Why does Node.js" fs.readFile() return a buffer instead of string?
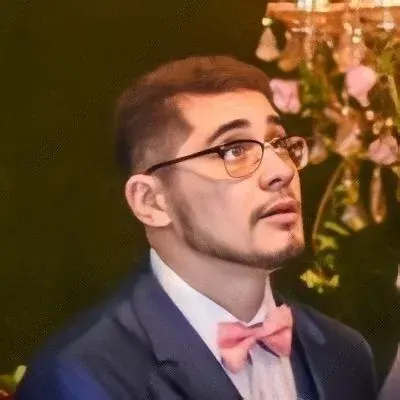
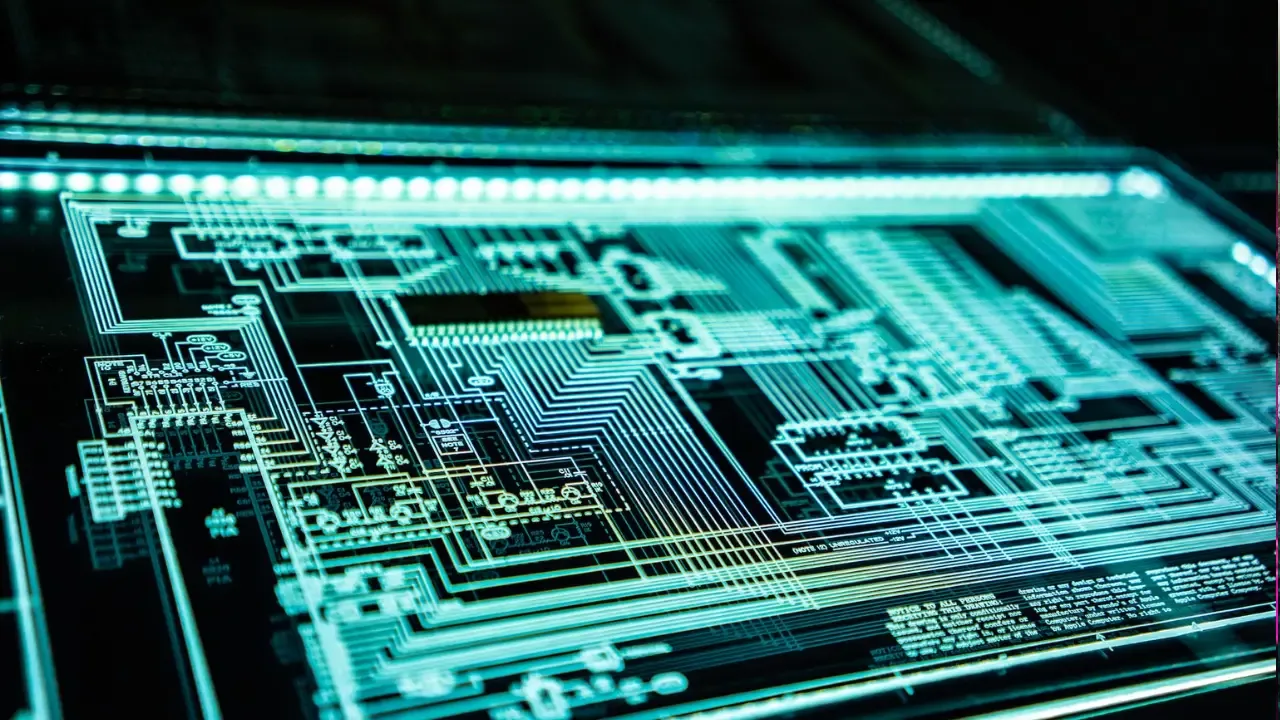
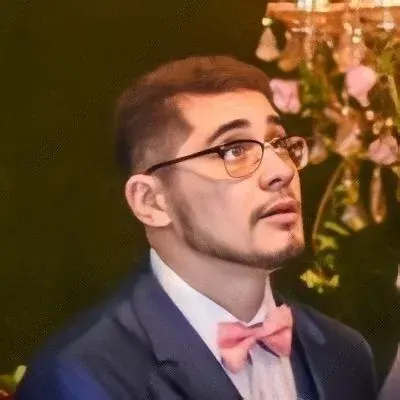
📝 Node.js fs.readFile() - Buffer vs String
Are you puzzled why Node.js's fs.readFile()
returns a buffer instead of a string? 🤔 Don't worry, you're not alone! Many developers have wondered about this too. In this blog post, we'll explore the reasons behind this design decision and provide easy solutions to work with the buffer data. 💪
⚠️ The Buffer Output Mystery
Let's start by taking a look at the code snippet you provided:
var fs = require("fs");
fs.readFile("test.txt", function (err, data) {
if (err) throw err;
console.log(data);
});
You expected it to display the content of test.txt
, which was created using the nano editor and contains the text "Testing Node.js readFile()". However, the output you received was a bit perplexing:
<Buffer 54 65 73 74 69 6e 67 20 4e 6f 64 65 2e 6a 73 20 72 65 61 64 46 69 6c 65 28 29>
🧐 Buffer Explained
The output you see is a buffer representation of the binary data read from the file. But why does Node.js use buffers instead of simply returning a string? 🤷♂️ Let's delve into the reasons:
1️⃣ Efficiency: Buffer objects are more efficient for storing and manipulating binary data compared to strings. Since files can contain any type of data (text, images, videos, etc.), using buffers provides a universal approach to reading and working with file contents.
2️⃣ Binary-Safe Handling: Buffers ensure that no data in the file is unintentionally modified during read or write operations. This characteristic is crucial when dealing with binary file types.
3️⃣ Streaming Capabilities: Node.js emphasizes efficient streaming of data, and buffers play an integral role in that. By using buffers, Node.js can process large files in chunks, avoiding memory overflow issues.
💡 Working with Buffers
Now that we understand why fs.readFile()
returns a buffer, let's explore how to work with it effectively.
1️⃣ Converting Buffer to String
If you want to display the file content as a string, you can use the .toString()
method. Here's an updated code snippet:
var fs = require("fs");
fs.readFile("test.txt", function (err, data) {
if (err) throw err;
console.log(data.toString());
});
Running this code will yield the expected result: "Testing Node.js readFile()".
2️⃣ Accessing Buffer Data
Sometimes, you may need to access the buffer data directly instead of converting it to a string right away. You can achieve this by accessing the individual elements of the buffer. Here's an example:
var fs = require("fs");
fs.readFile("test.txt", function (err, data) {
if (err) throw err;
for (var i = 0; i < data.length; i++) {
console.log(data[i]);
}
});
This code will print each character of the buffer as its corresponding numerical value.
📣 Join the Node.js Community
Node.js is a powerful platform with a vibrant community. Share your experiences working with buffers and learn more from fellow developers on forums, social media, and coding events.
Have you encountered any challenges or exciting use cases with buffers in Node.js? Share your thoughts in the comments below and join the conversation! Let's build a thriving Node.js community together. 🙌
Happy coding! 🚀