Best way to remove an event handler in jQuery?
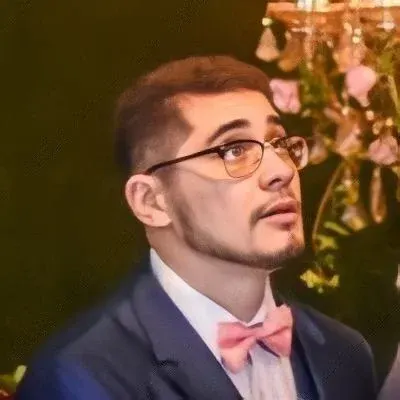
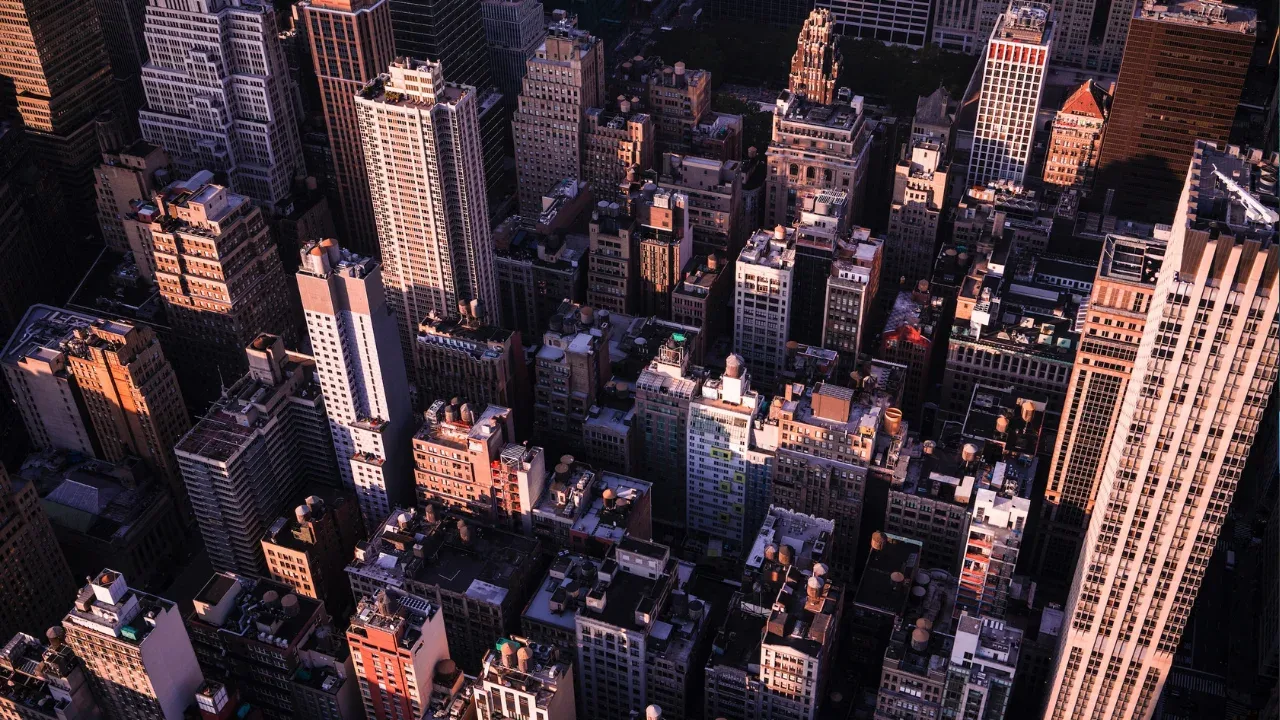
Best Way to Remove an Event Handler in jQuery 🌟
So, you've encountered a pesky problem with event handlers in jQuery. You have an input type="image"
element that triggers a popup when clicked, but you need to disable this event handler when the user enters a zero into an associated text box. But fear not, fellow coder! In this guide, I'll show you the best way to remove an event handler in jQuery.
Understanding the Issue 🤔
Let's break down the problem you're facing. You have an input type="image"
element that triggers a function when clicked. However, when the user enters a zero into the associated text box, you want to disable this function. The code you've tried, $('#myimage').click(function { return false; });
, doesn't seem to work. So, what's going wrong here?
The Issue with Your Code 💡
The problem lies in how you're attempting to remove the event handler. The code you've tried essentially binds a new empty function to the click
event of $('#myimage')
, which doesn't disable the existing event handler. It merely adds a new, ineffective one.
The Solution: Using $.off() Method 🔧
To truly remove the event handler, we need to access the jQuery off()
method. This method allows us to unbind specific event handlers or even remove all event handlers bound to an element. Let's see how to implement this.
$('#myimage').off('click');
By using off()
, we specify the event we want to unbind, which is 'click'
in this case. This single line of code will remove the event handler associated with the click
event from $('#myimage')
.
Easy Peasy! Let's Recap 😄
The code you tried,
$('#myimage').click(function { return false; });
, doesn't remove the event handler; it merely adds a new, ineffective one.To truly remove an event handler in jQuery, we can use the
off()
method.The
off()
method requires us to specify the event we want to unbind, which in this case is'click'
.By using
$('#myimage').off('click');
, we successfully remove the event handler associated with theclick
event from$('#myimage')
.
Time to Spice Up Your Code 🚀
Now that you know the best way to remove an event handler in jQuery, go ahead and implement it in your code. Say goodbye to ineffective event handlers and hello to more efficient and smoother user interactions!
If you found this guide helpful, don't forget to share it with your fellow coders and give it a thumbs up! And feel free to leave any comments or questions below. Happy coding! 😊✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
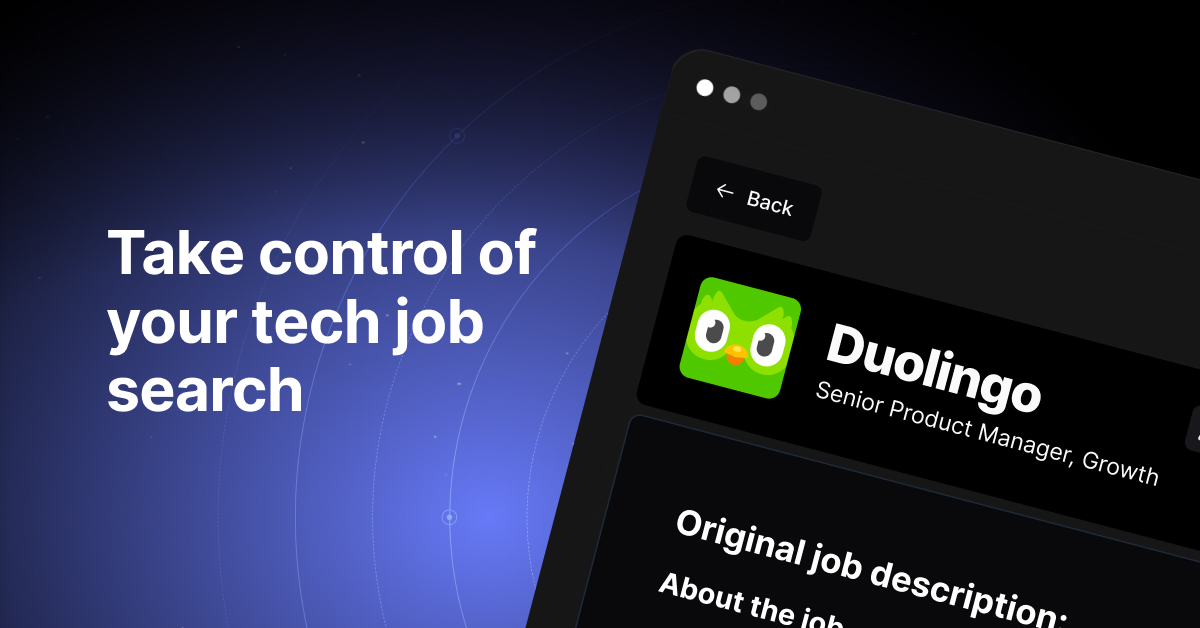