What is the non-jQuery equivalent of "$(document).ready()"?
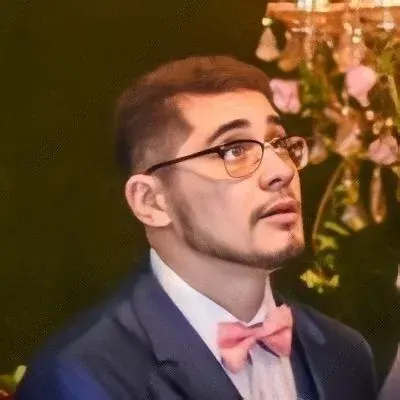
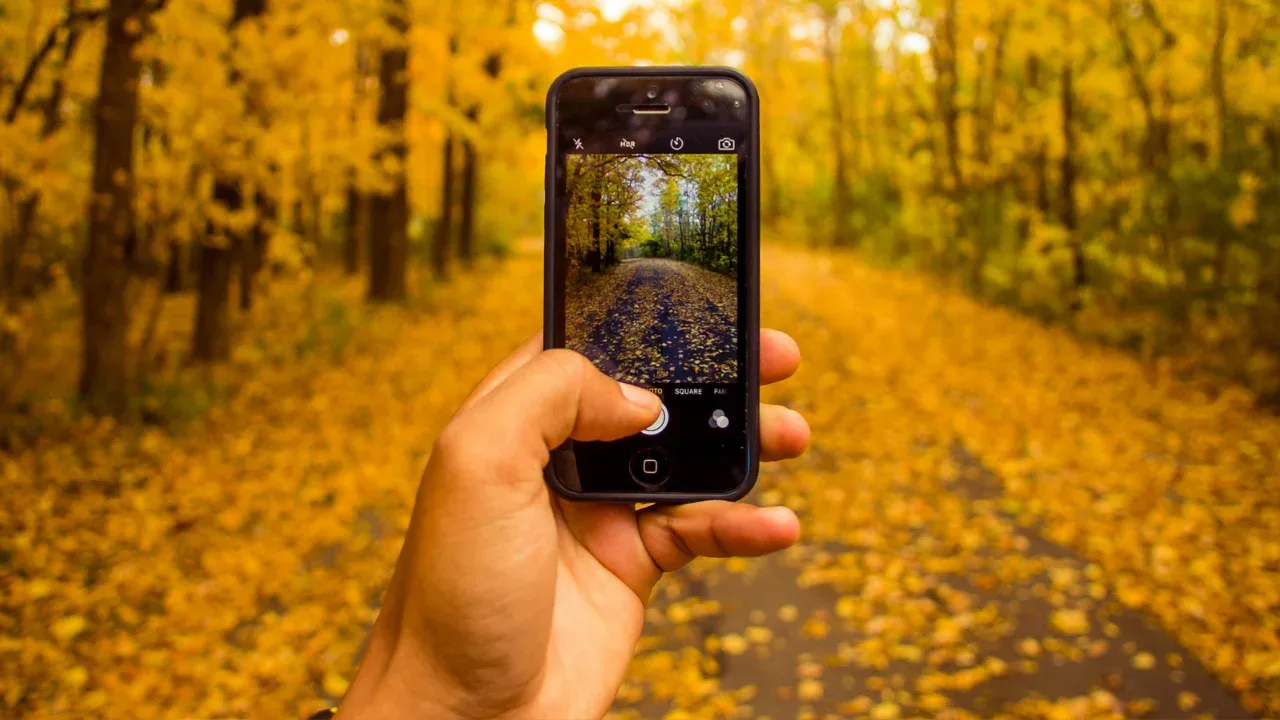
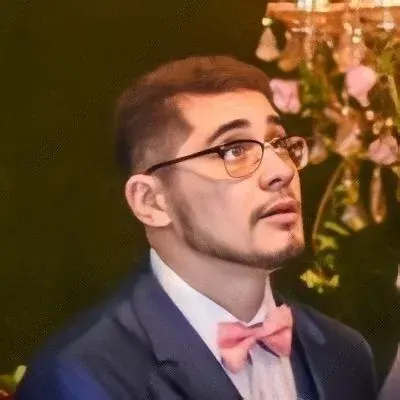
What's the 🚀 Non-jQuery Equivalent of $(document).ready()
?
So, you're dabbling in JavaScript and you're curious about the non-jQuery alternative to $(document).ready()
. Good for you! Let's dive right into it and uncover the mysteries of this JavaScript initialization function!
The $(document).ready()
Function in jQuery 🕺
Before we proceed, let's quickly recap what $(document).ready()
does. In the jQuery library, this function is used to execute code when the DOM (Document Object Model) has finished loading. Basically, it ensures that your JavaScript code runs only when the HTML document is fully loaded, avoiding any potential issues.
$(document).ready(function() {
// Your code here
});
The Non-jQuery 🌠 Way to Go
Now let's talk about how we can achieve the same effect without relying on jQuery. Here's the non-jQuery equivalent of $(document).ready()
:
document.addEventListener("DOMContentLoaded", function() {
// Your code here
});
Ta-da! 🎉 With the DOMContentLoaded
event, you can achieve the same magic as $(document).ready()
. This event fires when the HTML document has finished loading and parsing, enabling you to execute your JavaScript without any worries.
Common Pitfalls and Easy Solutions 👷♀️
Sometimes, you might encounter some issues while trying to use $(document).ready()
or DOMContentLoaded
. Here are a couple of common pitfalls and their easy solutions:
1. Placing JavaScript Code Before the DOM Element
Problem: You place your JavaScript code before the DOM element that it is manipulating, resulting in errors or undesired behavior.
Solution: Ensure that your JavaScript code is placed after the DOM element it is targeting. Move your script tag to a more appropriate position, either at the bottom of the HTML body or inside the <head>
section.
2. Multiple $(document).ready()
or DOMContentLoaded
Events
Problem: You have multiple instances of $(document).ready()
or DOMContentLoaded
events, causing conflicts or executing code unnecessarily.
Solution: To avoid conflicts and improve performance, consolidate all your code within a single $(document).ready()
or DOMContentLoaded
event.
Time to Experiment! 💡
Now that you know the non-jQuery equivalent of $(document).ready()
, it's time to put your newfound knowledge to the test! Try replacing your jQuery code with vanilla JavaScript using DOMContentLoaded
and observe the results.
Feeling confident? Great! Start optimizing your code and leave behind the jQuery dependency.
Don't forget to share your results and experiences in the comments section below! Let's celebrate our JavaScript victories together! 🙌
Wrapping Up 🎁
By now, you should be a pro at using the non-jQuery alternative to $(document).ready()
. Remember, the DOMContentLoaded
event is your new best friend when it comes to executing JavaScript code after the HTML document has finished loading.
Experiment with this newfound knowledge, tackle common pitfalls, and don't shy away from leaving your questions or comments below. Let's keep the JavaScript conversation going! 💬
Happy coding! 💻