Why does a RegExp with global flag give wrong results?
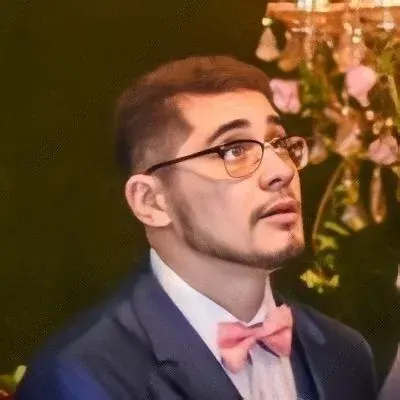
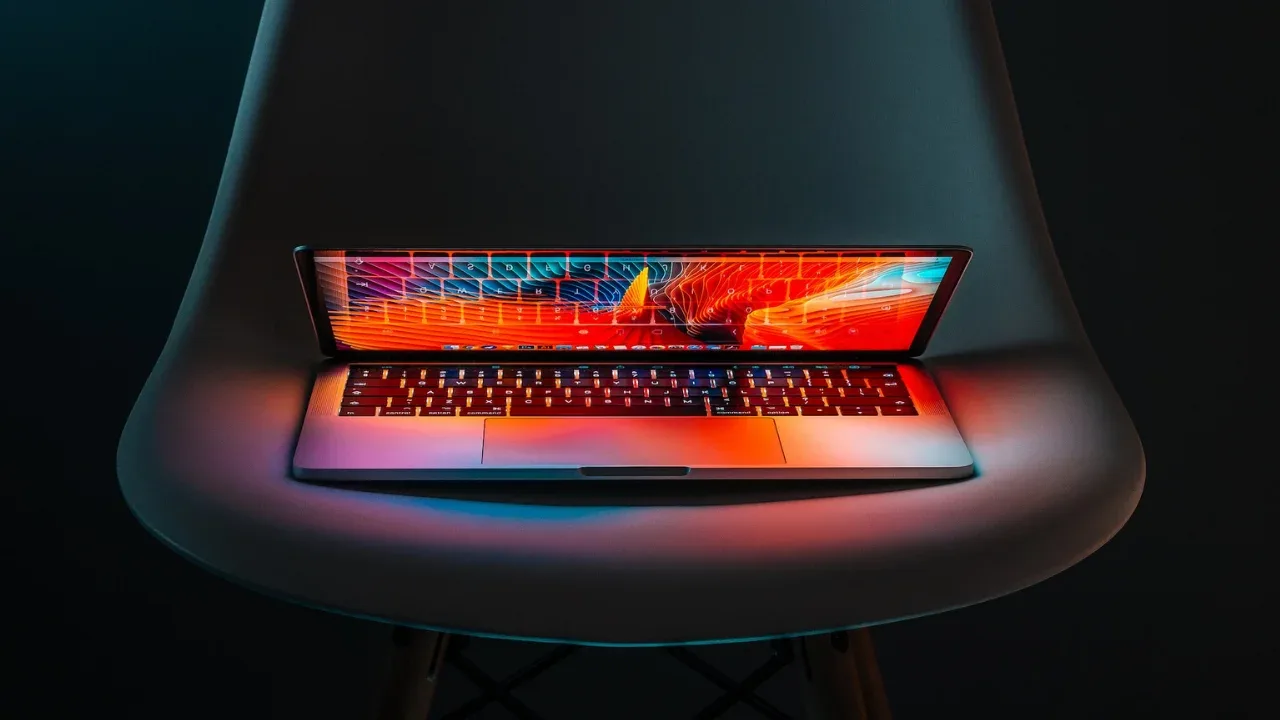
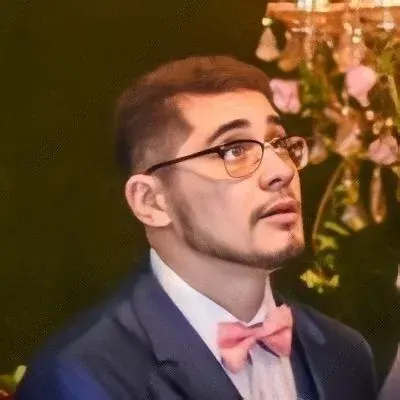
Why does a RegExp with global flag give wrong results?
Have you ever encountered a situation where a regular expression with the global flag (g
) doesn't provide the expected results? 😕 Well, you're not alone! Many developers have faced this issue, and in this post, we will explore the reasons behind this behavior and provide you with easy solutions to fix it.
Let's start by understanding the problem with an example:
var query = 'Foo B';
var re = new RegExp(query, 'gi');
var result = [];
result.push(re.test('Foo Bar'));
result.push(re.test('Foo Bar'));
// Expected result: [true, true]
// Actual result: [true, false]
In this example, we are using a regular expression with both the global (g
) and case-insensitive (i
) flags. Our goal is to find all occurrences of the query
string in the given text and push the results into the result
array.
However, the actual result is not what we expected. The first test returns true
, indicating that 'Foo Bar' contains the matching substring 'Foo B'. But the second test, performed on the same text, returns false
, even though the expected result is true
.
The Reason Behind the Wrong Results
The root cause of this issue lies in the behavior of the RegExp
object when used with the global flag. When a regular expression is created with the global flag (/pattern/g
or new RegExp(pattern, 'g')
), the test()
method keeps track of the index where it found the last match.
In the example above, the test()
method returns true
for the first test because it matches the 'Foo B' substring. However, since the method remembers the index of the match, it starts searching for the next occurrence from that index. In the second test, the search starts from index 6, resulting in a failed match.
Solution: Reinitializing the RegExp Object
To overcome this issue, we need to reinitialize the RegExp
object or create a new one for each test. Here's how we can modify the previous example to get the expected results:
var query = 'Foo B';
var re = new RegExp(query, 'gi');
var result = [];
result.push(re.test('Foo Bar'));
// Reinitialize the 're' variable
re = new RegExp(query, 'gi');
result.push(re.test('Foo Bar'));
// Expected result: [true, true]
// Actual result: [true, true]
By reinitializing the re
variable, we reset the index where the search starts. This ensures that each test()
method call starts searching from the beginning of the text, giving us the expected results.
A Compelling Call-to-Action
Now that you understand why a RegExp with a global flag might give wrong results and how to fix it, go ahead and try these solutions in your own code. Share your experience in the comments below and let us know if you have encountered any other interesting regex challenges!
Don't forget to 👍 this post and share it with your fellow developers who might be struggling with similar issues. Happy coding! 😃🎉