What is the most efficient way to deep clone an object in JavaScript?
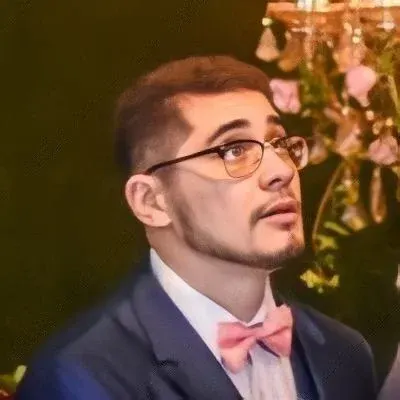

💡 The Quickest Way to Deep Clone an Object in JavaScript
Do you want to clone a JavaScript object without any fuss and hassle? Look no further! In this guide, we will address the common issues surrounding deep cloning objects in JavaScript and provide you with easy and efficient solutions. 🔥
The Problem: Inefficient Cloning Methods
You've probably come across several cloning methods that seem promising at first but ultimately fall short. Let's take a look at a few popular ones:
eval(uneval(o))
: While this method may work in Firefox, it is non-standard and lacks support in other browsers. Using non-standard features can lead to compatibility issues and headaches down the road. 😵JSON.parse(JSON.stringify(o))
: This approach seems simple, but it has its downsides. It works well for plain objects and arrays, but it falls flat when dealing with complex objects that include functions,Date
objects, and other non-serializable values. Plus, it can be slower for large objects. 🐌Recursive copying functions: Creating your own recursive function might seem like the best solution, but it can be error-prone and inefficient. Depending on the implementation, you may encounter issues with circular references, prototype chain preservation, or the omission of certain properties. 😓
The Solution: A Fast and Reliable Deep Cloning Method
Fear not, for we have a reliable solution that combines efficiency, compatibility, and simplicity. 🚀
Behold the power of the Lodash library! Lodash provides a cloneDeep
function that performs deep cloning in a safe and efficient manner. First, make sure to include the Lodash library in your project:
<script src="https://cdn.jsdelivr.net/npm/lodash@4.17.21/lodash.min.js"></script>
Now, let's see cloneDeep
in action:
const clonedObject = _.cloneDeep(originalObject);
That's it! With just one line of code, you can now confidently deep clone any complex JavaScript object. Lodash takes care of all the edge cases, preserving circular references, prototypes, and non-serializable values seamlessly. 😎
The Call to Action: Share Your Cloning Stories!
Now that you know how to efficiently deep clone objects in JavaScript, it's time to put your newfound knowledge to the test. Try it out in your own projects and see the magic unfold! ✨
We would love to hear about your experiences with cloning objects in JavaScript. Have you faced any challenges or found alternative solutions? Share your thoughts, tips, and tricks in the comments below. Let's clone objects like a pro and help each other strive for efficient and reliable code! 💪🤓
Remember, sharing is caring. If you found this guide helpful, don't forget to share it with your fellow developers and spread the knowledge! 🌟
Happy cloning! 😄🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
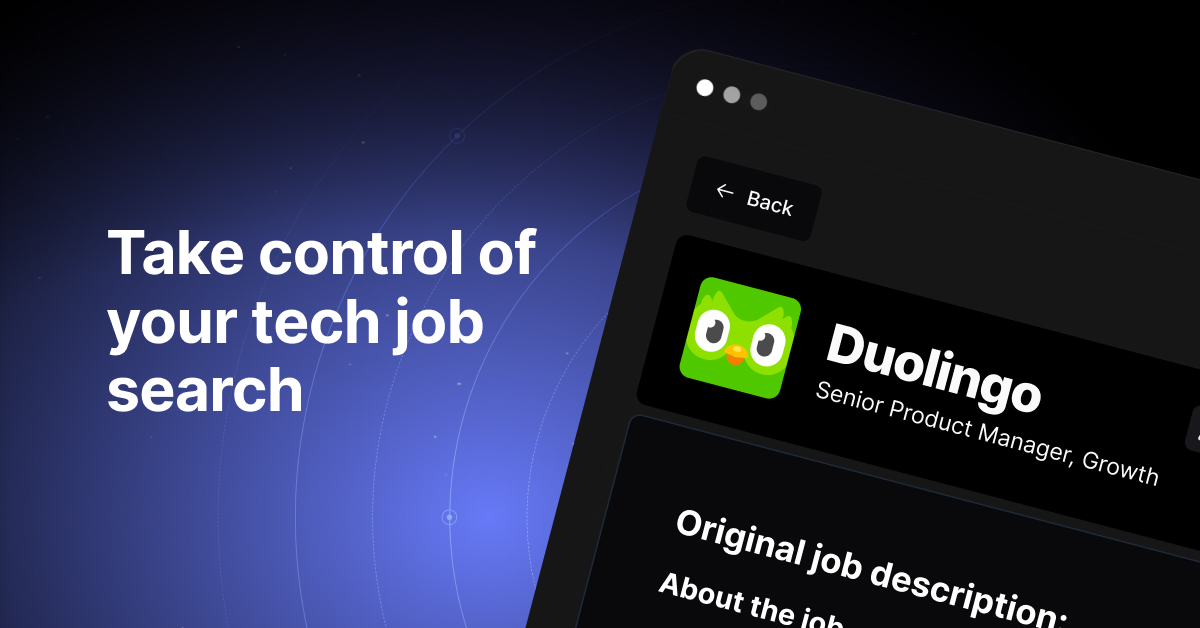