When to use ES6 class based React components vs. functional ES6 React components?
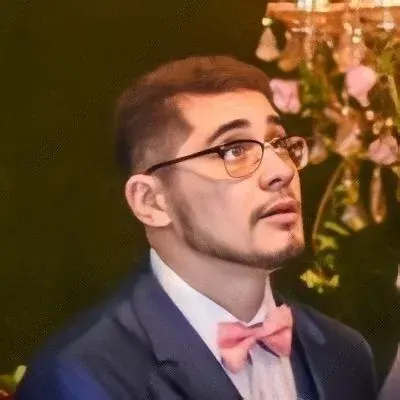
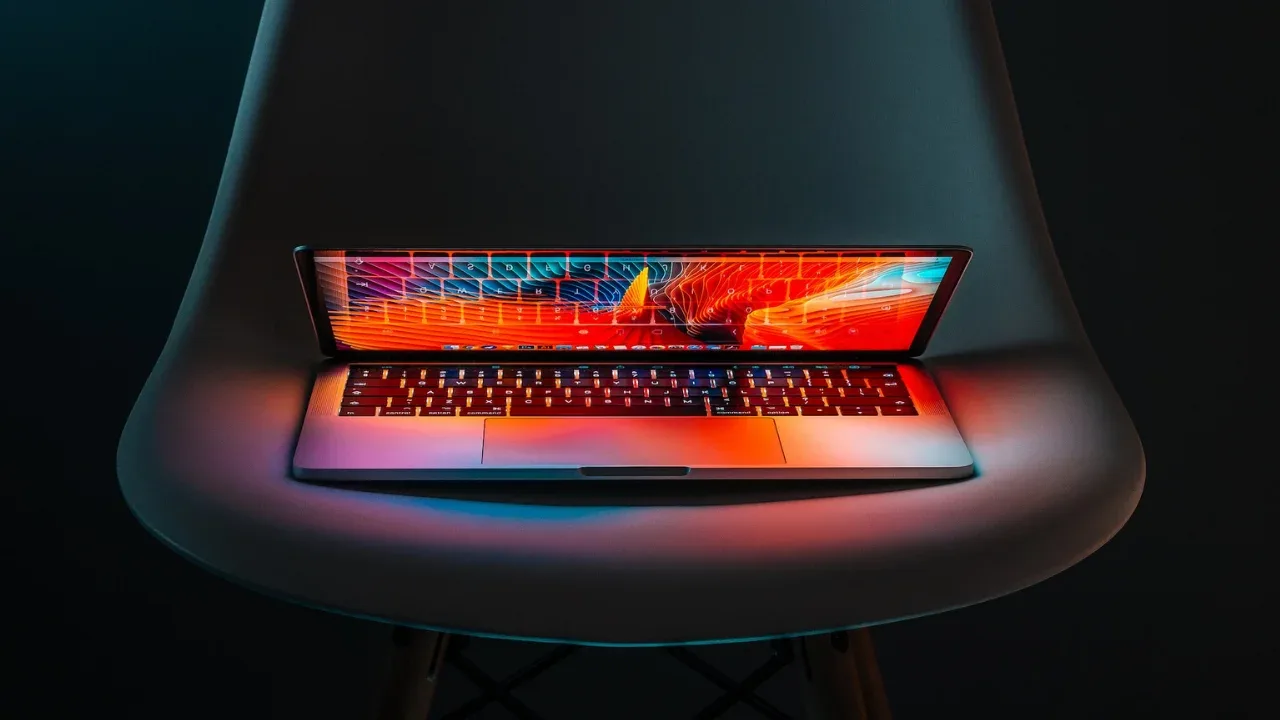
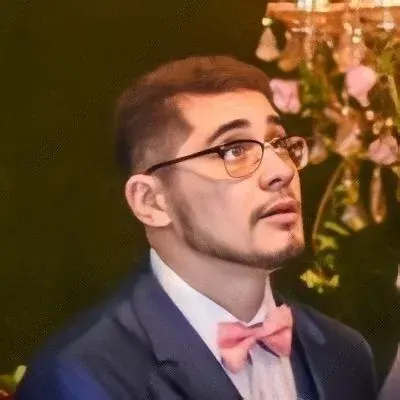
When to use ES6 class based React components vs. functional ES6 React components?
<p>React offers two main paradigms for creating components: ES6 class based components and functional ES6 components. Understanding when to use each one is key to writing efficient and maintainable code.</p>
ES6 Class Based Components
<p>ES6 class based components are created by extending the `Component` class from the `react` package. They are ideal for complex components that require managing state and using lifecycle methods.</p>
Benefits
State Management: By using class based components, you have access to the
state
object which allows you to manage component-level state. This is important when your component needs to handle user interactions or maintain its own state.Lifecycle Methods: Class based components have access to various lifecycle methods such as
componentDidMount
,componentDidUpdate
, andcomponentWillUnmount
. These methods provide hooks to handle component initialization, updating, and cleanup.
Example
import React, { Component } from 'react';
export class MyComponent extends Component {
render() {
return (
<div></div>
);
}
}
Functional Components
<p>Functional components are created using JavaScript functions. They are simpler and more lightweight than class based components, making them suitable for simple presentational components that don't require state management or lifecycle methods.</p>
Benefits
Simplicity: Functional components have a simpler syntax and are easier to read and understand. They also typically have a smaller file size compared to class based components.
Performance: Since functional components do not have state or lifecycle methods, they are generally faster to render than class based components. They are a good choice for components that only need to render UI elements.
Example
const MyComponent = (props) => {
return (
<div></div>
);
}
Choosing the Right Component
<p>When deciding between ES6 class based components and functional components, consider the following guidelines:</p>
State Management: If your component needs to manage state or handle user interactions, ES6 class based components are the way to go. They provide a clear mechanism for updating and mutating state efficiently.
Lifecycle Methods: If your component requires lifecycle methods to initialize or maintain its behavior, choose ES6 class based components. Lifecycle methods allow you to perform actions at specific points during a component's lifecycle.
Simplicity: If your component is simple and doesn't require state management or lifecycle methods, functional components are a good choice. They have a smaller learning curve and are more straightforward to work with.
Performance: If performance is a top concern and you don't need state management or lifecycle methods, consider using functional components. They are often faster to render and have a smaller overhead.
Remember, there is no strict rule on when to use each type of component. It ultimately depends on your specific use case and the requirements of your component. React is designed to be flexible and accommodate different approaches.
Conclusion
<p>Understanding when to use ES6 class based React components versus functional React components is essential for writing clean and efficient code. By considering factors such as state management, lifecycle methods, simplicity, and performance, you can make an informed decision on which type of component to use for a given situation.</p>
<p>So, the next time you're creating a new React component, think about its requirements and choose the right option: ES6 class based or functional. And as always, happy coding! 😄</p>
<hr>
Have any questions or insights to share about React components? Let's discuss in the comments below! 👇
<p>For more awesome React tips and tutorials, don't forget to subscribe to our newsletter!</p>