Which keycode for escape key with jQuery
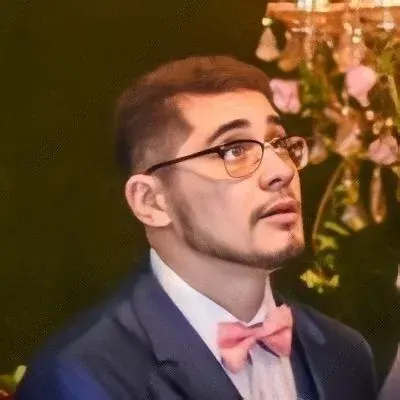
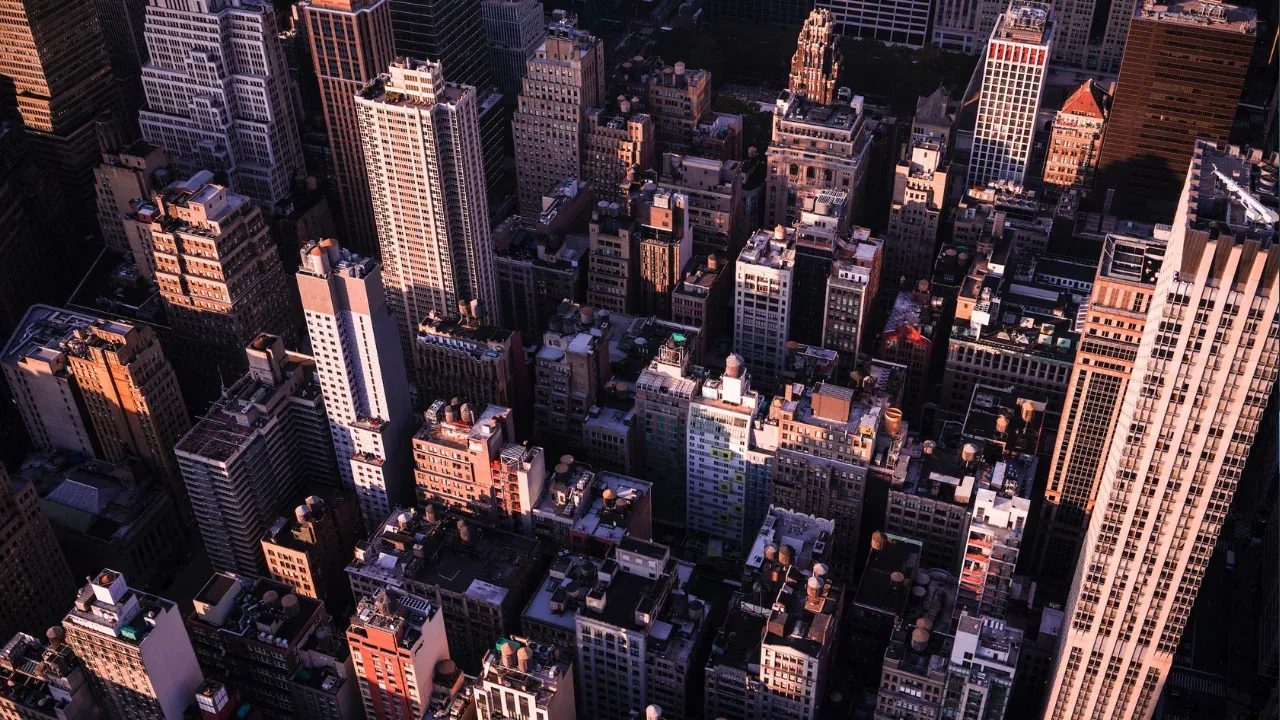
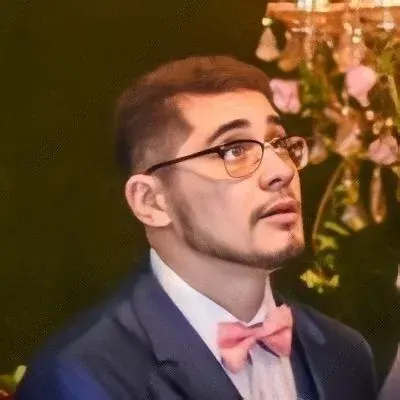
Unlocking the Mystery of the Escape Key with jQuery 🗝️
Have you ever encountered a situation where pressing the Escape key doesn't trigger the expected action in your web application? 🤔 It can be frustrating to deal with, especially when you have everything else working perfectly. But fear not! In this post, we'll delve into the magical world of keycodes and show you how to correctly handle the elusive Escape key using jQuery. 🌟
The Mysterious Case of the Disobedient Escape Key 🕵️♀️
Let's consider a scenario: you have two functions that need to be executed when certain keys are pressed. When the Enter key is pressed, your application's functionality works flawlessly. However, when you press the Escape key, nothing happens! What gives? 😩
Here's a snippet of code that illustrates this problem:
$(document).keypress(function(e) {
if (e.which == 13) $('.save').click(); // enter (works as expected)
if (e.which == 27) $('.cancel').click(); // esc (does not work)
});
In the example above, we're using e.which
to capture the keycode of the pressed key. For the Enter key, it works perfectly fine. But for the Escape key, it fails to trigger the desired action. The culprit? 😈 An incorrect keycode!
Cracking the Code: Understanding Keycodes 🚀
To solve this mystery and make the Escape key behave accordingly, we need to know the correct keycode for it. For the Escape key, the keycode is 27. But how can we ensure that our code handles it correctly? Let's dive into the easy solutions! 💡
Easy Solution 1: Utilizing the keyup Event 🆙
The keypress
event might not capture the Escape key accurately in all cases. Instead, we can use the keyup
event to ensure reliable detection across different browser environments. Let's modify our code to leverage this event:
$(document).keyup(function(e) {
if (e.which == 13) $('.save').click(); // enter (works as expected)
if (e.which == 27) $('.cancel').click(); // esc (now works like a charm)
});
By using keyup
, the Escape key will be accurately detected, ensuring our application's functionality is consistent across different browsers.
Easy Solution 2: Embrace the Power of keydown
⚡
Another reliable way to handle the Escape key is by utilizing the keydown
event. This event is triggered when a key is first pressed down. Let's take a look at how we can adapt our code to make this work:
$(document).keydown(function(e) {
if (e.which == 13) $('.save').click(); // enter (works as expected)
if (e.which == 27) $('.cancel').click(); // esc (works like a charm, yet again)
});
By using keydown
, we can capture the Escape key promptly, ensuring a smooth user experience.
Engage with the Escape Key and Beyond! 💥
The Escape key might have been elusive, but with these easy solutions, you can now handle it like a pro! 🎉 Take a moment to implement the changes in your code and see the magic unfold.
If you found this post helpful or have any questions, feel free to leave a comment below. We love to hear from our fellow developers and help them conquer their coding challenges! Let's unlock the full potential of web development together! 💪💻
Happy coding! 🚀✨