What is the purpose of Node.js module.exports and how do you use it?
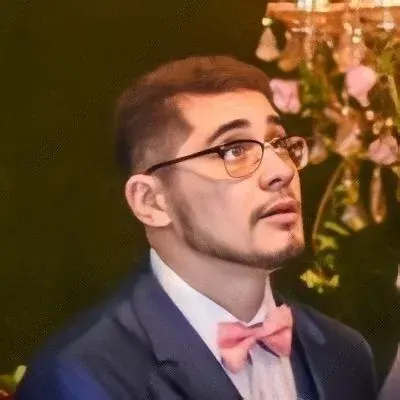
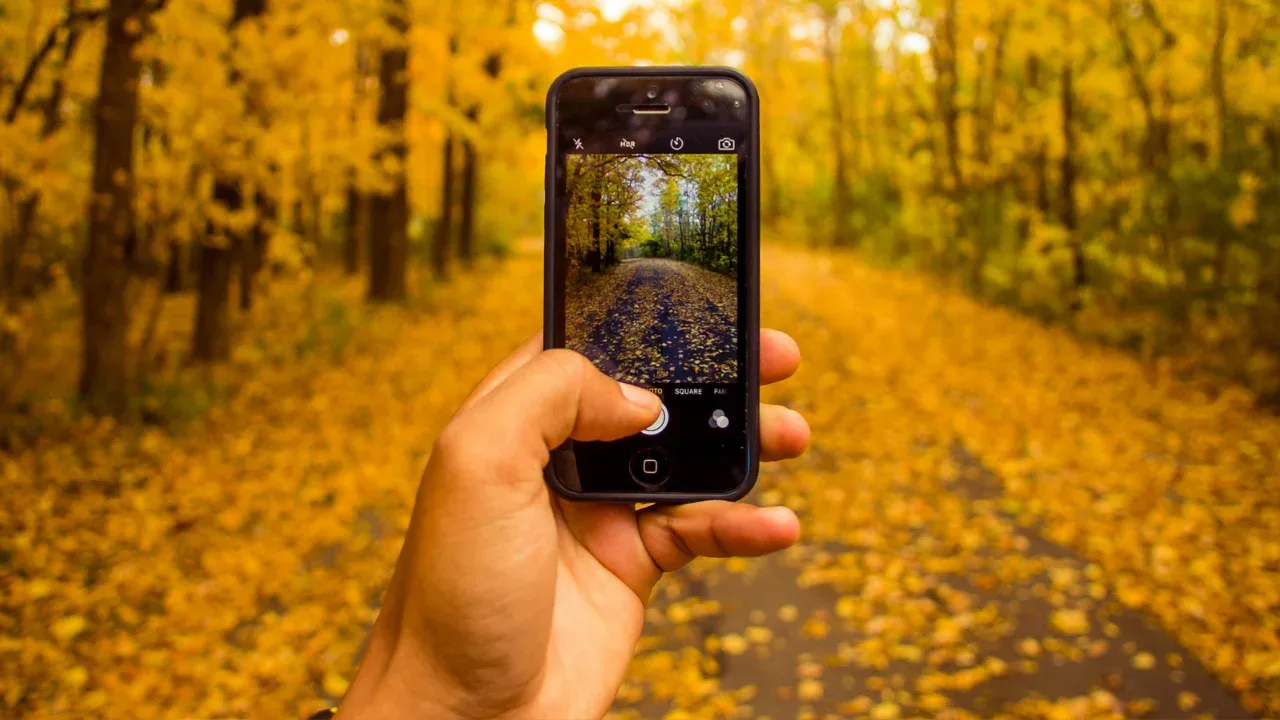
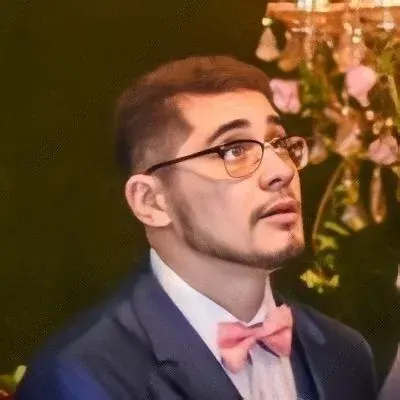
📣 Hey there tech enthusiasts! 😄 Are you baffled by the purpose of Node.js module.exports
? Wonder no more, because in this blog post, we'll dive into its functionality and show you how to use it like a pro! 💪 So, let's get started! 🚀
Understanding the Purpose of module.exports
In Node.js, the module.exports
object is an essential part of the CommonJS module system. It allows you to define what objects, functions, or values should be exported from a module and made available to other modules that require it. Think of it as a packaging system to share code between different files in your application. 📦🔗
How to Use module.exports
To utilize the power of module.exports
, follow these easy steps:
Defining Exports: Inside your module file, assign the object, function, or value you want to export to
module.exports
. For example, let's say we have a file calledcalculator.js
, and we want to export a function namedadd
:// calculator.js const add = (a, b) => a + b; module.exports = add;
In this case, we assign the
add
function tomodule.exports
, thereby making it accessible to other modules.Requiring the Module: In another file where you want to use the exported functionality, use the
require
function to import the module. This allows you to access the exported object, function, or value. For our example above, we can use theadd
function fromcalculator.js
like this:// main.js const add = require('./calculator'); console.log(add(2, 3)); // Output: 5
By calling
require('./calculator')
, we can access theadd
function and use it as if it was defined in the current module.
And that's it! With just these two steps, you can easily export and import the desired functionality using module.exports
. 🎉
Common Issues and Easy Solutions
🔍 Let's address some common issues and provide you with easy solutions:
Issue: "I want to export multiple functions or values." To export multiple functions or values, simply attach them as properties to the
module.exports
object. For example:// myModule.js function greet() { console.log('Hello!'); } function farewell() { console.log('Goodbye!'); } module.exports = { greet, farewell, };
Then, you can require and use these functions in another module.
Issue: "I want to rename the imported module." If you want to rename the imported module, you can assign it to a variable with a different name during the
require
statement. For example:// main.js const myCalculator = require('./calculator'); console.log(myCalculator(2, 3)); // Output: 5
Here, we rename the
add
function fromcalculator.js
tomyCalculator
for convenience.
Your Turn to Shine! ✨
Now that you have a good understanding of module.exports
, it's time to apply it in your own projects! Experiment with different types of exports and try importing them into other files. Don't forget to share your experiences and any cool use cases you come across in the comments below! Let's learn from each other and create awesome code together! 🤝🌟
Keep coding, stay curious, and until next time! Happy creating! 😃👩💻👨💻
Don't miss out on our latest tech tips and tricks! Join our newsletter for regular updates at www.awesomeblog.com/newsletter.