Which equals operator (== vs ===) should be used in JavaScript comparisons?
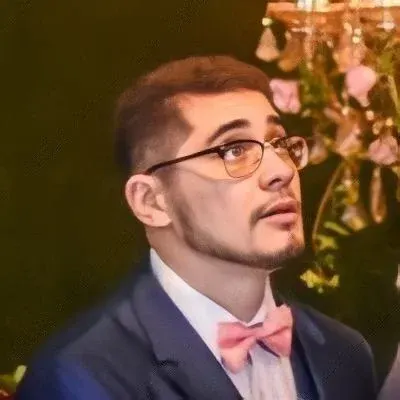
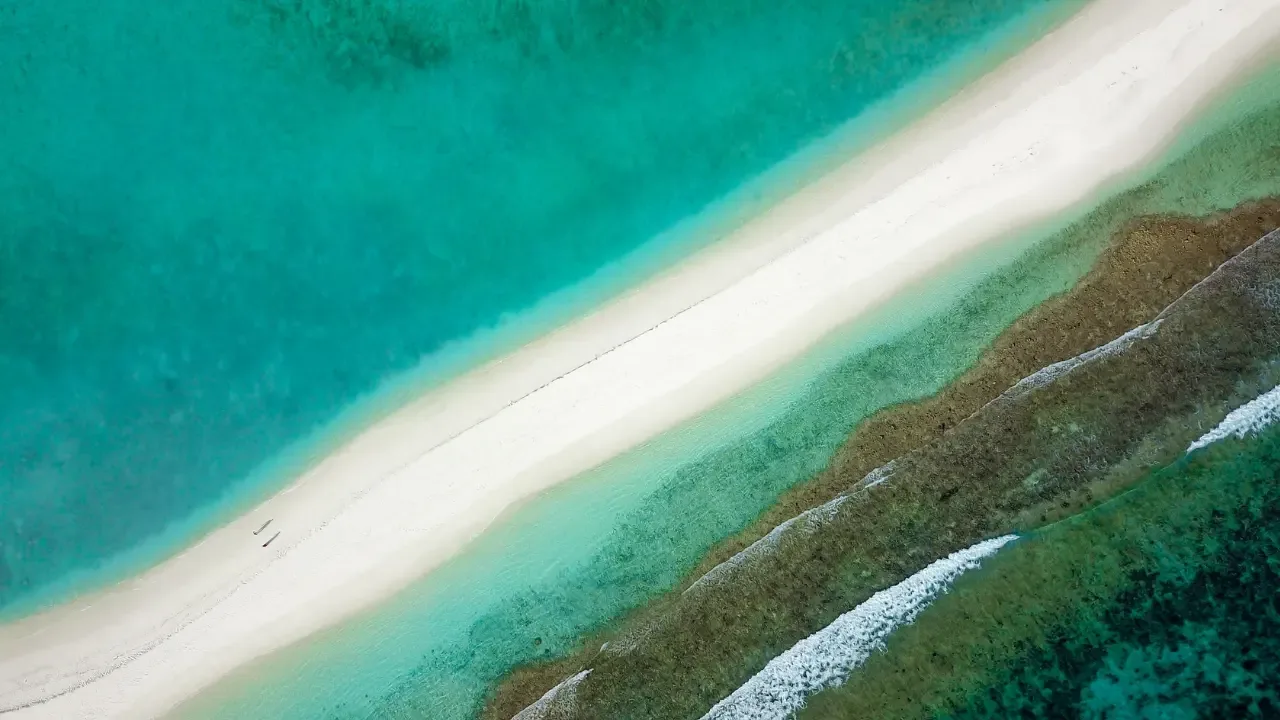
Comparing Operators in JavaScript: To Double Equals or to Triple Equals? 🤔
JavaScript offers two equality operators: ==
(double equals) and ===
(triple equals). Both are used for comparisons, but they have different behaviors and considerations.
Understanding the Difference 🔄
The ==
operator performs loose equality comparisons. It attempts to convert the operands to the same type before making the comparison. On the other hand, ===
performs strict equality comparisons. It checks for both value equality and type equality without any type conversion.
Example 1: Loose Equality Comparison using ==
console.log(5 == "5"); // true
console.log(true == 1); // true
In the above example, JavaScript converts the string "5"
to the number 5
and the boolean true
to the number 1
before making the comparison. This can sometimes lead to unexpected results, especially when dealing with different data types.
Example 2: Strict Equality Comparison using ===
console.log(5 === "5"); // false
console.log(true === 1); // false
In this example, ===
checks not only the values but also the data types. Since the types are different, the comparisons return false.
When to Use Which Operator? 🤔
Now that we understand the difference between ==
and ===
, let's explore when to use each operator:
Use
==
when you need to perform loose equality comparisons and want JavaScript to automatically convert the types.if (idSele_UNVEHtype.value.length == 0) { // Perform some action }
In the above example,
==
allows for type coercion, making it convenient for scenarios where you want to compare values of potentially different types.Use
===
when you need to perform strict equality comparisons and want to ensure both value and type matches.if (idSele_UNVEHtype.value.length === 0) { // Perform some action }
Here,
===
provides more precise control over the comparison and helps in avoiding unintended results caused by type coercion.
Performance Considerations ⚡️
The question above also touches on the performance aspect of ==
vs ===
. Generally, there is a negligible performance difference between the two operators. Modern JavaScript engines are optimized to handle both efficiently.
However, it's worth noting that strict equality comparisons (===
) tend to be faster than loose equality comparisons (==
). This is because there is no need for type coercion in strict comparisons, which saves some processing time.
It's important to prioritize code readability and maintainability over micro-optimizations for performance gains. Unless you are dealing with massive and repeated comparisons, the performance impact will be minimal.
Conclusion: Be Conscious of Your Comparison! 🧐
To sum it up, choosing between ==
and ===
depends on your specific use case. If you need type coercion or want to compare values of different types, ==
is a suitable choice. However, for strict comparisons where both the values and types should match, ===
is the way to go.
Always prioritize code clarity and consistency to avoid hard-to-find bugs caused by unexpected type conversions! Remember, it's about writing code that is not only functional but also maintainable for you and your team.
Let us know your thoughts in the comments below. Which operator do you lean towards when comparing values in JavaScript?
🔽🔽🔽 Keep scrolling to leave your comment! 🔽🔽🔽
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
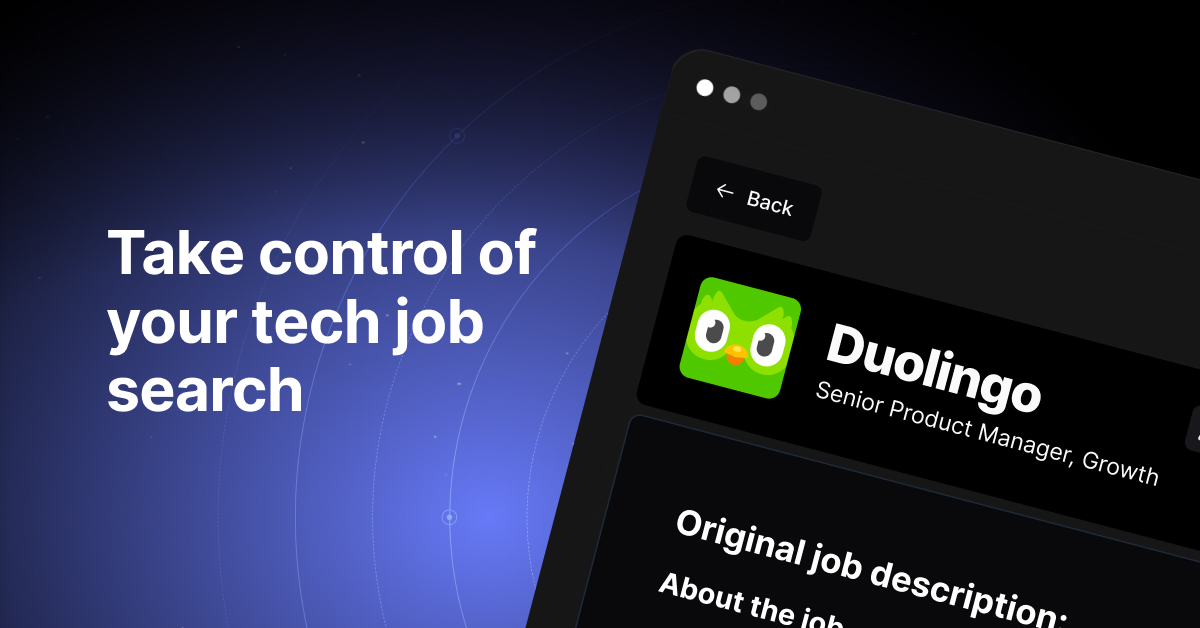