from jquery $.ajax to angular $http
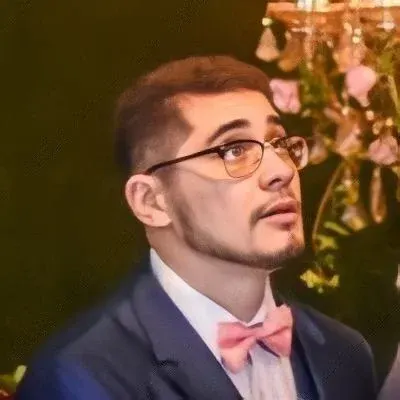
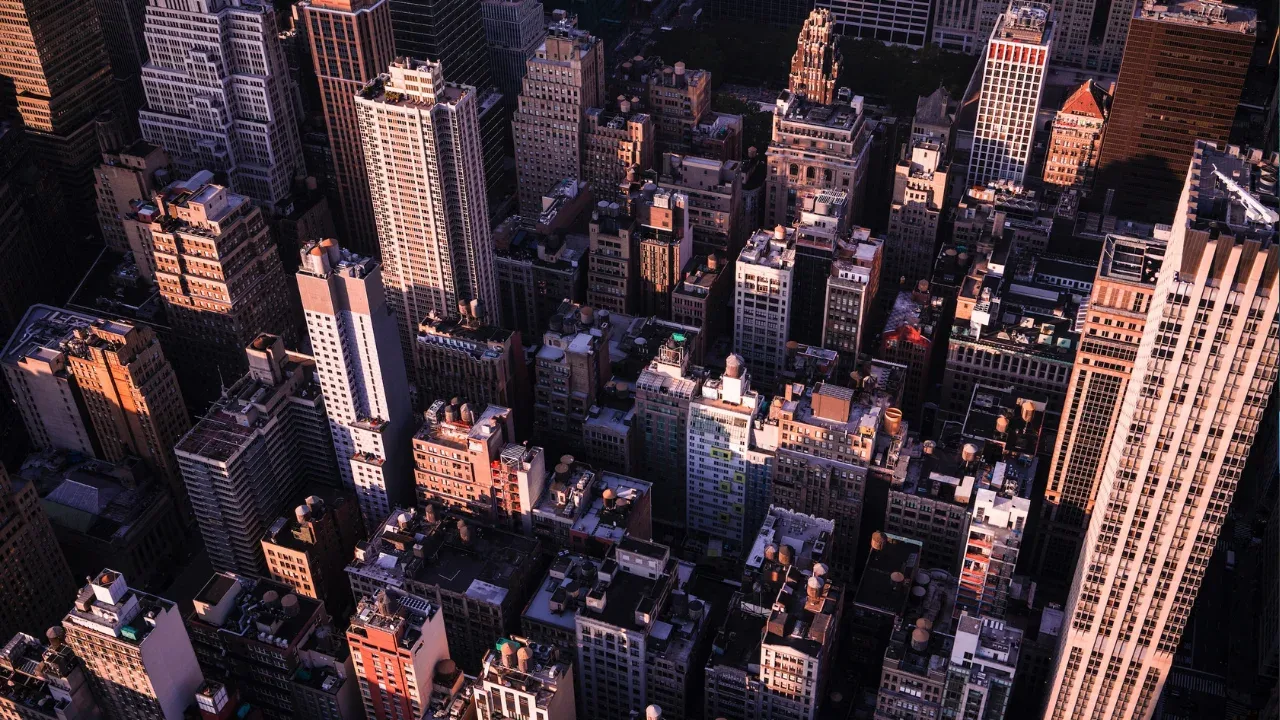
From jQuery $.ajax to Angular $http: Easy Solutions for Common Issues
So you've got some jQuery code that makes an AJAX request and works perfectly fine cross-origin. But now you're trying to convert it to Angular.js code using $http, and you're facing some challenges. Don't worry, we've got you covered! In this blog post, we'll address common issues when transitioning from jQuery $.ajax to Angular $http, provide easy solutions, and offer a compelling call-to-action to keep you engaged.
The Problem
Let's take a look at the code snippet in question:
jQuery.ajax({
url: "http://example.appspot.com/rest/app",
type: "POST",
data: JSON.stringify({"foo":"bar"}),
dataType: "json",
contentType: "application/json; charset=utf-8",
success: function (response) {
console.log("success");
},
error: function (response) {
console.log("failed");
}
});
Based on the code, we can see that a POST request is made to an external API (cross-origin) with some JSON data. The response is then logged in the console based on whether the request was successful or not.
Now, let's move on to the attempted conversion using Angular's $http:
$http({
url: "http://example.appspot.com/rest/app",
dataType: "json",
method: "POST",
data: JSON.stringify({"foo":"bar"}),
headers: {
"Content-Type": "application/json; charset=utf-8"
}
}).success(function(response){
$scope.response = response;
}).error(function(error){
$scope.error = error;
});
The Solutions
Solution 1: Handling the Success and Error Callbacks
In Angular, the success and error callbacks have been replaced with promises. Here's how you can update your code to handle the response correctly:
$http({
url: "http://example.appspot.com/rest/app",
method: "POST",
data: JSON.stringify({"foo":"bar"}),
headers: {
"Content-Type": "application/json; charset=utf-8"
}
}).then(function(response){
$scope.response = response.data;
}, function(error){
$scope.error = error;
});
In this updated code, we're using the .then()
function instead of .success()
and .error()
. The response
object now includes the actual data in response.data
, which is why we're assigning it to $scope.response
.
Solution 2: Configuring Cross-Origin Requests
By default, Angular's $http service enforces the browser's same-origin policy. To enable cross-origin requests, you'll need to configure CORS (Cross-Origin Resource Sharing) on the server-side. Check the documentation of the server or API you're interacting with for instructions on how to enable this.
Solution 3: Angular's Dependency Injection
Make sure that you have injected the $http
service into your controller or wherever you're using it. If you haven't done so, the code snippet won't work as expected.
Try it Out & Engage!
Now that you have the solutions to the common issues when transitioning from jQuery's $.ajax to Angular's $http, it's time to put them into action! Give the updated code a try and see if it resolves the problem you were facing.
If you have any further questions or want to share your experience, feel free to leave a comment below. We'd love to hear from you and help you out!
Keep exploring, keep coding ✨🔥💻
Join the conversation!
Leave a comment below and let us know:
Have you encountered any issues when transitioning from jQuery's $.ajax to Angular's $http?
Did the provided solutions help you resolve those issues?
Share your experience or any tips you have for others facing similar challenges.
Remember, learning is a journey, and we're here to support you every step of the way!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
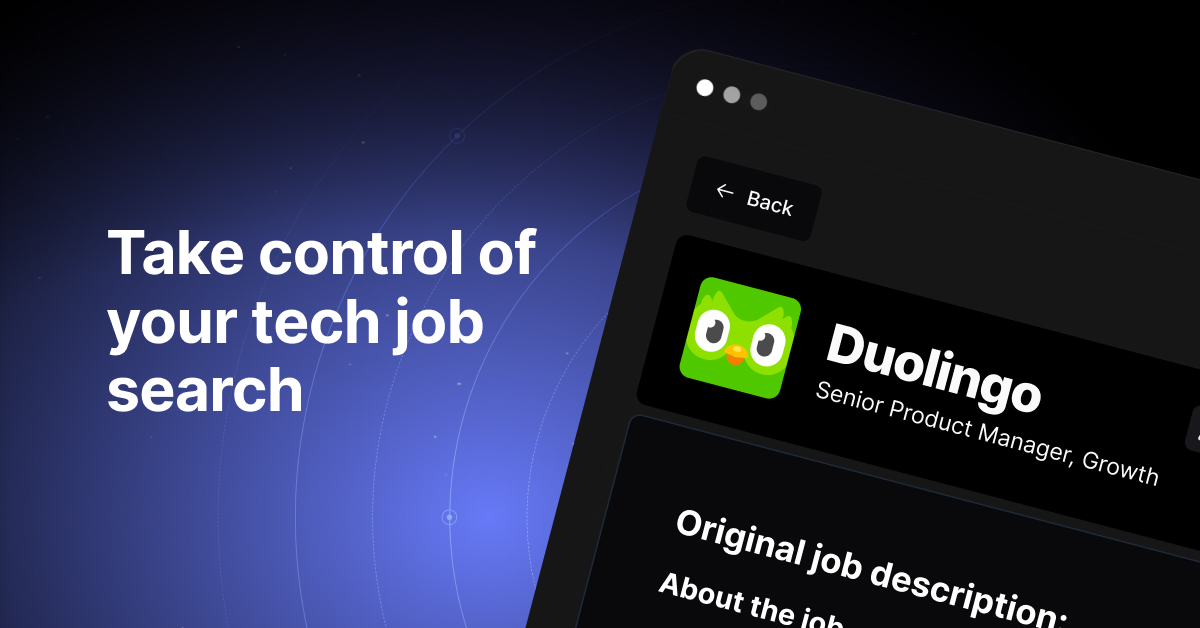