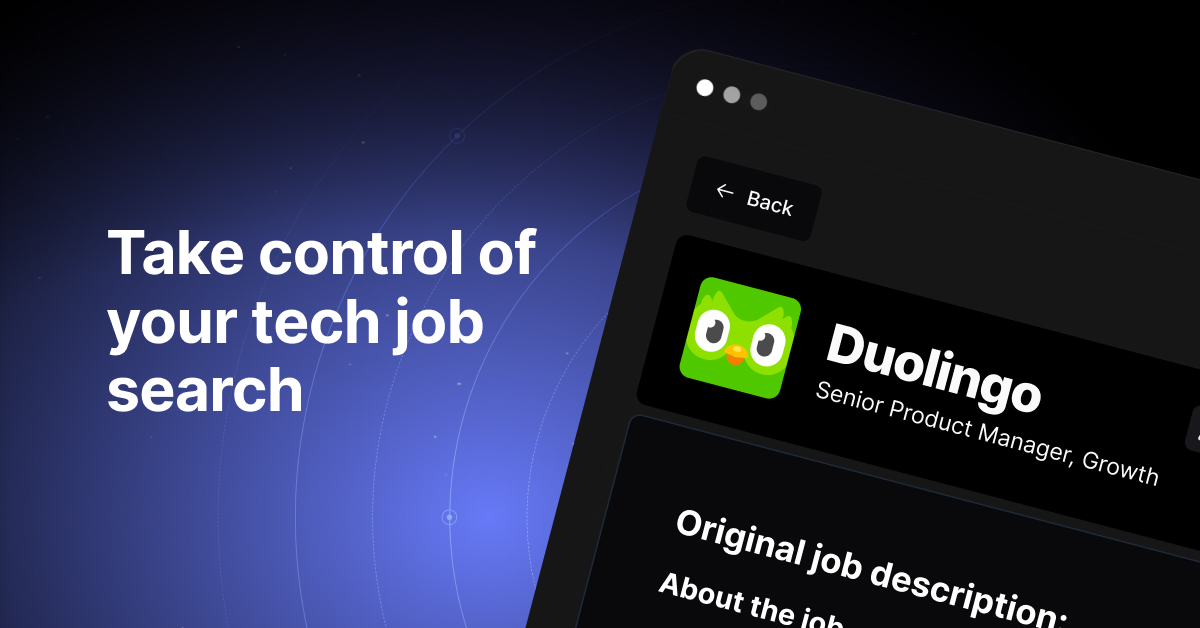