What is the JavaScript version of sleep()?
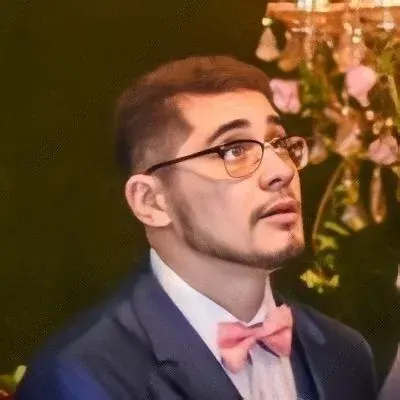
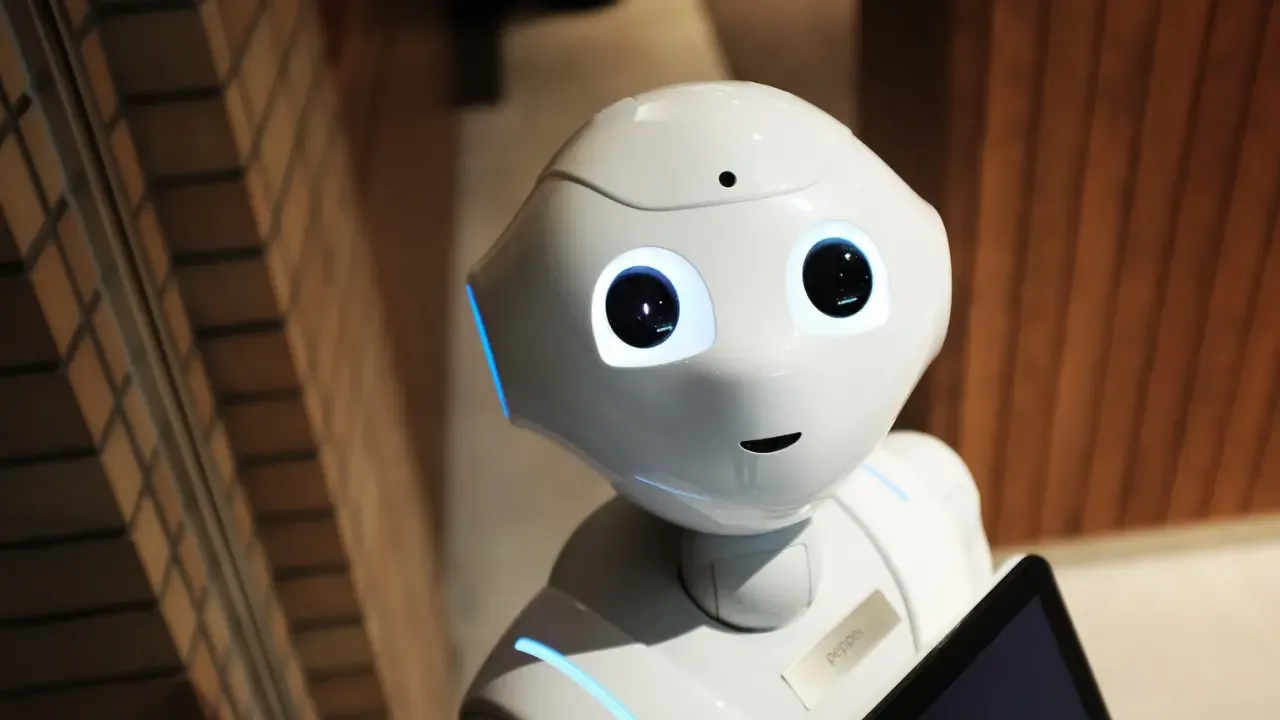
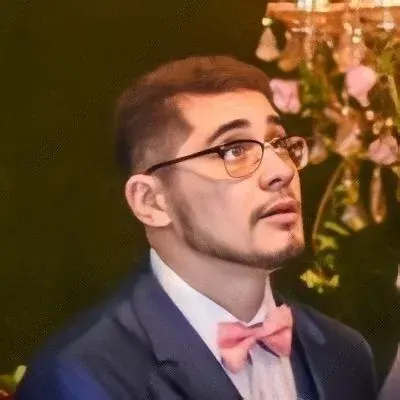
💤 Mastering the Art of JavaScript Sleep 💤
Have you ever wished for a magical ✨ sleep function ✨ in JavaScript that could pause the execution of your code for a certain amount of time? Maybe you wanted to create a suspenseful effect or simulate a delay between actions. Fear not, my fellow JavaScript enthusiast! I am here to unveil the secrets of JavaScript sleep 🌙.
The "pausecomp" Dilemma 😴
Let's start by addressing the existing solution 🤔. The mentioned pausecomp
function may work, but deep down, we know it's not the right way to go. It relies on using a do-while loop that continuously checks the current date until the desired pause time has elapsed. This approach not only consumes unnecessary resources but also blocks the main thread, preventing other operations from being executed.
⏰ Introducing the Awaited Sleep in JavaScript ⏰
To achieve a proper sleep functionality in JavaScript, we need to leverage a powerful concept called Promises. Promises allow us to handle asynchronous tasks in a more elegant and organized way.
Here's the most common and recommended method to create a JavaScript sleep function using Promises:
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
Looks pretty neat, right? With this sleep
function, we can now pause the execution of our code for a specified duration 🎉. Let's see it in action:
console.log('Before sleep');
await sleep(2000);
console.log('After sleep');
In this example, the console will display "Before sleep," pause the execution for 2 seconds (2000 milliseconds), and then print "After sleep". Voilà! It's as simple as that.
🐢 But Wait! "await" Can Only Be Used in Async Functions 🐢
You might have noticed the await
keyword in our example code, which requires an async function to work. If you're not already familiar with async functions, they allow us to write asynchronous code in a synchronous-like manner. Thankfully, it's effortless to create an async function and call it to enjoy the beauty of JavaScript sleep.
async function myFunction() {
console.log('Before sleep');
await sleep(2000);
console.log('After sleep');
}
myFunction();
Now, when we invoke myFunction()
, it will execute the code sequentially, just like we're used to, with the added benefit of sleep between the console logs.
💪 Level Up Your JavaScript Skills with Custom Improvements 💪
What if we want to enhance our sleep function even further? Maybe we want to add some extra functionality, like displaying a message during the sleep duration.
Here's an upgraded version of our sleep function, now with an optional message parameter:
function sleep(ms, message = '') {
return new Promise(resolve => {
if (message) console.log(message);
setTimeout(resolve, ms);
});
}
Now, we can provide an additional message to be logged, making our sleep experience more personalized:
await sleep(1000, 'Please hold on tight, processing your request...');
🌟 Join the JavaScript Sleep Revolution! 🌟
Congratulations, dear reader, for reaching this far! You are now equipped with the knowledge to gracefully pause the execution of your JavaScript code. Start implementing this newfound power in your projects and let your creativity soar! 💫
Feel free to explore more about the fascinating world of Promises and async functions. They will undoubtedly level up your JavaScript skills and unlock a plethora of possibilities.
Now go ahead and use JavaScript sleep responsibly. And remember to share this post with other sleep-deprived developers who could use a JavaScript power nap. Sharing is caring after all! ❤️
What other clever use cases have you encountered for the JavaScript sleep function? Share your thoughts in the comments below!