Whatβs the difference between "Array()" and "[]" while declaring a JavaScript array?
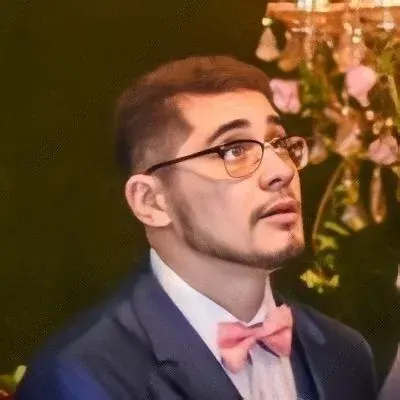
![Cover Image for Whatβs the difference between "Array()" and "[]" while declaring a JavaScript array?](https://images.ctfassets.net/4jrcdh2kutbq/7cZyndlm0F3cClG6jnlxP/0f5c7f1adcd51bf8800b707ba8d88d19/Untitled_design__1_.webp?w=3840&q=75)
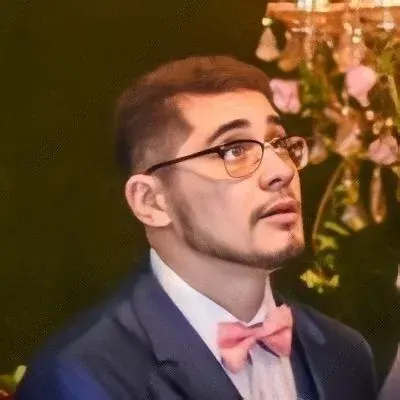
Array() vs []
Are you confused about how to declare an array in JavaScript? π€ Don't worry, you're not alone! Many developers wonder about the difference between Array()
and []
when declaring a JavaScript array. In this blog post, we will address this common question, explain the key differences, and provide easy solutions to help you understand and use arrays effectively. Let's dive in! π
The Basics: Declaring an Array
In JavaScript, an array is used to store multiple values in a single variable. You can declare an array using either the Array()
constructor or the shorthand []
notation. Both approaches will create an empty array, but there are some significant differences between them. Let's take a closer look at each method.
Using the Array()
Constructor
var myArray = new Array();
When you use the Array()
constructor to declare an array, you are calling a built-in JavaScript function that creates a new instance of the Array
object. The new
keyword is necessary to initialize the array. This method allows you to specify the initial size of the array as a parameter.
Using the []
Notation
var myArray = [];
The []
notation is the preferred and more commonly used way to declare an array in JavaScript. It is shorter, simpler, and more intuitive. When you use this notation, you directly assign an empty array to the variable without invoking the Array
constructor explicitly.
The Key Differences
At first glance, both methods seem to accomplish the same thing β creating an empty array. However, there are a few differences worth noting:
Creating Arrays with Specific Length:
The
Array()
constructor allows you to define an array size by passing an integer argument. For example:var myArray = new Array(5); // Creates an array with length 5
However, if you provide a non-integer argument or multiple arguments, the
Array()
constructor will create an array with those elements:var myArray = new Array(1, 2, 3); // Creates an array with elements [1, 2, 3] var myArray = new Array("Hello"); // Creates an array with a single element ["Hello"]
On the other hand, the
[]
notation does not support specifying the length directly. It always creates an array without any predefined length.Potential Confusion with Arguments:
As shown in the previous point, when using the
Array()
constructor with multiple arguments, it creates an array with those values. This can lead to unexpected results and potential confusion. To avoid this ambiguity, it is recommended to use the[]
notation to create arrays with specific elements.var myArray = [1, 2]; // Creates an array with elements [1, 2]
By using the
[]
notation, the intention of initializing the array with specific elements becomes clear and unambiguous.Compatibility:
The
[]
notation was introduced in ECMAScript 3 (ES3), which is supported by all modern browsers. In contrast, theArray()
constructor has been available since the earlier versions of JavaScript. However, using the[]
notation is generally considered best practice for creating arrays.
Easy Solutions
Now that you understand the key differences between Array()
and []
, how should you declare an array? The answer is simple: use the []
notation!
The []
notation is more concise, widely accepted, and less prone to confusion. It is also compatible with all modern JavaScript environments. If you need to create an array with specific elements, simply assign them using the []
notation:
var myArray = [1, 2, 3];
If you want to create an array with a specific length, you can leverage the Array.from()
method or the fill()
method:
var myArray = Array.from({ length: 5 });
// Creates an array with length 5, filled with undefined
var myArray = Array(5).fill(0);
// Creates an array with length 5, filled with zeros
Your Turn!
Now that you've learned the difference between Array()
and []
when declaring a JavaScript array, it's time to put your knowledge into practice! π
Next time you need to create an array, remember to use the []
notation for simplicity, clarity, and compatibility. Feel free to experiment with different scenarios and explore more advanced array operations to enhance your JavaScript skills.
If you have any questions, comments, or other JavaScript array declarations to share, drop them in the comments below. Let's get the conversation started! π¬
Happy coding! π©βπ»π¨βπ»
(Image source: Unsplash.com)
π£ Call to Action:
Enjoyed this article? Want to learn more about JavaScript and web development? Join our vibrant community! Subscribe to our newsletter to receive the latest updates, tutorials, and tips straight to your inbox. Don't miss out! π₯
βοΈ Subscribe Now: [Enter Your Email Address]
π₯ Follow Us: [Twitter] | [Facebook] | [Instagram]
π‘ More Resources:
Disclaimer: The information provided in this blog post is for educational purposes only. Please refer to official documentation and consult with professionals for any critical implementations.