What"s the difference between `useRef` and `createRef`?
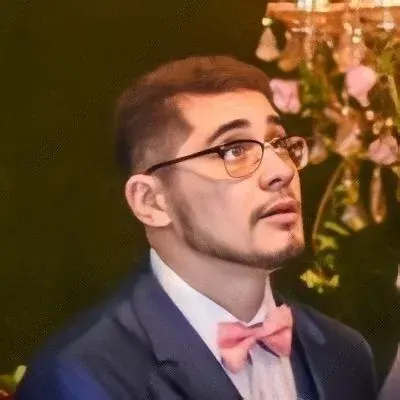
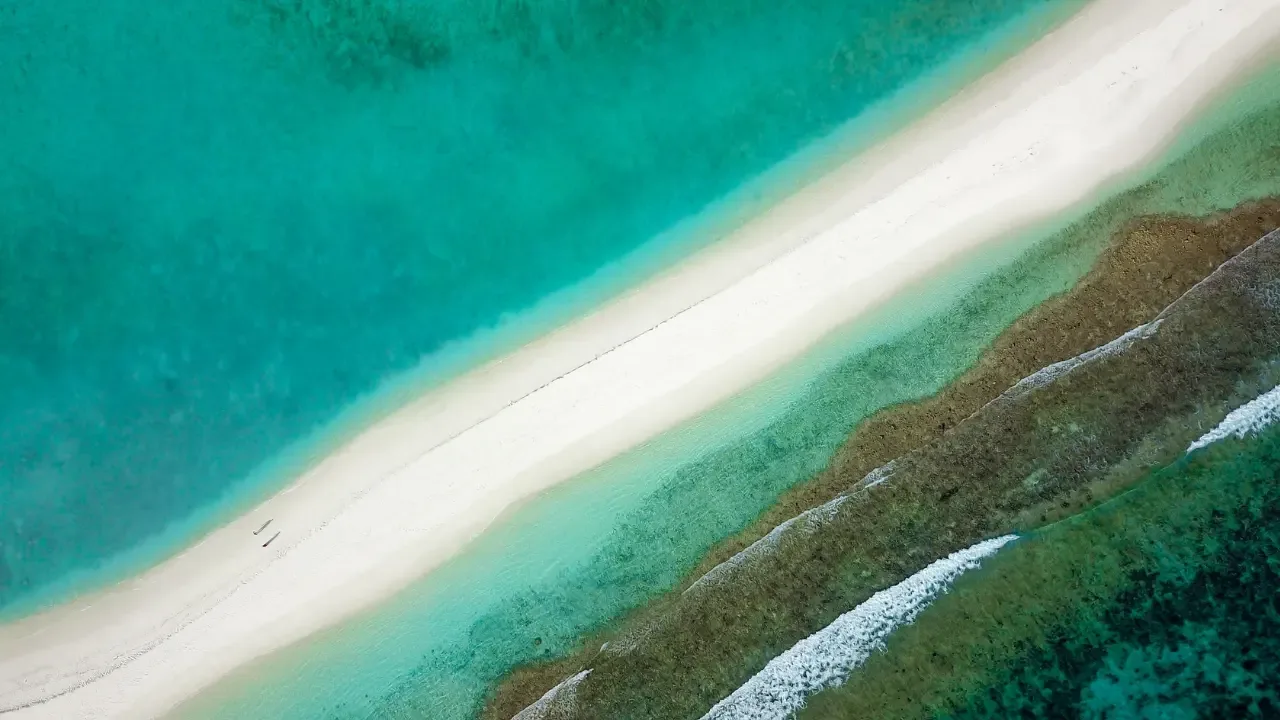
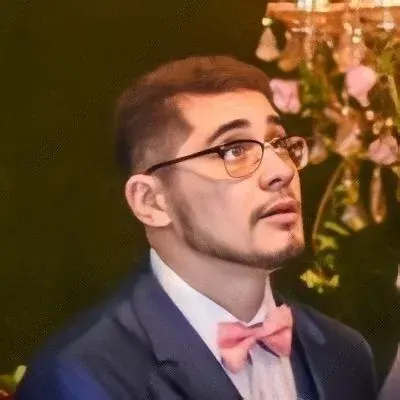
The Mystery of useRef and createRef: Demystified! š§
š Hey there, tech aficionados! Welcome to my blog, where we unravel and clarify the confusing concepts of the tech world. Today, we are diving into the enigma of useRef
and createRef
in React Hooks. šµļøāāļø
š¤ The Dilemma: useRef or createRef?
If you've delved into the wonderful world of React and stumbled upon the tantalizing useRef
, you might wonder - do I really need this hook when I have createRef
? š¤·āāļø
So, let's take a closer look at these two, shall we? Let's crack this puzzling mystery together! š
createRef
- The Traditional Ref
createRef
is often used in class components to create a ref that can be attached to DOM elements or React components. It has been around in React for quite a while. Let's take a look at a quick example to refresh our memory:
class TextInputWithFocusButton extends React.Component {
constructor(props) {
super(props);
this.inputRef = React.createRef();
}
onButtonClick = () => {
// `current` points to the mounted text input element
this.inputRef.current.focus();
};
render() {
return (
<>
<input ref={this.inputRef} type="text" />
<button onClick={this.onButtonClick}>Focus the input</button>
</>
);
}
}
In the above code, createRef
is used to create a ref called inputRef
, and later in the onButtonClick
method, we use this.inputRef.current
to access the DOM input element and give it focus when the button is clicked.
š So, in a nutshell, createRef
is used in class components to create a ref that remains constant throughout the component's lifecycle.
Now let's proceed to the new kid on the block, useRef
. š
useRef
- The Agile Ref
With the introduction of React Hooks, useRef
swooped in to provide a more flexible and functional approach to managing refs in functional components. Let's examine how useRef
can be utilized:
function TextInputWithFocusButton() {
const inputRef = React.useRef(null);
const onButtonClick = () => {
// `current` points to the mounted text input element
inputRef.current.focus();
};
return (
<>
<input ref={inputRef} type="text" />
<button onClick={onButtonClick}>Focus the input</button>
</>
);
}
In this scenario, useRef
creates a ref called inputRef
(initialized with null
), ensuring it remains persistent across different renders of the component. We can access and modify the ref's value using inputRef.current
.
š” The key difference here is that while createRef
in class components requires initialization inside the constructor, useRef
in functional components can be initialized directly inside the component body. This flexibility makes it a superior choice in most cases.
š The Real Difference
Now you might be wondering, "Why should I bother with useRef
when I already have createRef
?" Let's weigh the two options:
ā
Performance: useRef
has a slight performance advantage over createRef
due to its lightweight nature. However, the difference is usually negligible in most scenarios.
ā
Flexibility: With useRef
, you can update the ref value without triggering a re-render of the component, allowing more agility in managing component state. This feature is particularly beneficial in performance optimizations and managing imperative DOM operations.
ā
Hooks Consistency: By solely using useRef
in functional components, you maintain a consistent and unified approach, avoiding codebase inconsistencies between functional and class components.
š” The Conclusion
So here's the bottom line: if you're working on a class component, createRef
is still your trusted confidant. However, if you embrace the lightweight wonders of functional components, I strongly recommend using useRef
to unlock a broader range of possibilities and maintain a clean and concise codebase.
š But hey, don't just take my word for it! Dive in, experiment, and see which suits your project's unique needs. Happy coding, my fellow techies! š»š„
Have any thoughts or questions? I'd love to hear from you! Share your insights in the comments section below and let's ignite a lively conversation. š£ļøš¬
Keep exploring, keep learning! Until next time, tech enthusiasts! āļø