What"s the difference between returning value or Promise.resolve from then()
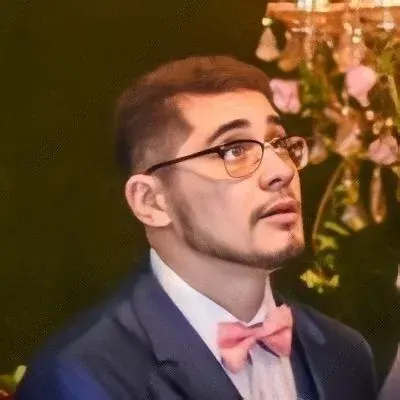
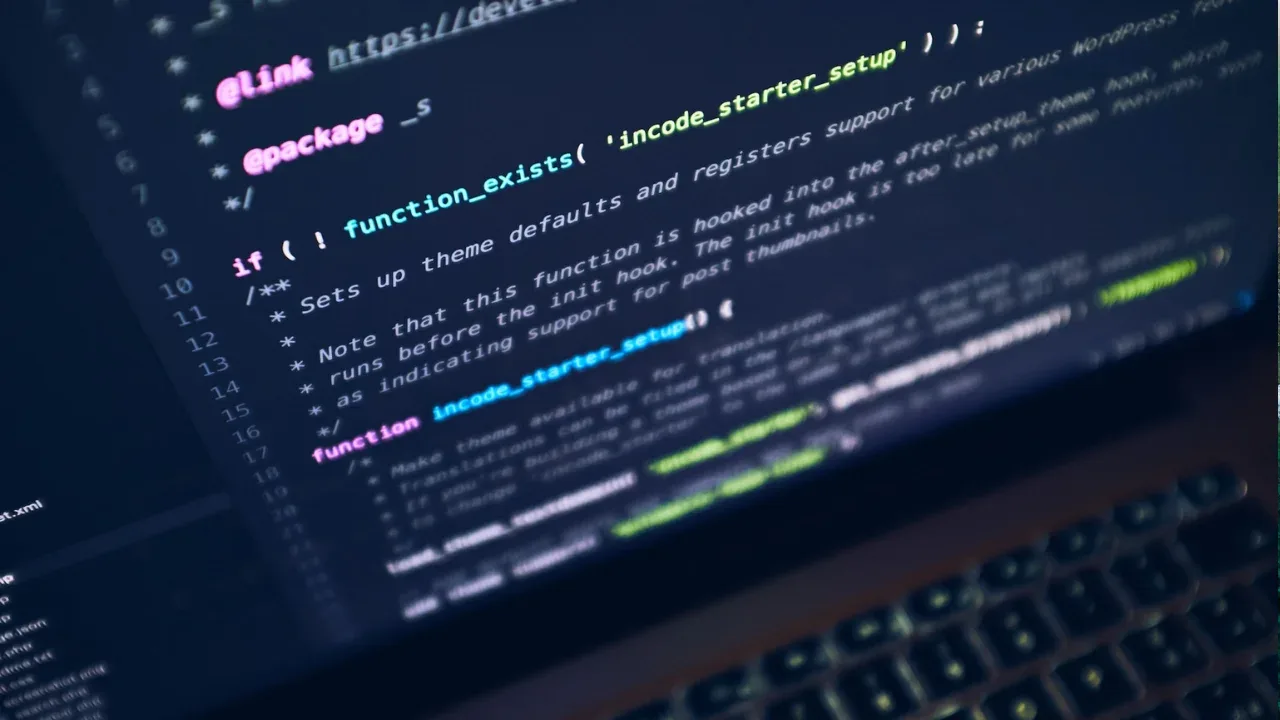
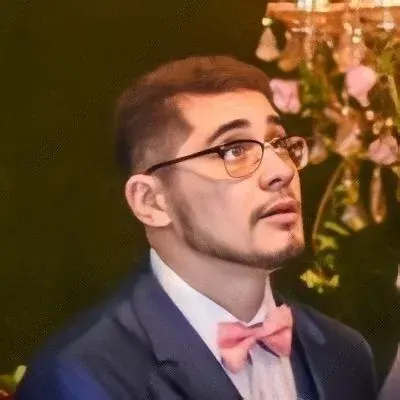
Understanding the Difference between Returning a Value and Promise.resolve in .then()
So, you're facing some issues with Angular and the $http service while using chaining .then(). You've noticed two different approaches when it comes to returning values or using Promise.resolve in the .then() method. Let's dive into this issue and uncover the difference between them.
Code Examples
Here are the two examples, showcasing the difference between returning a value and using Promise.resolve in .then():
// Example 1: Returning a Value
new Promise(function(res, rej) {
res("aaa");
})
.then(function(result) {
return "bbb"; // directly returning a string
})
.then(function(result) {
console.log(result);
});
And here's the second approach:
// Example 2: Using Promise.resolve
new Promise(function(res, rej) {
res("aaa");
})
.then(function(result) {
return Promise.resolve("bbb"); // returning a promise
})
.then(function(result) {
console.log(result);
});
Understanding the Difference
In Example 1, "bbb" is directly returned as a simple value from the first .then() callback. This means that the next .then() in the chain receives "bbb" as its input.
On the other hand, in Example 2, Promise.resolve("bbb") is returned from the first .then() callback. This means that the next .then() in the chain receives a Promise that resolves to "bbb".
Why does it matter?
The difference between returning a value and using Promise.resolve might seem subtle, but it can have important implications, especially when dealing with asynchronous operations.
By returning a value directly, you can pass the value synchronously to the next .then() in the chain. This is suitable for scenarios where you have a simple value and don't need any extra asynchronous processing.
On the other hand, using Promise.resolve allows you to chain the next .then() with a Promise instead of a simple value. This becomes useful when you need to perform additional asynchronous operations within the .then() chain.
Solutions and Best Practices
Returning a value: If you have a simple value and don't require additional asynchronous processing, directly returning the value is a straightforward approach. This keeps your code concise and easy to follow.
Using Promise.resolve: If you need to perform further asynchronous operations within the .then() chain, returning a Promise using Promise.resolve is the way to go. This ensures proper sequencing and synchronization of operations.
Call-to-Action
Next time you encounter issues with chaining .then() in Angular or any other framework, take a moment to verify whether returning a simple value or using Promise.resolve is the appropriate approach.
Remember, a simple value directly returned works well for synchronous scenarios, while using Promise.resolve allows you to handle additional asynchronous operations within the .then() chain.
So, experiment with both approaches and see how they work in your specific use case. Have fun coding, and don't forget to share your experiences and any other interesting discoveries in the comments below!
🚀 Keep coding and keep learning! 🎉