What is the purpose of the var keyword and when should I use it (or omit it)?
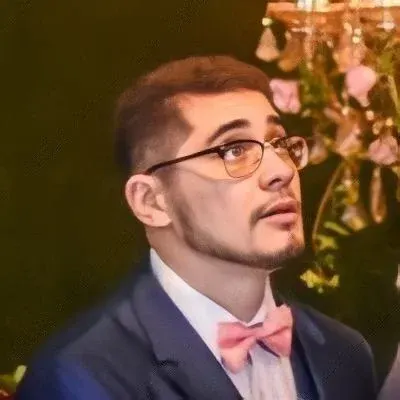
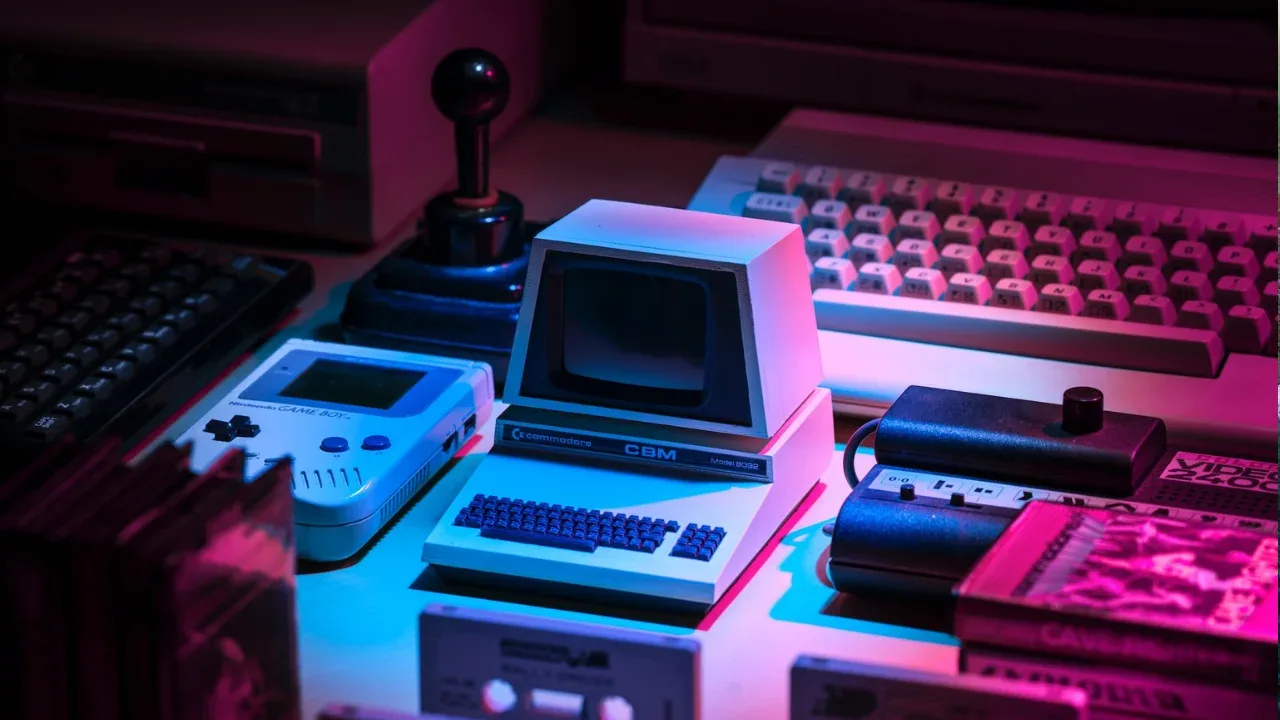
š The Purpose of the var
Keyword in JavaScript š
š Hey there, JavaScript enthusiasts! šāØ Have you ever wondered what the purpose of the var
keyword is in JavaScript? š¤ Today, we'll dive deep into this topic to answer all of your burning questions. So grab your favorite šæ snack and let's get started!
šµļøāāļø The Function of the var
Keyword
š® The var
keyword is used in JavaScript to declare variables. š It is mainly used in ECMAScript 3 and 5 versions of JavaScript. However, please note that the answers provided here might become outdated with the introduction of new features in ECMAScript 6 and later releases.
š When you declare a variable using the var
keyword, it is function-scoped. This means that the variable's scope is limited to the function in which it is declared. If a variable is declared within a function, it is not accessible outside of that function. On the other hand, if a variable is declared outside of any function, it becomes a global variable and can be accessed from anywhere within the program.
š Here's an example to illustrate how the var
keyword works:
function exampleFunction() {
var localVar = 'I am a local variable';
globalVar = 'I am a global variable';
}
exampleFunction();
console.log(localVar); // Throws an error because localVar is not accessible here
console.log(globalVar); // Prints 'I am a global variable' because globalVar is accessible globally
In this example, localVar
is declared using the var
keyword, and it can only be accessed within the exampleFunction
. globalVar
, on the other hand, is not declared using the var
keyword, making it a global variable. This means it can be accessed from anywhere within the program, even outside of the exampleFunction
.
š¤·āāļø When to Use the var
Keyword (or Omit it)
š¤ Now that we know the function of the var
keyword, let's address the question of when to use it and when to omit it.
š It is generally recommended to avoid using the var
keyword in modern JavaScript code. Instead, use the const
and let
keywords, which were introduced in ECMAScript 6. These keywords provide block-scoping, which is more predictable and less error-prone than function-scoping.
š However, if you are working with legacy code or need to maintain compatibility with ECMAScript 3 or 5, using the var
keyword is still necessary.
š Here's a simple guide to help you decide when to use the var
keyword or omit it:
Use
var
when:Maintaining compatibility with ECMAScript 3 or 5
Working with legacy code that relies on function-scoping
Use
const
orlet
when:Writing modern JavaScript code
Embracing block-scoping for more predictable and readable code
š Wrap Up and Take Action!
š Congratulations, you're now equipped with the knowledge of what the var
keyword does and when to use it (or omit it) in JavaScript! š
š Remember, it's generally recommended to use const
or let
instead of var
in modern JavaScript code for better scoping.
š We hope you found this guide helpful and insightful. If you have any further questions or topics you'd like us to cover, feel free to leave a comment below. Let's keep learning and growing together! š±šŖ
š Don't forget to share this post with your JavaScript-loving friends and colleagues. Sharing is caring, and together we can make the coding world a better place! šā¤ļø
See you in the next post! Happy coding! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
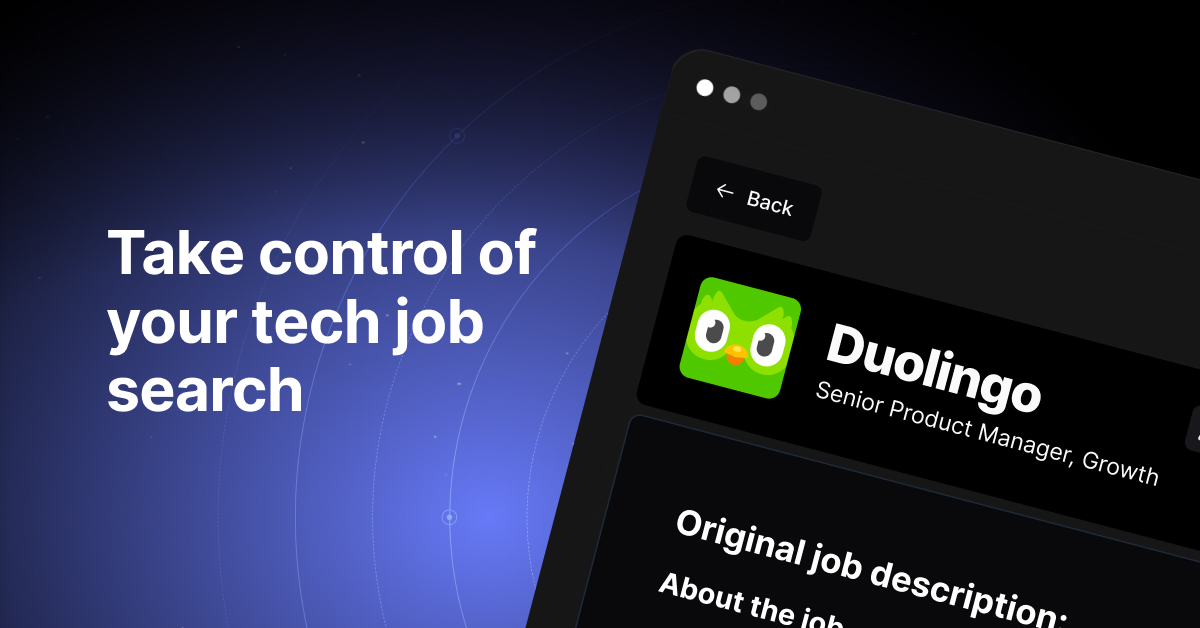