Writing to files in Node.js
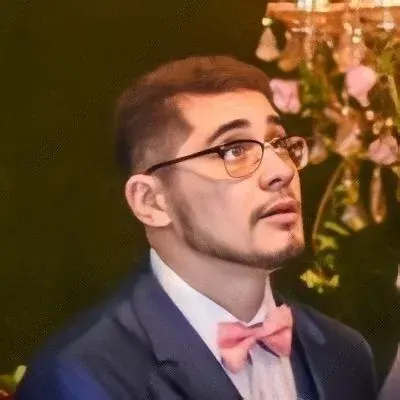
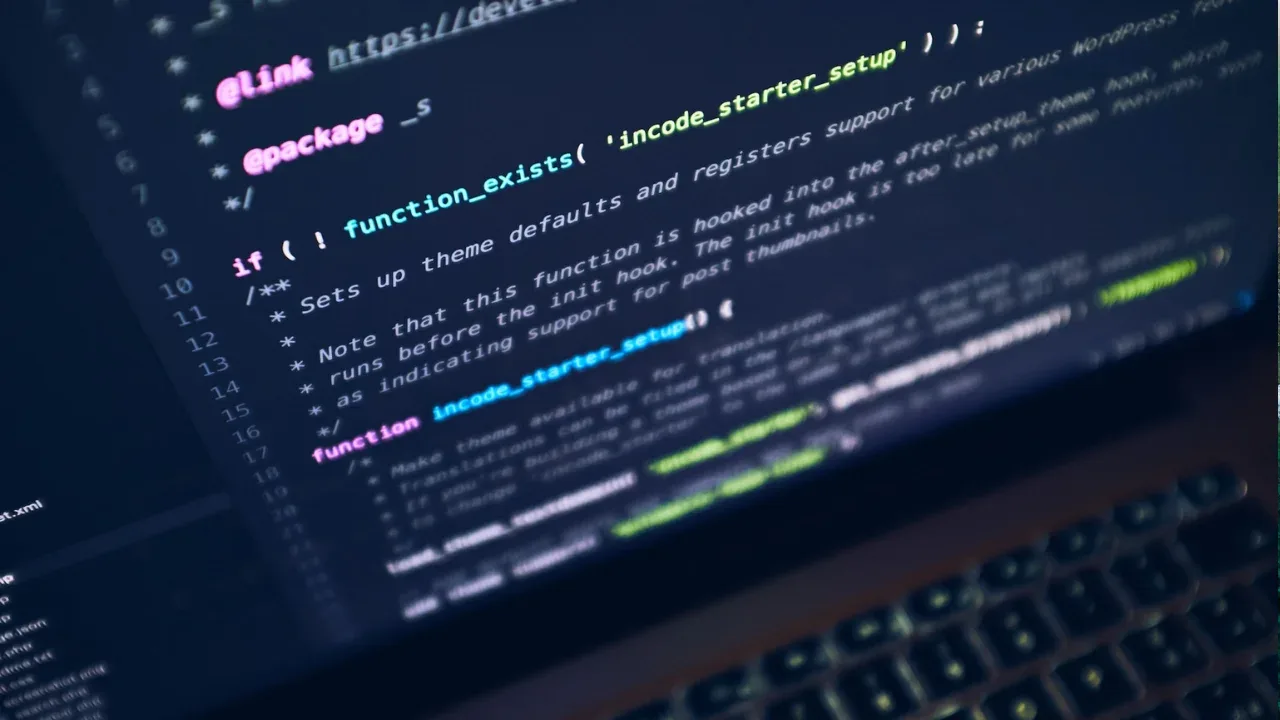
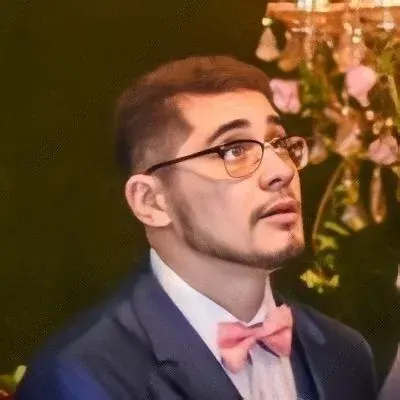
Writing to Files in Node.js: A Simple Guide
So you've been exploring the world of Node.js and you've landed on a roadblock: writing to a file. Don't worry, you're not alone! Many developers face this challenge when working with Node.js. In this blog post, we'll demystify writing to files in Node.js, address common issues, and provide you with easy solutions. Let's dive right in! 🚀
Understanding the Basics
Before we jump into the solutions, let's quickly cover the basics of writing to files in Node.js.
In Node.js, you can use the built-in fs
module to interact with the file system. This module provides various methods for reading, writing, and manipulating files. When it comes to writing to a file, the fs.writeFile()
method is your best friend. This method takes in the file path, the data you want to write, and an optional callback function.
Now that we have a little background, let's explore a common problem and its easy solution.
The Problem: Writing to a File
Imagine you have a simple text file named myFile.txt
and you want to write the string "Hello, World!" to this file using Node.js. How can you accomplish this?
The Solution: Using fs.writeFile()
Here's a step-by-step solution using the fs.writeFile()
method:
Import the
fs
module:const fs = require('fs');
Specify the file path:
const filePath = 'path/to/myFile.txt';
Use
fs.writeFile()
to write data to the file:fs.writeFile(filePath, 'Hello, World!', (err) => { if (err) throw err; console.log('Data written to file successfully!'); });
That's it! You have successfully written "Hello, World!" to the myFile.txt
file.
Handling Common Issues
Error Handling
When writing to a file, it's essential to handle errors. The callback function passed to fs.writeFile()
includes an error parameter (err
). Check it to ensure there were no errors during the write operation. If an error occurs, you can handle it gracefully, maybe by logging an error message or taking appropriate action.
Appending Data
If you want to append data to an existing file rather than overwrite it, you can use the fs.appendFile()
method instead. The usage is similar to fs.writeFile()
, but this time, the data will be appended to the end of the file.
Encoding
By default, fs.writeFile()
writes data to a file using the 'utf8'
encoding. If you're working with a different encoding, you can specify it as the third argument in the method. For example, to write data using the 'latin1'
encoding, you would modify the code as follows:
fs.writeFile(filePath, 'Hello, World!', 'latin1', (err) => {
if (err) throw err;
console.log('Data written to file successfully!');
});
Get Creative!
Now that you know how to write to files in Node.js, let your imagination run wild! You can automate file generation, save user data, or even build a full-fledged file-processing application. The possibilities are endless. Share your creations in the comments below! 💡
Conclusion
In this blog post, we explained how to write to files in Node.js using the fs.writeFile()
method. We covered the basics, provided a step-by-step solution, addressed common issues, and gave you the tools to get creative. Start writing code and let the files be your canvas! 🔥
Did this guide help you solve your file-writing problem? Share your success stories or lingering questions in the comments section. Happy coding! 💻📝
Sources: