Check if inputs are empty using jQuery
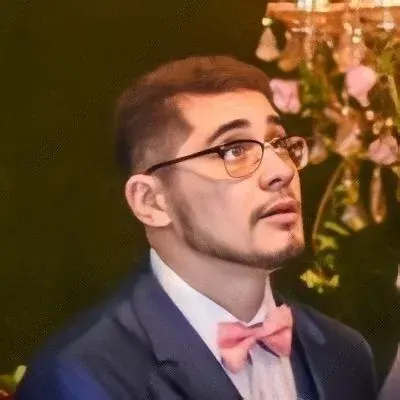
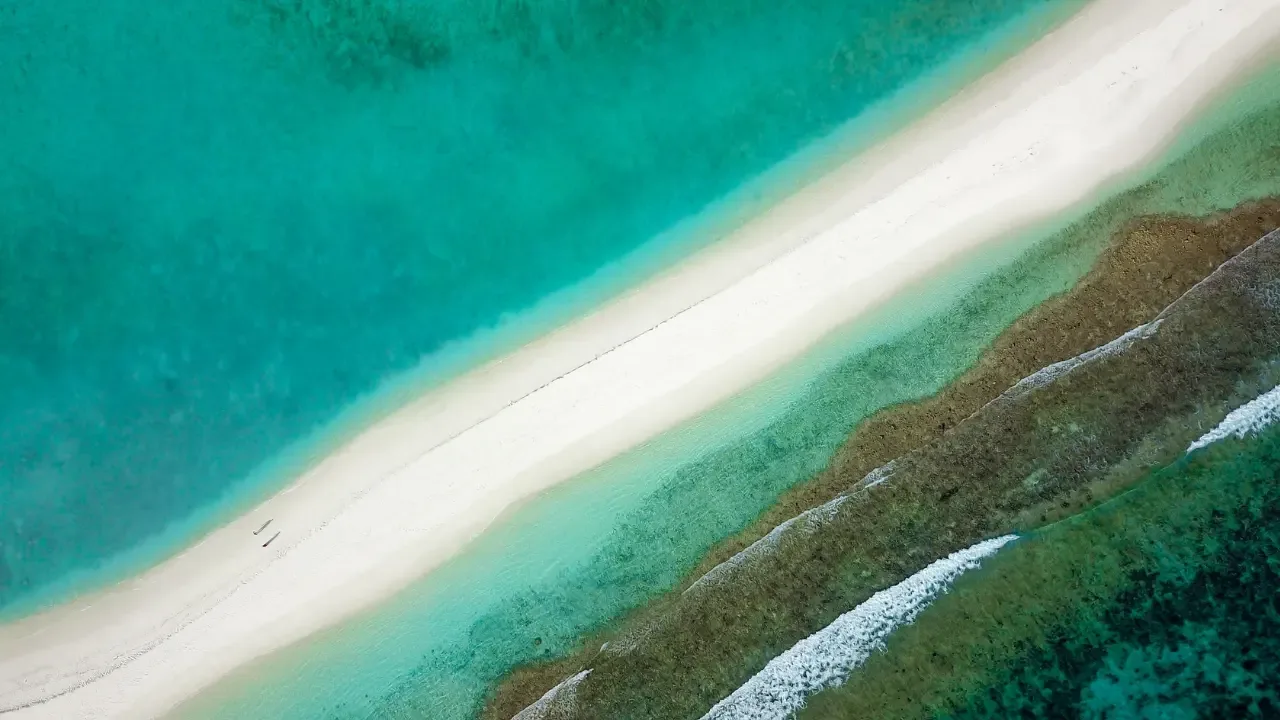
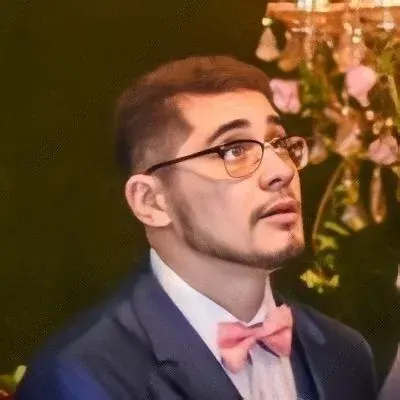
📝 Blog Post Title: "Easy jQuery Trick to Check if Inputs are Empty"
Introduction: Hey there tech enthusiasts! 😄 In today's blog post, we're going to delve into a highly requested topic that developers often struggle with: checking if inputs are empty using jQuery. We'll explore a common issue and provide you with an easy-to-implement solution. So, if you're ready, let's dive in and solve this problem together! 💪
Understanding the Problem:
Our reader, let's call them Jane, was working on a web form that required all fields to be filled in. Jane specifically wanted to display a red background if a field was clicked into but not filled out. However, the code Jane shared isn't producing the desired result. Let's understand what's going wrong in her code.
Analyzing the Code:
$('#apply-form input').blur(function () {
if ($('input:text').is(":empty")) {
$(this).parents('p').addClass('warning');
}
});
In Jane's code, she is attaching a blur event handler to all input elements inside the element with the ID "apply-form". The blur event occurs when the input loses focus, meaning when the user clicks outside the input box.
Inside the event handler function, Jane is trying to check if the input field is empty using $('input:text').is(":empty")
. However, this condition always evaluates to true
, which results in adding the "warning" class to the parent paragraph ($(this).parents('p')
) regardless of whether the field is filled or not.
The Solution:
To solve the issue and check if the input fields are empty, we can modify Jane's code slightly. Instead of using :empty
, we need to utilize the .val()
method to get the input's value and check its length. Here's the updated code:
$('#apply-form input').blur(function () {
if ($(this).val().length === 0) {
$(this).parents('p').addClass('warning');
}
});
By using $(this).val().length
inside the condition, we can accurately determine if the input field is empty. If the length of the input value is zero, we add the "warning" class to the parent paragraph.
Let's Test It Out:
To ensure our solution works as expected, let's apply it to Jane's code. Now, when an input field is clicked into and left empty, the corresponding parent paragraph will have the "warning" class added, resulting in a red background.
Go ahead and give it a try! Experiment with different scenarios and see the magic happen. Make sure your inputs magically turn red when empty!
🌟 Call-to-Action: Share Your Experience!
We hope this quick and easy solution helped you solve the problem of checking if inputs are empty using jQuery. If you have any additional questions, faced any issues, or want to share your success story, please let us know in the comments below. We love hearing from our fellow tech enthusiasts!
Don't forget to share this blog post with your developer friends who are struggling with this problem. Together, we can make their coding lives easier! 💻🚀
Happy coding! 😊✨