Find out whether radio button is checked with JQuery?
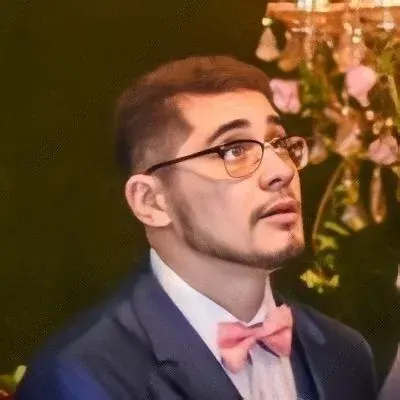
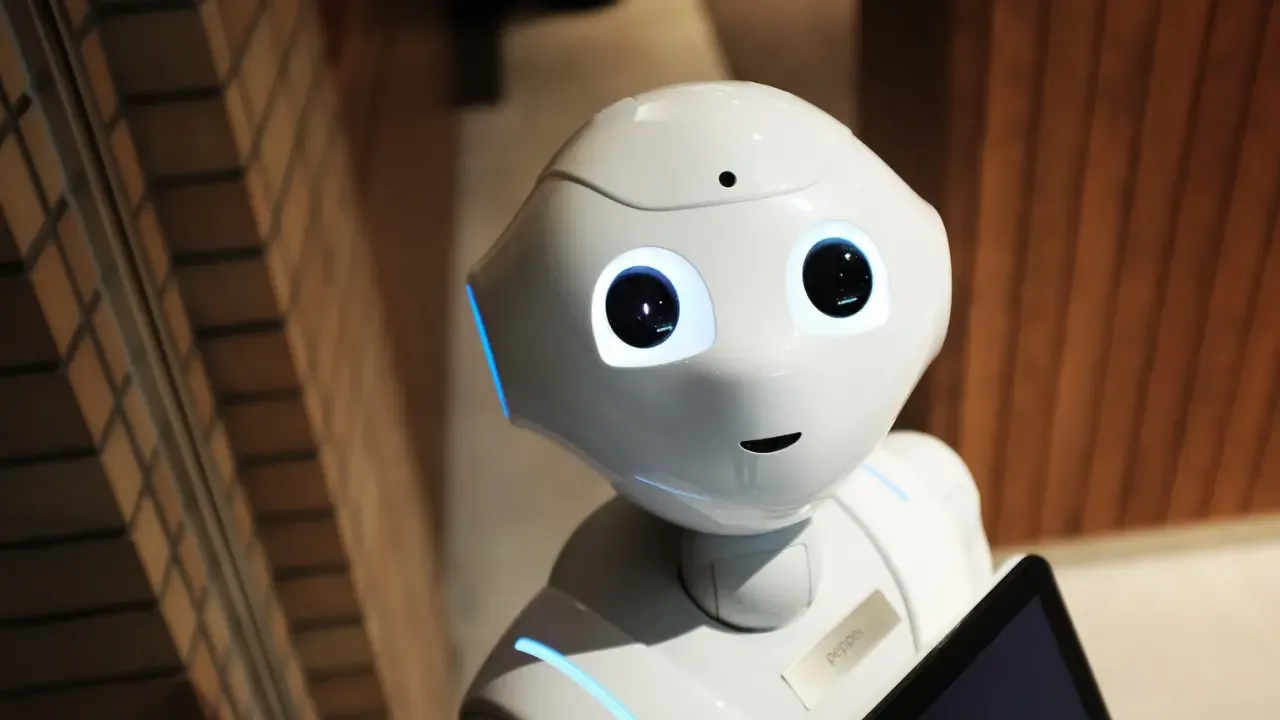
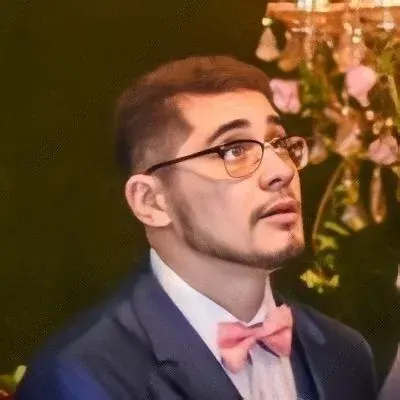
Understanding Radio Buttons and JQuery
Radio buttons are a popular and widely-used form element in web development. They allow users to select one option from a group of choices. In this blog post, we will address a common question: how do we find out if a radio button is checked using JQuery?
The specific problem presented is that the code provided sets a radio button to checked successfully but lacks the functionality to trigger an alert when the radio button is checked without the use of the click function. Let's dive into the solutions!
Solution 1: Using Change Event
One way to achieve the desired functionality is by utilising the change
event in JQuery. By attaching an event listener to the radio button input, we can execute code whenever its checked state changes.
$('#radio_button').change(function() {
if ($(this).is(':checked')) {
// Your code here
alert("Radio button is checked!");
}
});
The change
event will be triggered whenever the radio button's state changes, either by clicking on it or through other means (e.g., selecting an option using the keyboard).
Solution 2: Monitor Whole Group
If you have a group of related radio buttons, and you want to check if any of them are selected, you can accomplish this by monitoring the entire group. You can use a common class for all the radio buttons within the group, and then attach the change
event handler to the class instead of the individual radio buttons.
$('.radio_group').change(function() {
if ($(this).is(':checked')) {
// Your code here
alert("One of the radio buttons in the group is checked!");
}
});
In this example, replace .radio_group
with the appropriate class name used for your radio button group.
Take it a Step Further
Now that you know how to find out if a radio button is checked with JQuery, you can enhance this functionality to suit your specific needs. Some additional ideas could be:
Instead of an alert, you could display a message on the web page using
console.log
or by updating a specific element's text.You could combine the change event with conditional statements to perform different actions based on the selected radio button.
Time to Get Creative!
With these solutions in your toolbelt, you can now easily detect when a radio button is checked without relying solely on the click function. Perfect for creating exciting and interactive user experiences on your website!
Feel free to experiment with these solutions, and don't forget to share your thoughts and any cool implementations you come up with in the comments below. Happy coding! 👩💻🔥
📚 Learn more about JQuery events: JQuery Events Documentation
🌟 Did you find this blog post helpful? Let us know by tapping the 👍 button and sharing it with your friends!