What is the scope of variables in JavaScript?
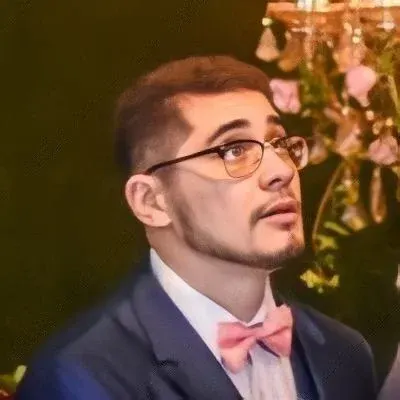

Understanding the Scope of Variables in JavaScript 🤔
Hey there, tech-savvy peeps! 👋
Welcome back to our tech blog, where we unravel complex concepts into bite-sized, easily digestible pieces. Today, we're diving into the depths of JavaScript variables and their scope. 🚀
The Tale of Two Scopes 🌌
When it comes to JavaScript, variables can have two distinct scopes: global and local.
Global Scope 🌍
Variables declared outside of any function, in what we call the global scope, can be accessed from anywhere within your script. This means they have a global reach (pun intended 😉).
For example, let's say we have the following code snippet:
const planet = "Earth";
function greet() {
console.log("Hello, " + planet + "!");
}
greet(); // Output: Hello, Earth!
In this case, the planet
variable is declared globally, so both the greet
function and any other part of the script can access and use its value.
Local Scope 🔍
On the other hand, variables declared within a function have a local scope, meaning they are only accessible within the confines of that specific function.
function greet() {
const person = "John";
console.log("Hello, " + person + "!");
}
greet(); // Output: Hello, John!
console.log(person); // ReferenceError: person is not defined
In this example, the person
variable has a local scope within the greet
function. Trying to access it from outside the function will result in a ReferenceError
since it's out of scope.
It's All About Context 🧐
When it comes to variables inside and outside functions, context matters. Variables declared inside a function using the var
, let
, or const
keywords are limited to that particular function's scope.
Let's dive a bit deeper into this:
function sayHello() {
var greeting = "Hello!";
if (true) {
var greeting = "Hola!";
console.log(greeting); // Output: Hola!
}
console.log(greeting); // Output: Hola!
}
console.log(greeting); // Output: ReferenceError: greeting is not defined
In this case, the greeting
variable is declared twice, both times using the var
keyword. The beauty (and potential trap) of using var
is that it doesn't respect block-level scopes (like if statements). So, the value of greeting
is updated within the if statement, affecting its value outside the if statement as well.
Where Do Global Variables Live? 🤔
Great question! 🧐 Global variables defined outside of any function in JavaScript are stored in a special object called the global object.
In a browser environment, the global object is window
, meaning that global variables become properties of the window object. For example:
var fullName = "John Doe";
console.log(window.fullName); // Output: John Doe
By accessing window.fullName
, we can retrieve the value of fullName
, which was declared globally.
Wrapping It All Up 🎁
Understanding variable scopes is crucial for writing clean and maintainable JavaScript code. Remember:
Global variables have a global scope and can be accessed anywhere in your script.
Local variables exist within a specific function's scope and are inaccessible outside of it.
Be mindful of the context when declaring variables; using
var
can lead to unexpected behavior.Global variables reside within the global object,
window
in browser environments.
So, whether you're running JavaScript in a browser or a server, scoping is a fundamental concept that fuels the language's flexibility and power.
That wraps it up, folks! We hope this post shed some light on the mysterious world of variable scopes in JavaScript. 🌟
If you found this post helpful, be sure to share it with your fellow developers! And don't forget to leave a comment below with your thoughts and any other topics you'd like us to cover.
Until next time, happy coding! 💻🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
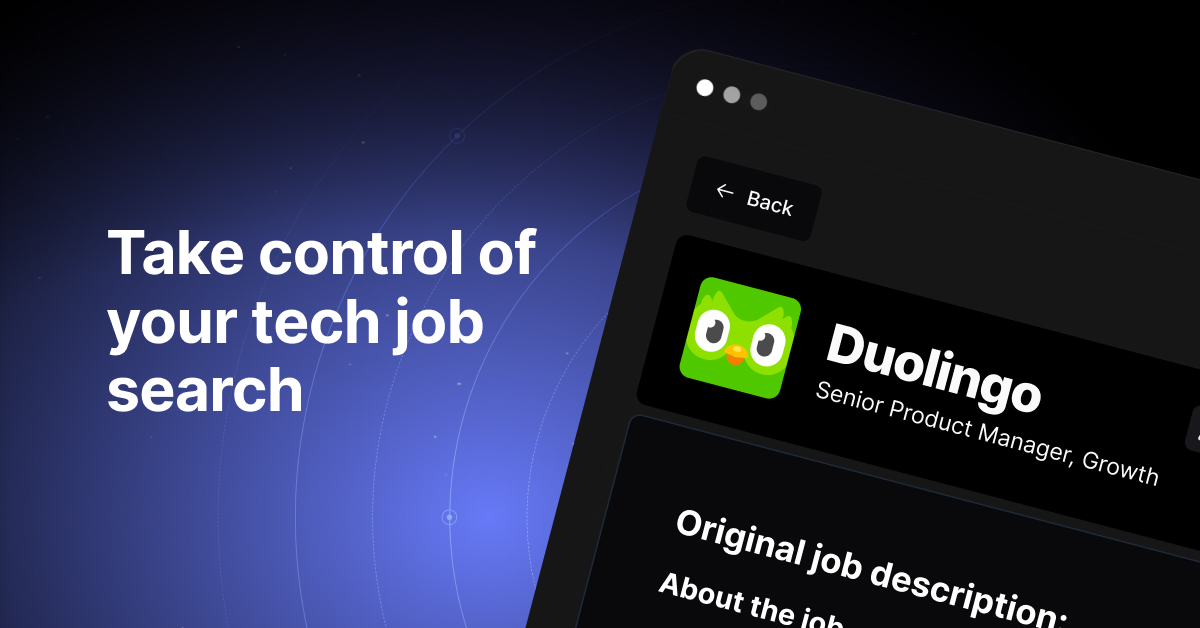