Why does calling react setState method not mutate the state immediately?
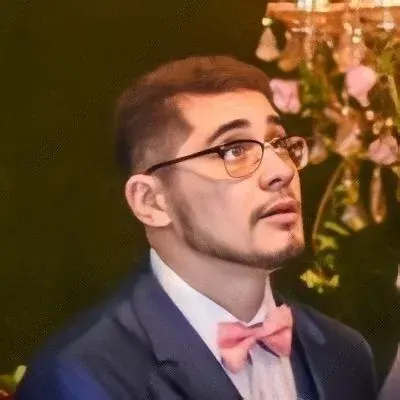
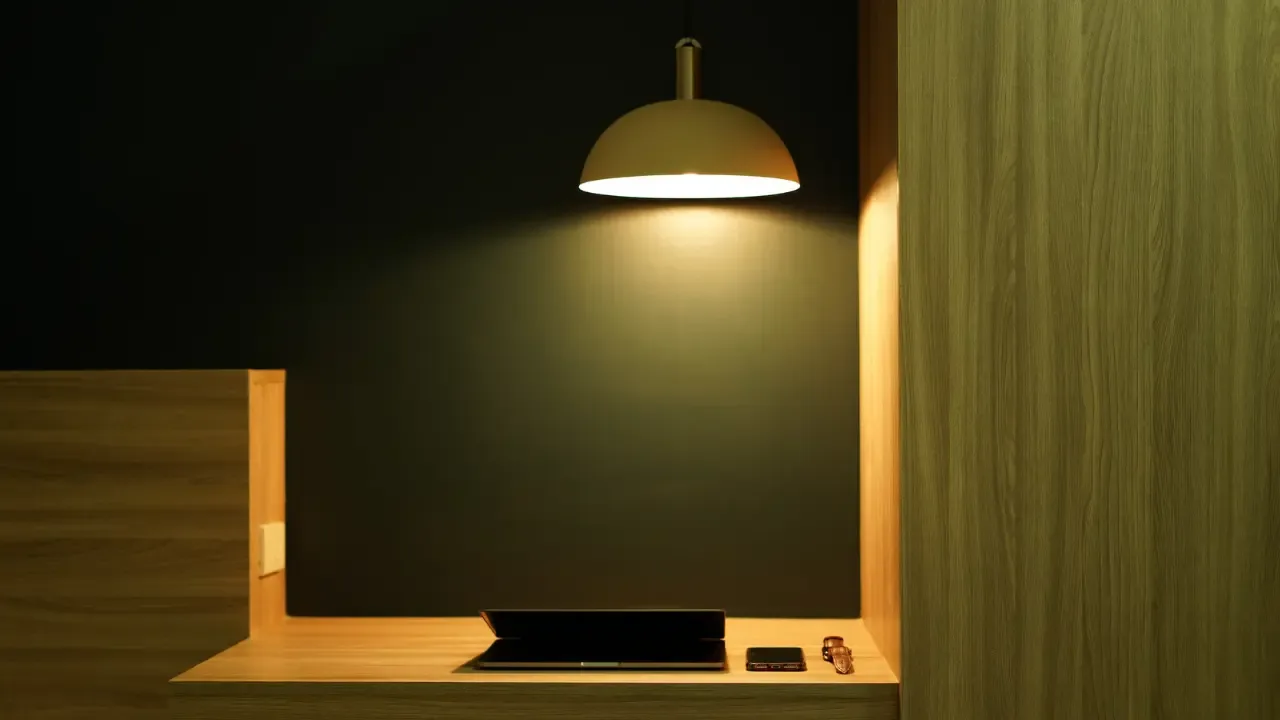
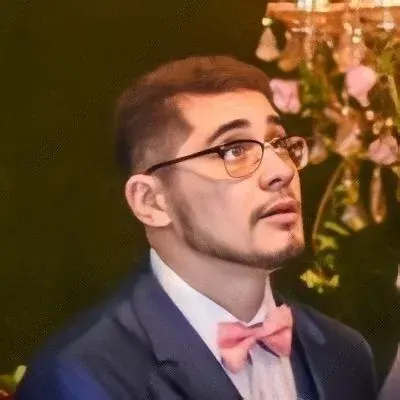
๐ Title: Why doesn't calling React setState method mutate the state immediately?
Introduction: Hey there! ๐Are you a React.js enthusiast who's been wondering why calling the setState method doesn't immediately mutate the state? ๐ค Don't worry, you're not alone! In this blog post, we'll dive into this puzzling question and demystify the reasons behind it. We'll also explore common issues and provide easy solutions for a seamless React.js experience. So, let's get started! ๐ช
Understanding the Issue: To better understand the issue at hand, let's take a look at the code provided. ๐ฅ๏ธ In the code, we have a ControlledForm component with an input field and a handleChange method. When the input's value changes, we log the current value using the state and then update the state using the setState method. However, when we log the value again, it remains the same. ๐ฎ
Explanation: The reason for this behavior is that setState is asynchronous in React.js. ๐ When you call setState, React puts the state update in a queue and then merges it with the current state. The actual state update occurs at a later time when React performs a "reconciliation" process, where it calculates the differences between the new state and the virtual DOM, and then efficiently updates the actual DOM.
Solution: If you need to perform actions after the state has been updated, React provides a callback parameter for the setState method. ๐ You can pass a function as the second argument, which will execute right after the state has been updated. Let's modify our code to include this callback:
this.setState({ value: event.target.value }, () => {
console.log(this.state.value);
});
Now, when you run the code and update the input value, you'll see that the second console.log will print the updated value as expected! ๐
Another Option: React's SyntheticEvent: Another option to access the updated state in the handleChange method is by using React's SyntheticEvent. When you access event.target.value directly, it still refers to the old value since the event is pooled and reused by React.
However, if you change your handleChange method to the following:
handleChange: function(event) {
console.log(event.target.value);
this.setState({ value: event.target.value });
}
You'll notice that event.target.value will correctly display the updated value. ๐
Conclusion: In conclusion, React's setState method doesn't mutate the state immediately because it uses an asynchronous process to update the state. By taking advantage of the callback parameter or using React's SyntheticEvent, you can easily access the updated state and perform any necessary actions. ๐
If you found this blog post helpful, why not share it with your fellow React.js enthusiasts? And don't forget to leave a comment below if you have any questions or further insights. Let's keep the conversation going! ๐