XML parsing of a variable string in JavaScript
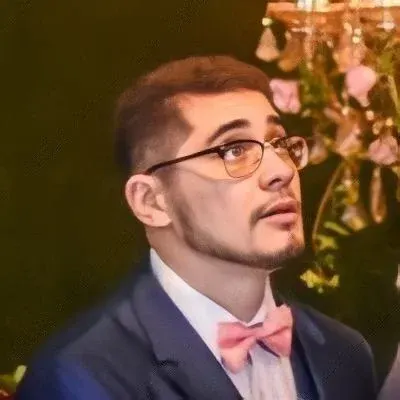
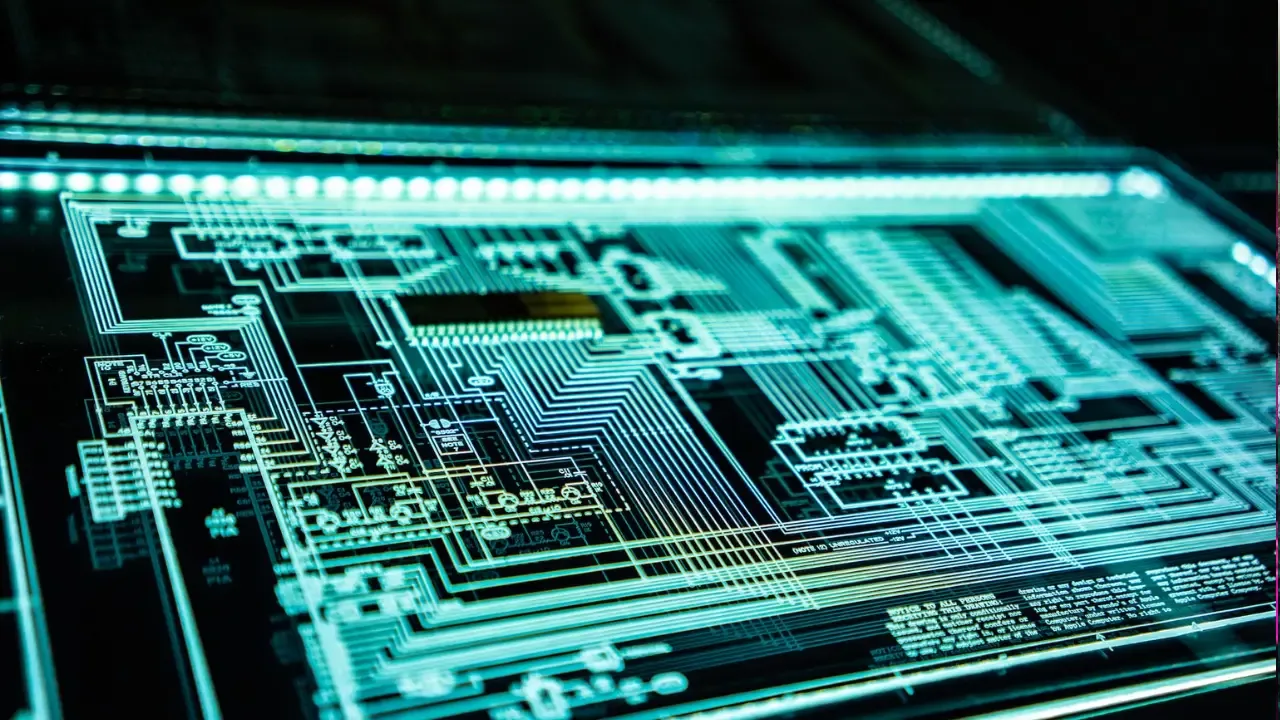
🕵️♀️ Demystifying XML Parsing in JavaScript
Are you faced with the challenge of parsing a variable string containing well-formed and valid XML in JavaScript? Don't fret! In this blog post, we'll explore common issues associated with XML parsing and provide easy solutions to help you overcome this hurdle. So, grab your coding hat and let's dive in! 💻🤓
🧩 Understanding the Problem
So, you have a variable string that contains XML, and you want to extract data or perform further processing using JavaScript. The key here is to parse the XML, converting it into a format that JavaScript can easily understand and manipulate.
🔍 Exploring Solutions
Thankfully, JavaScript provides several built-in methods that make XML parsing a breeze. Let's explore three popular options:
1. Using the DOMParser API
The DOMParser API allows us to parse an XML string into a Document object, which we can then traverse and manipulate using JavaScript.
const xmlString = "<example><foo>bar</foo></example>";
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(xmlString, "text/xml");
// Now, you can access and manipulate the XML document
const fooValue = xmlDoc.getElementsByTagName("foo")[0].textContent;
console.log(fooValue); // Output: "bar"
2. Employing the XML Parser Library
Sometimes, the XML parsing requirements might go beyond what the DOMParser API can handle. In such cases, leveraging popular XML parser libraries, like xml2js or fast-xml-parser, can simplify your life by providing more advanced parsing capabilities.
These libraries allow you to transform XML into an easily manipulatable JavaScript object or JSON structure, making it a breeze to work with. Remember, if you choose this approach, you'll need to install the library using a package manager like npm or yarn.
const xml2js = require('xml2js');
const xmlString = "<example><foo>bar</foo></example>";
xml2js.parseString(xmlString, (error, result) => {
if (error) {
console.error(error);
return;
}
const fooValue = result.example.foo[0];
console.log(fooValue); // Output: "bar"
});
3. Leaning on Browser-Specific XML Parsers
Sometimes, you might come across browser-specific XML parsing requirements. For instance, Internet Explorer versions older than IE9 require the use of ActiveX objects. Although it's rare to encounter such scenarios today, if you're working on a legacy project, it's worthwhile to consider these special cases and seek appropriate solutions.
📣 Taking Action
Now that you're armed with solutions to parse XML in JavaScript, it's time to put your newfound knowledge into practice. Select the most suitable approach based on your project requirements. You can also experiment with other XML parsing libraries or browser-specific techniques.
Share your XML parsing experiences or any additional tips and tricks in the comments below. Let's learn from each other! 👇🤩
Happy XML parsing! Happy coding! 🚀💻
Disclaimer: The examples provided in this blog post assume a browser environment or a compatible JavaScript runtime. Please ensure your target environment supports the specified methods or libraries.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
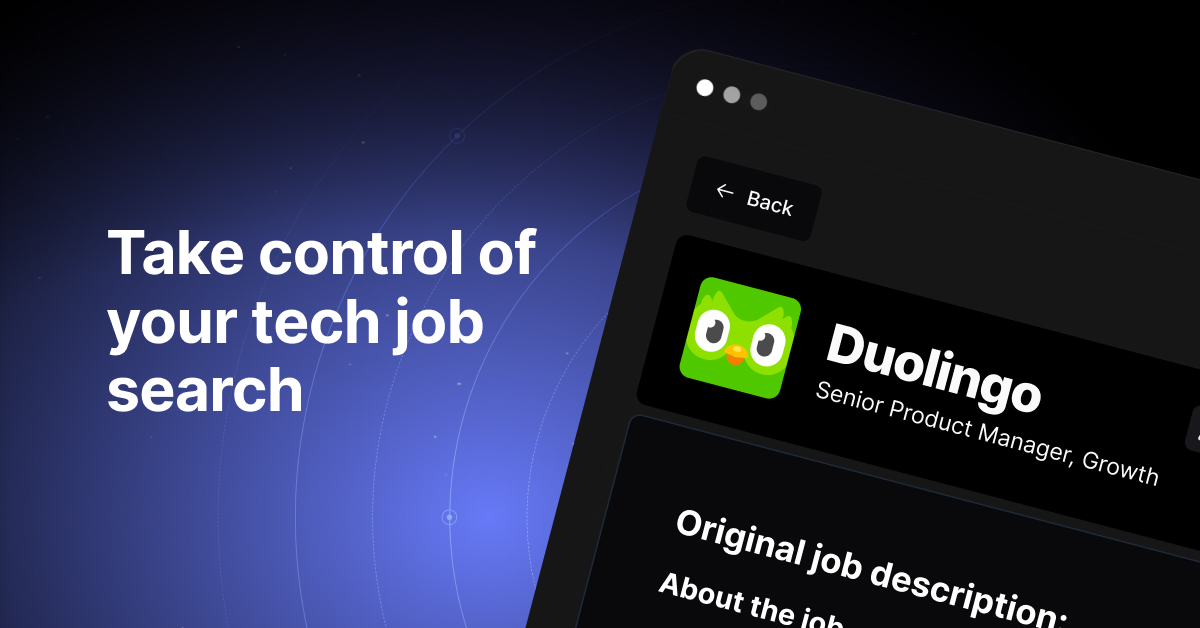