Write / add data in JSON file using Node.js
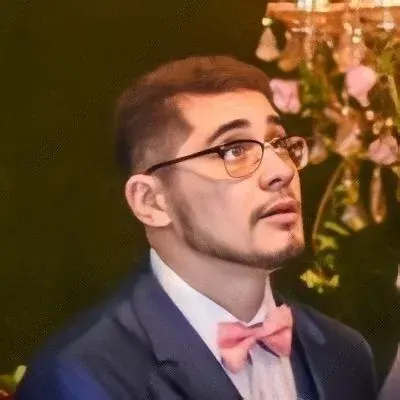
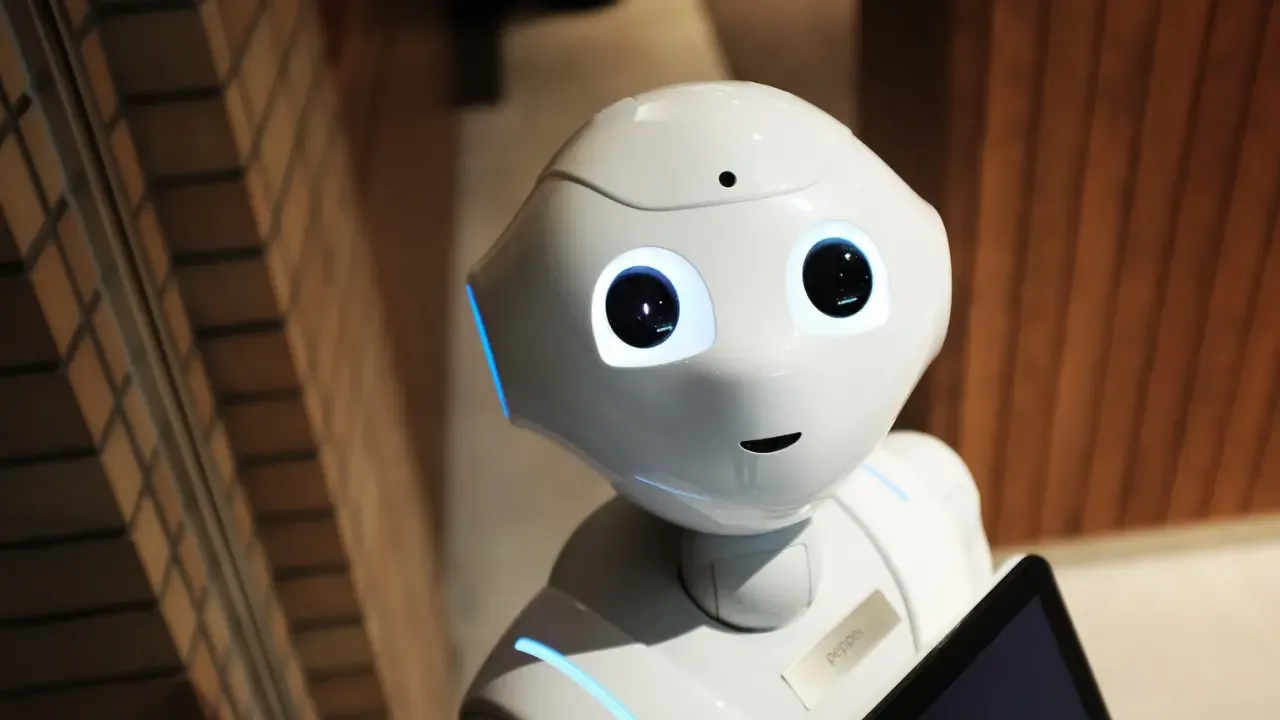
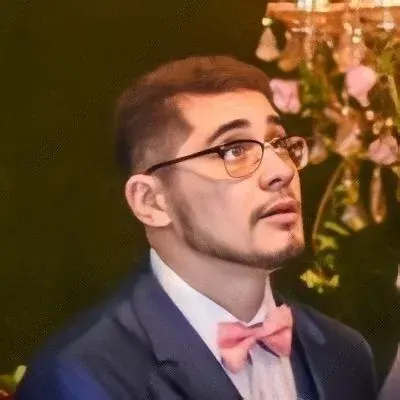
How to Write and Add Data in a JSON File using Node.js
Are you struggling to write and add data to a JSON file using Node.js? Look no further! In this guide, we will walk you through a step-by-step process to help you achieve your goal. 📝💻
The Problem
Let's start by addressing the problem at hand. The original code provided in the question uses the jsonfile
module to write data to a JSON file. However, each time the code runs, it overwrites the existing data in the file instead of adding new elements. The desired output is a JSON file with an array of objects, where each object represents the ID and square of a number.
The Solution
To solve this problem, we will use the built-in fs
module in Node.js. This module provides file system-related functionality, allowing us to read, write, and modify files.
Here's an updated version of the code that will give you the desired output:
const fs = require('fs');
// Check if the file exists
fs.exists('myjsonfile.json', function(exists) {
if (exists) {
console.log("File exists");
fs.readFile('myjsonfile.json', function readFileCallback(err, data) {
if (err) {
console.log(err);
} else {
let obj = JSON.parse(data);
for (let i = 0; i < 5; i++) {
obj.table.push({
id: i,
square: i * i
});
}
let json = JSON.stringify(obj);
fs.writeFile('myjsonfile.json', json);
}
});
} else {
console.log("File does not exist");
let obj = {
table: []
};
for (let i = 0; i < 5; i++) {
obj.table.push({
id: i,
square: i * i
});
}
let json = JSON.stringify(obj);
fs.writeFile('myjsonfile.json', json);
}
});
In this updated code, we first check if the file myjsonfile.json
exists. If it does, we read its content and parse it into a JavaScript object. We then loop through the data and add new elements to the table
array. Finally, we convert the updated object back into a JSON string and write it back to the file.
If the file does not exist, we create a new object and follow the same steps as above to add the elements. This ensures that the code can be re-run multiple times without losing the existing data.
Conclusion
Congratulations! 🎉 You now know how to write and add data to a JSON file using Node.js. By using the fs
module and following the example code provided, you can easily achieve your desired output.
Feel free to experiment with the code and customize it to fit your specific needs. If you have any questions or need further assistance, drop a comment below and we'll be happy to help!
Now it's your turn to try it out. Let's write some data to JSON files! 💪😊