Where to put model data and behaviour? [tl; dr; Use Services]
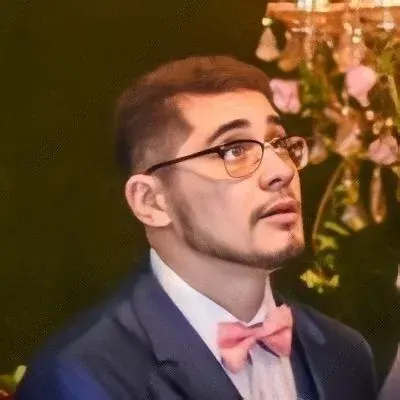
![Cover Image for Where to put model data and behaviour? [tl; dr; Use Services]](https://images.ctfassets.net/4jrcdh2kutbq/4LzpjqnGluxbuQcWmbAGX0/5d51a487df5b06a3f30146f2e7a52efb/Untitled_design__3_.webp?w=3840&q=75)
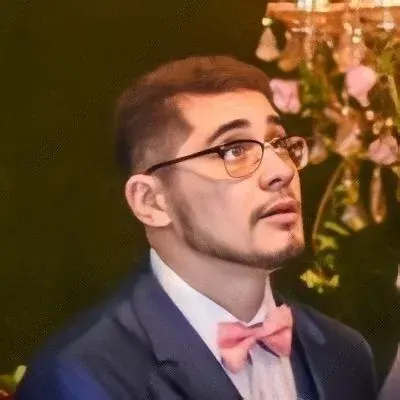
📝 Where to put model data and behaviour? (tl;dr: Use Services) 🤔
Are you working with AngularJS for your latest project? 🖥️ If so, you might have noticed that in the documentation and tutorials, all model data is put into the controller scope. 🎛️ While this makes it available for the controller and corresponding views, it might not be the best practice for implementing the actual model. 🙅♂️
Putting everything into the controller breaks the MVC pattern, and it can get complex and difficult to manage, especially if you want to reuse the model in another context or app. 😱
So, how can we implement models decoupled from the controller, while following AngularJS best practices? 🤔
One way to achieve this is by using services. 💡 Services in AngularJS provide a way to share data and behavior across multiple components, making them a perfect fit for implementing decoupled models. 🤝
Here's how you can do it:
Create a new service:
app.service('myModel', function() { // Your model implementation goes here });
Define your model data and behavior within the service:
app.service('myModel', function() { this.data = { // Your model data goes here }; this.behavior = function() { // Your model behavior goes here }; });
Inject the service into your controller:
app.controller('myController', function($scope, myModel) { // Access the model data and behavior using myModel $scope.data = myModel.data; $scope.behavior = myModel.behavior; });
By using a service, you can now keep your model data and behavior separate from the controller, following the principles of the MVC pattern. 🎉
Not only does this lead to cleaner and more maintainable code, but it also allows for easy reuse of the model in different contexts or apps. 🔄
So why not give it a try? Implement your models using services, and witness the magic of decoupling in your AngularJS projects! 💫
Got any questions or suggestions on implementing models in AngularJS? Share them in the comments below! Let's dive into the world of decoupled models together! 💪
💡💬🔧