What"s the best way to retry an AJAX request on failure using jQuery?
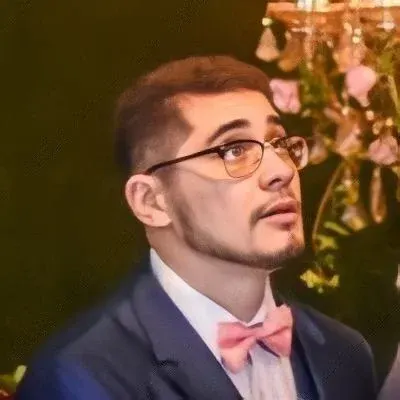

💡 Retry AJAX Requests Like a Pro with jQuery
Have you ever encountered a frustrating situation where an AJAX request fails and leaves your users hanging? 😫 Fear not, because today we're going to dive into the best way to retry an AJAX request on failure using jQuery!
The Problem: AJAX Request Failures 🚫
When working with AJAX requests, it's not uncommon to come across failures due to various reasons, such as network issues or server problems. These failures can disrupt the user experience and leave them hanging without the desired information or action. That's where retrying the request comes into play!
The Solution: Retry and Exponential Back-off ⏰
To retry an AJAX request on failure using jQuery, we can utilize the ajaxError
event handler. This nifty little function allows us to capture any AJAX errors thrown during the request and perform additional actions.
Let's take a look at the code snippet you provided:
$(document).ajaxError(function(e, xhr, options, error) {
xhr.retry()
})
This code snippet attaches an event handler to the ajaxError
event of the document
object. Whenever an AJAX request encounters an error, this function will be triggered. However, there's a small issue with this code – the retry()
method doesn't actually exist in jQuery. 😬
To implement retries, we'll need to employ some additional techniques. One popular approach is implementing exponential back-off. This means that instead of immediately retrying the request, we progressively increase the delay between retries, giving the server more time to recover.
Here's an enhanced version of the code that incorporates exponential back-off:
let maxRetries = 3;
let retryDelay = 1000; // 1 second
$(document).ajaxError(function(e, xhr, options, error) {
if (xhr.status === 0 || Math.floor(xhr.status / 100) === 5) {
if (maxRetries > 0) {
setTimeout(function() {
$.ajax(options);
maxRetries--;
retryDelay *= 2;
}, retryDelay);
} else {
alert("Sorry, we're experiencing server issues. Please try again later.");
}
}
});
In this code snippet, we introduced two variables, maxRetries
and retryDelay
. maxRetries
defines the maximum number of retries we're willing to attempt, and retryDelay
represents the initial delay between retries.
Upon encountering an AJAX error, we check if the error is due to a server-related issue. If so, we schedule a retry after the specified delay using setTimeout
. We also reduce the maxRetries
count by one and double the retryDelay
, ensuring the exponential back-off effect.
If we exhaust all retries without success, we notify the user with a helpful message.
Time to Level Up! ⚡️
Now that you know how to retry AJAX requests on failure using jQuery and even implement exponential back-off, it's time to level up your skills! You can customize the code snippet to meet your specific needs, such as adjusting the maximum retries or initial delay.
Feel free to experiment with different retry strategies, such as using different back-off algorithms or dynamically adjusting the delay based on response times.
Remember, coding is all about continuous learning and improvement! ✨
Share Your Success Story! 🎉
Did you successfully implement AJAX request retries using jQuery in your project? We would love to hear your success stories and any challenges you faced along the way. Share your thoughts and experiences in the comments below!
Let's strive for resilient applications that never let our users down. Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
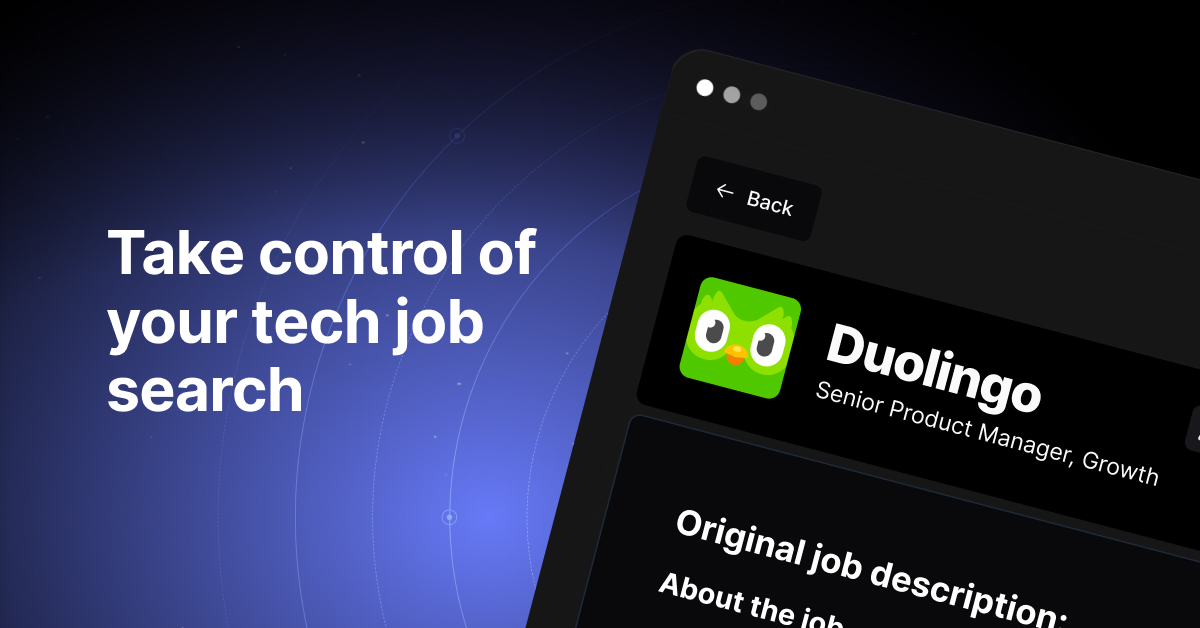