What is the max size of localStorage values?
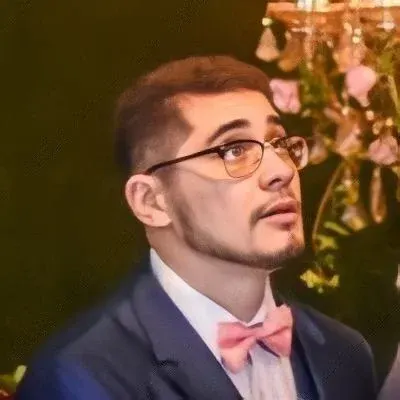
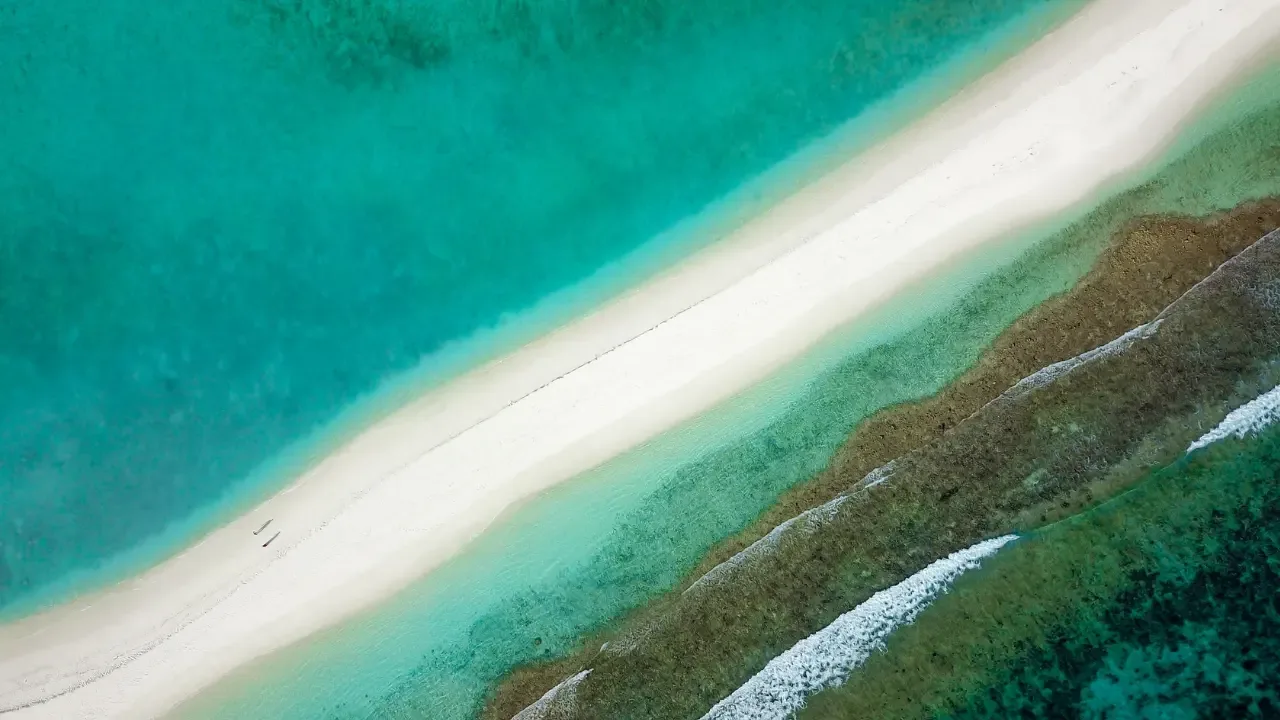
The Ultimate Guide to the Max Size of LocalStorage Values π
<p>Have you ever wondered about the maximum size of <code>localStorage</code> values? π€ It's a common question among developers, and in this guide, we'll delve into this topic and provide you with easy solutions. So grab your coding hats and let's get started! π§’π»</p>
Understanding the Issue π€π‘
<p>First things first, let's understand the problem. ππ</p>
<p>As you may know, <code>localStorage</code> only supports strings as values. This means that any objects or data you want to store need to be converted to strings before storing them. The most common way to do this is by stringifying the objects and storing them as JSON-strings. ππ’</p>
<p>But here's the kicker: is there a defined limitation to the length of these values? Does it vary between browsers? ππ€·ββοΈ</p>
The Big Question: Maximum Size Limit? βπ
<p>It's time to tackle the big question! ποΈββοΈ</p>
<p>Unfortunately, there isn't a straightforward answer that applies to all browsers. The size limit of <code>localStorage</code> values can vary based on the browser and device you are using. β οΈπ»</p>
<p>However, there are general guidelines that can help you work around this issue. Let's explore these solutions! ππ§</p>
Solution 1: Check the Length Attribute ππ
<p>One way to determine the size of a <code>localStorage</code> value is by checking its length attribute. This attribute returns the number of characters in the string, which gives you a rough estimate of the size. ππ€</p>
const value = localStorage.getItem('myKey');
const size = value.length;
<p>By using this method, you can compare the length against the known limits of different browsers and devices to stay within the safe range. ππ</p>
Solution 2: Chunking Your Data ππ
<p>If you are dealing with large data sets that exceed the size limit, then chunking your data is a great solution. π‘πͺ</p>
<p>Chunking involves splitting your data into smaller pieces and storing them as separate <code>localStorage</code> values. You can use unique keys for each chunk and recombine them when needed. This way, you can bypass the size limitation. π§©βπ</p>
const data = /* large data */;
const chunkSize = /* your desired chunk size */;
for(let i = 0; i < data.length; i += chunkSize) {
const chunk = data.slice(i, i + chunkSize);
localStorage.setItem(`chunk_${i}`, chunk);
}
<p>Just remember to define a strategy to handle the recombination of the chunks when you need to retrieve the data. ππ</p>
Solution 3: Using Web SQL or IndexedDB ππ§
<p>If you find that the size limit of <code>localStorage</code> is too restrictive for your needs, you can consider using Web SQL or IndexedDB. These are databases that offer more storage capacity and advanced features. πͺπ</p>
<p>While the implementation may be more complex, these alternatives provide a more scalable solution for storing large amounts of data. πΎπ</p>
It's Your Turn to Code! ππ©βπ»
<p>Now that you have learned about different solutions, it's your turn to put them into action! Try experimenting with the methods we discussed and see what works best for your specific use case. π‘π§ͺ</p>
<p>Remember, understanding the limitations and adapting to them is a crucial skill for every developer. So don't give up! Keep exploring, learning, and coding. ππ»</p>
Join the Discussion! π¬π€
<p>We hope this guide helped you navigate the complex world of <code>localStorage</code> size limitations. If you have any thoughts, tips, or additional questions, we would love to hear from you! Let's build a vibrant community by sharing our knowledge. ππ</p>
<p>Leave a comment below and join the conversation! Happy coding! ππ»</p>
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
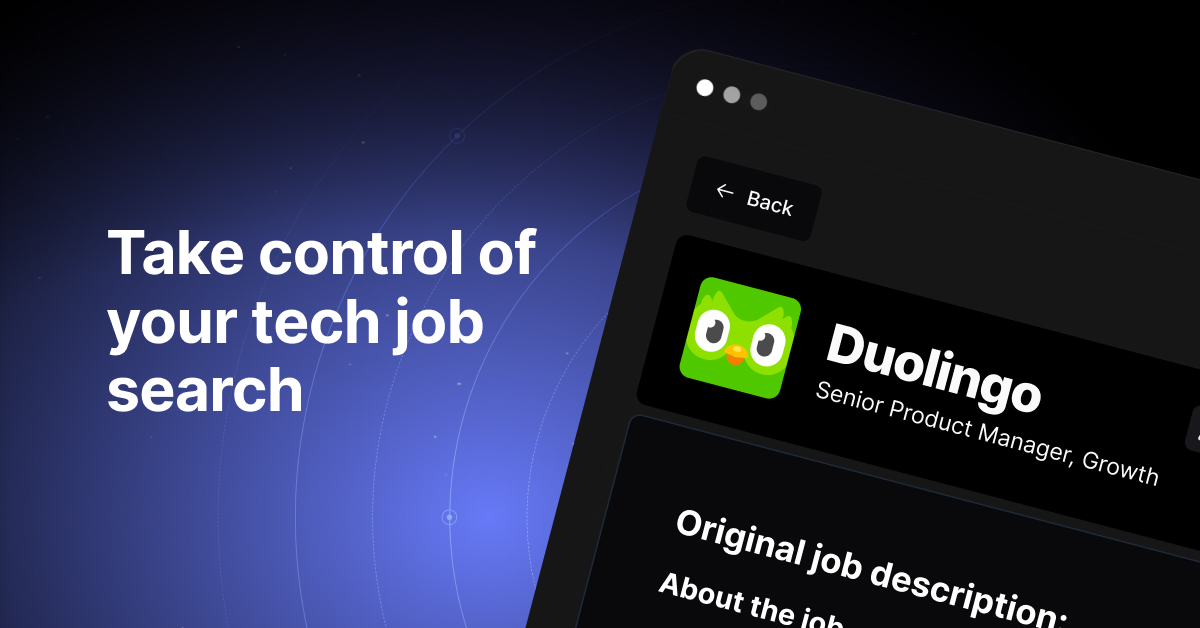