Why is using "for...in" for array iteration a bad idea?
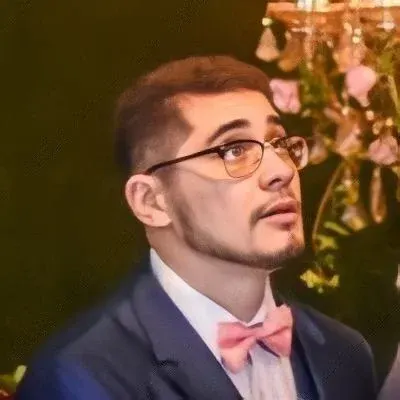
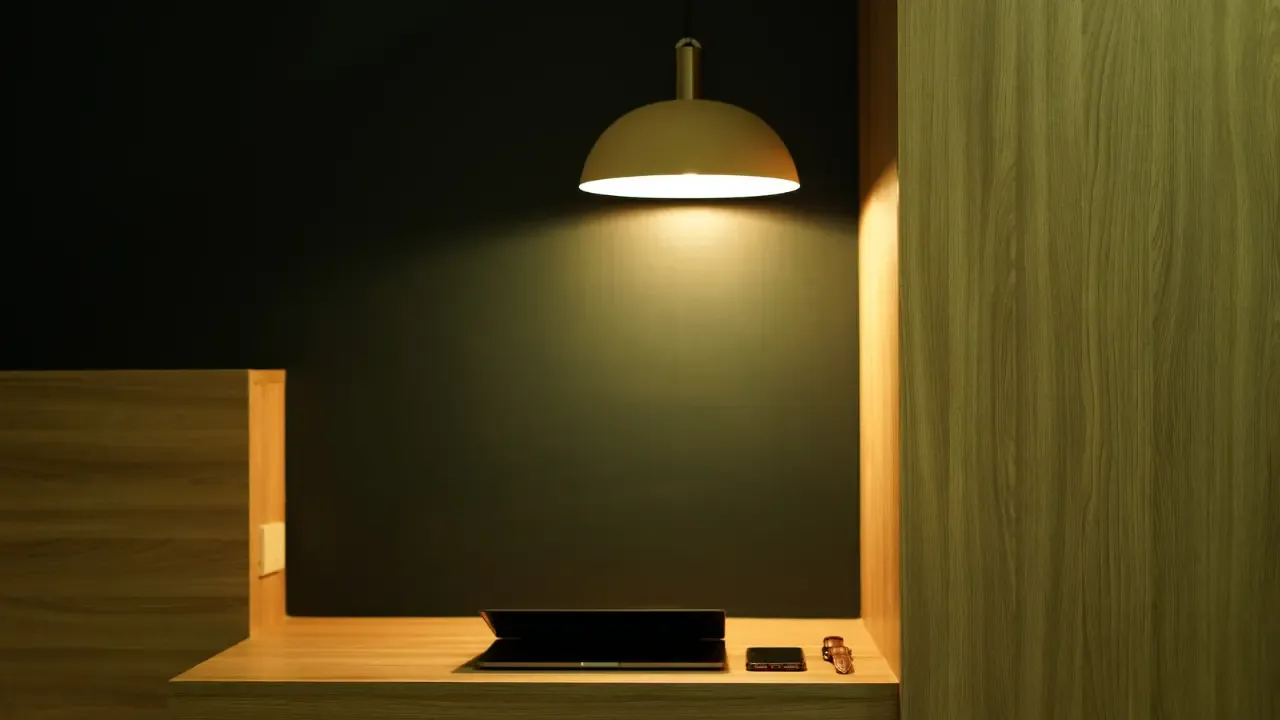
Why is using "for...in" for array iteration a bad idea? ๐ซ๐
So, you've been told not to use for...in
with arrays in JavaScript, huh? Good on you for seeking answers! ๐ค It's important to understand why certain practices are discouraged in order to write clean and efficient code. Let's dive in and find out why using for...in
for array iteration can be a bad idea. ๐ฅ
The Problem ๐ฉ
When it comes to iterating through arrays, JavaScript offers several methods like forEach()
, map()
, and for...of
. However, using for...in
for array iteration can lead to unexpected and problematic behavior. ๐ฑ
1. Including Non-Numerical Properties ๐ซ๐ข
The for...in
loop is designed to loop over the enumerable properties of an object. But, arrays in JavaScript are not just objects, they also come with some built-in properties and methods, such as length
, forEach()
, and map()
. When using for...in
on an array, these properties are also included in the iteration, which is usually not what we want. ๐
const myArray = ["apple", "banana", "cherry"];
for (let key in myArray) {
console.log(key);
}
The output of this code will be:
0
1
2
forEach
map
length
2. Order of Iteration ๐โ ๏ธ
When using for...in
, there is no guarantee for the order in which the properties will be iterated. This can lead to unexpected results when you rely on a specific order for your array elements. Arrays are meant to be ordered collections, and using for...in
might break this principle. ๐ฉ
const myArray = ["apple", "banana", "cherry"];
Array.prototype.customMethod = function() {
console.log("Custom method");
};
for (let key in myArray) {
console.log(key);
}
The output of this code might be:
0
1
2
customMethod
3. Prototype Dilemma โ ๏ธ๐ฌ
As shown in the previous example, properties added to the Array.prototype
can also be included in the iteration. While we might not have intentionally added any properties to the prototype, some libraries or polyfills might do so. Consequently, unexpected properties could be iterated over, resulting in potential bugs and hard-to-find issues. ๐จ
The Solution โ
Now that we understand the problems with using for...in
for array iteration, let's explore some better alternatives. ๐
1. Use "for...of" ๐โจ
The for...of
loop is specifically designed for iterating through iterable objects, including arrays. It provides a clean syntax and ensures that only the array elements are considered, without any added properties or methods.
const myArray = ["apple", "banana", "cherry"];
for (let item of myArray) {
console.log(item);
}
The output of this code will be:
apple
banana
cherry
2. Use Array Methods ๐งช๐ฌ
JavaScript arrays come with powerful built-in methods like forEach()
, map()
, filter()
, and many others. These methods provide a functional and concise way to iterate through arrays, perform operations, and avoid the pitfalls of for...in
.
const myArray = ["apple", "banana", "cherry"];
myArray.forEach((item) => {
console.log(item);
});
The output of this code will be the same as the previous example:
apple
banana
cherry
Conclusion ๐๐๏ธ
Using for...in
for array iteration can lead to unexpected results and potential bugs. It includes non-numerical properties, has an unpredictable order of iteration, and can be affected by prototype modifications. Instead, opt for cleaner alternatives like for...of
or the various array methods available.
Next time you find yourself needing to iterate through an array, remember to choose the right tool for the job. Your code will be cleaner, more reliable, and easier to debug. ๐
Have you ever encountered any issues using for...in
with arrays? Share your experience below! Let's make our JavaScript code better together! ๐ช๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
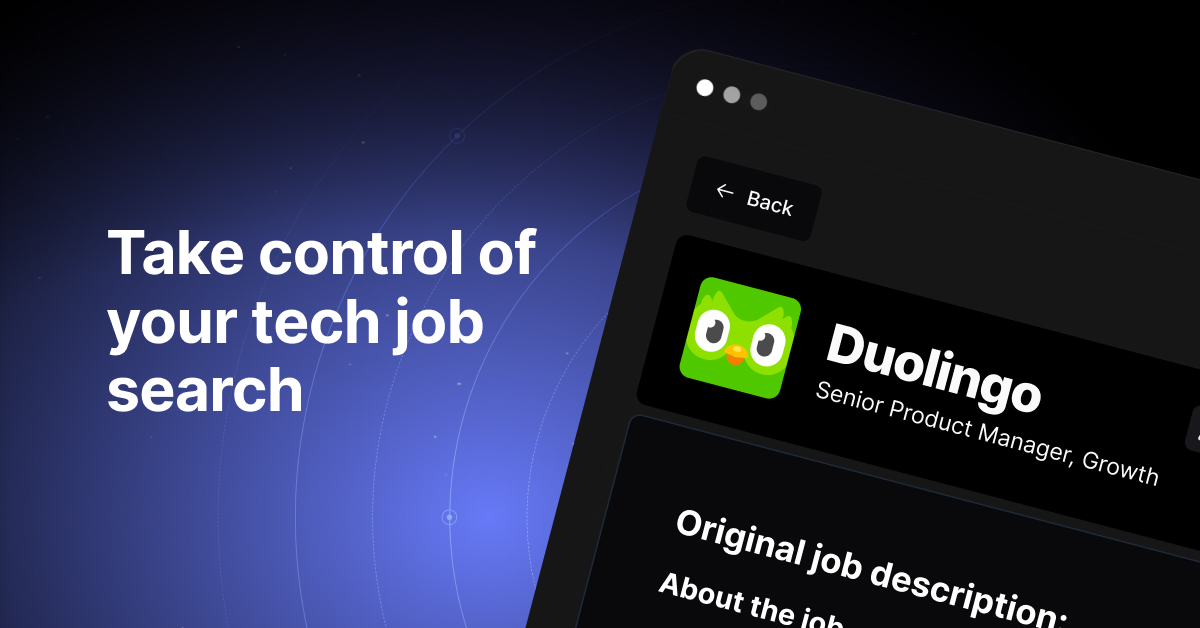