Why does my JavaScript code receive a "No "Access-Control-Allow-Origin" header is present on the requested resource" error, while Postman does not?
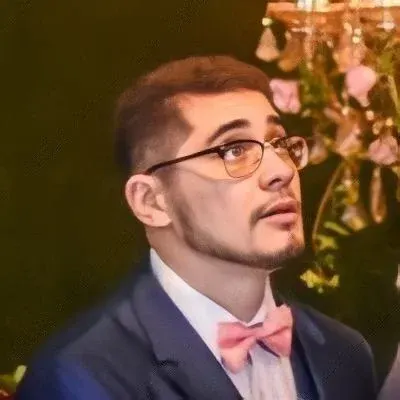
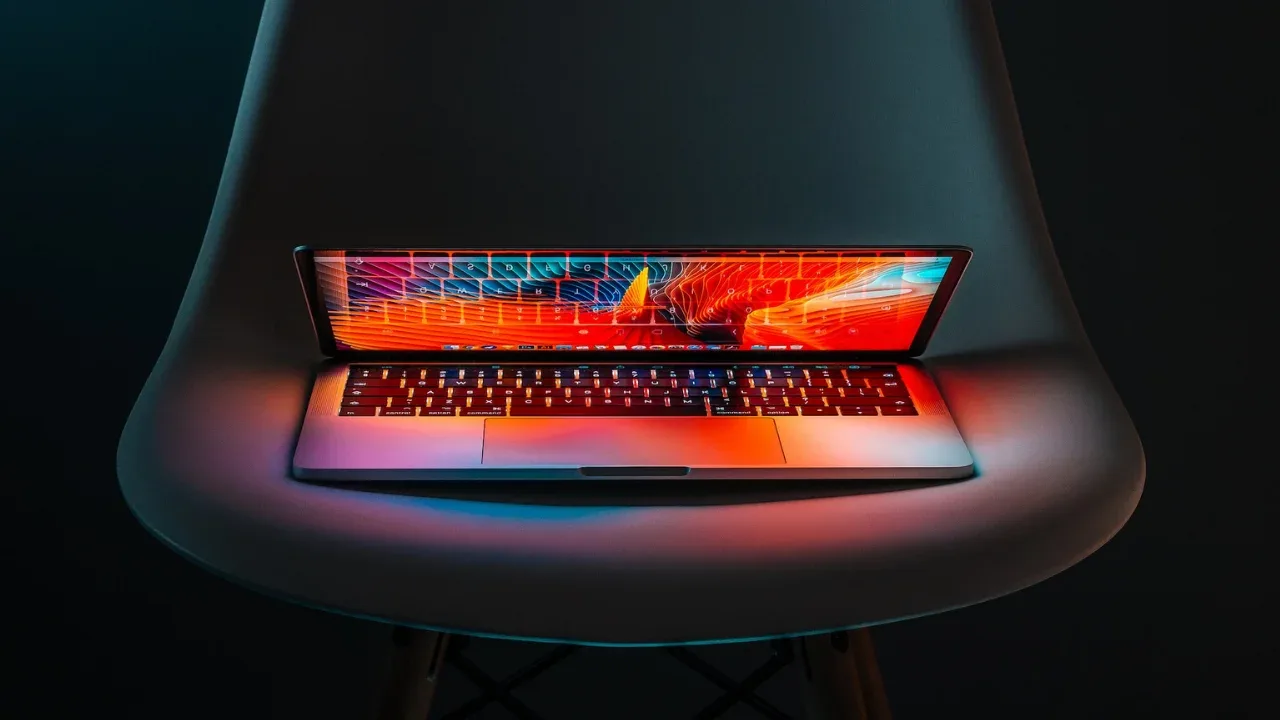
📝 Blog Post: Why does my JavaScript code receive a "No 'Access-Control-Allow-Origin' header is present on the requested resource" error, while Postman does not? 🤔
Are you struggling with a frustrating error message in your JavaScript code that says "No 'Access-Control-Allow-Origin' header is present on the requested resource"? You're not alone! Many developers face this issue when trying to connect to a RESTful API using JavaScript. But here's the twist: why does the request work perfectly fine in Postman, the Chrome extension? That's what we'll explore in this blog post! 🚀
Understanding the CORS Problem
First things first, let's understand what the error message means. The "No 'Access-Control-Allow-Origin' header is present on the requested resource" error is an example of CORS, which stands for Cross-Origin Resource Sharing. CORS is a security feature implemented by browsers to restrict cross-origin requests. It ensures that a web page or application can only make requests to the same origin it has been loaded from, for security reasons.
When your JavaScript code tries to make a request to a different origin (e.g., a different domain or port), the browser blocks the request if the server's response doesn't include the necessary headers. And that's why you encounter this error.
The Postman Magic ✨
Now, you might be wondering, why does Postman work without any issues? 🤔 Unlike web browsers, Postman doesn't have the same restrictions imposed by the Same Origin Policy. It's specifically designed for API testing and allows requests to any origin or domain. That's why you don't face the "No 'Access-Control-Allow-Origin' header is present on the requested resource" error in Postman. Lucky you! 😉
Solutions to Fix the Error
Okay, so now that we understand the problem, let's talk about the solutions. There are a few ways to resolve this error and make your JavaScript code work smoothly:
Enable CORS on the server: The most common and recommended solution is to configure the server to include the 'Access-Control-Allow-Origin' header in its response. This header tells the browser that requests from your web page are allowed. Check the documentation of your backend or API framework to learn how to enable CORS.
Use a proxy server: Another workaround is to set up a proxy server to make the request on behalf of your JavaScript code. The proxy server acts as an intermediary, and since the request is made from the same origin, CORS restrictions don't apply. You can create a simple proxy server using Node.js or choose from existing proxy solutions.
JSONP (JSON with Padding): JSONP is an alternative technique for making cross-origin requests without encountering CORS errors. Instead of using the XMLHttpRequest or fetch API, you can use the
<script>
tag to load JavaScript from a different domain that returns JSON data. However, keep in mind that JSONP has its limitations and might not be suitable for all scenarios.
Remember, these solutions might require some configuration and change in your codebase, so it's crucial to understand the implications and choose the one that best fits your application's needs.
Let's Take Action and Learn More!
Now that you're armed with the knowledge to tackle the "No 'Access-Control-Allow-Origin' header is present on the requested resource" error, it's time to put it into action! Implement one of the aforementioned solutions and watch your JavaScript code run smoothly, just like Postman. 🎉
If you want to dive deeper into web development, CORS, and related topics, check out the following resources:
The Mozilla Developer Network (MDN) CORS Guide - Detailed documentation on CORS, including best practices and advanced techniques.
Stack Overflow CORS Questions - Find solutions to common CORS-related issues asked by developers like yourself.
Remember, sharing is caring! If you found this blog post helpful, spread the knowledge by sharing it with your fellow developers. Let's help each other overcome these roadblocks and create amazing web experiences! 💪
Now it's your turn! Have you encountered the "No 'Access-Control-Allow-Origin' header is present on the requested resource" error? How did you solve it? Share your experiences, tips, and tricks in the comments below. Let's continue the conversation and learn from each other!
Happy coding! 😊🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
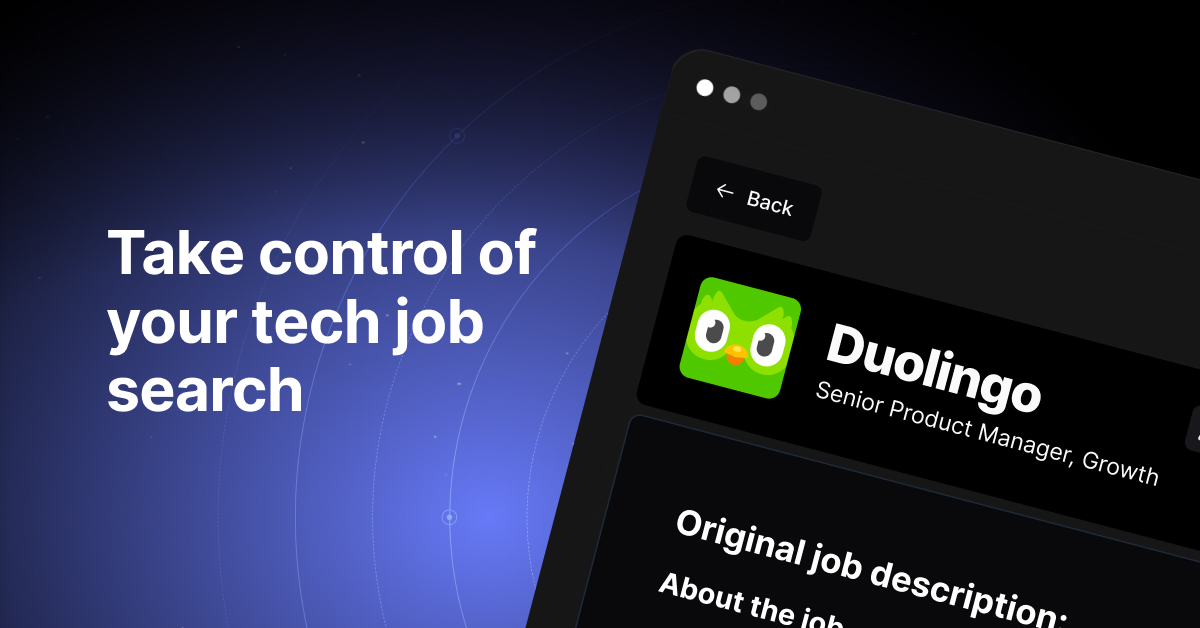