What is the $$hashKey added to my JSON.stringify result
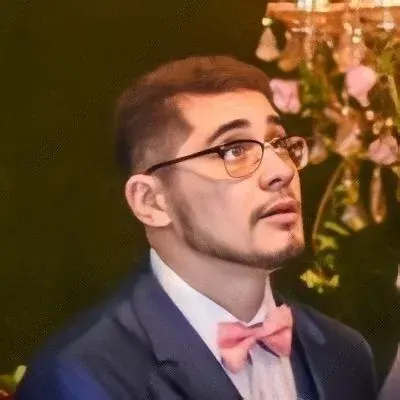
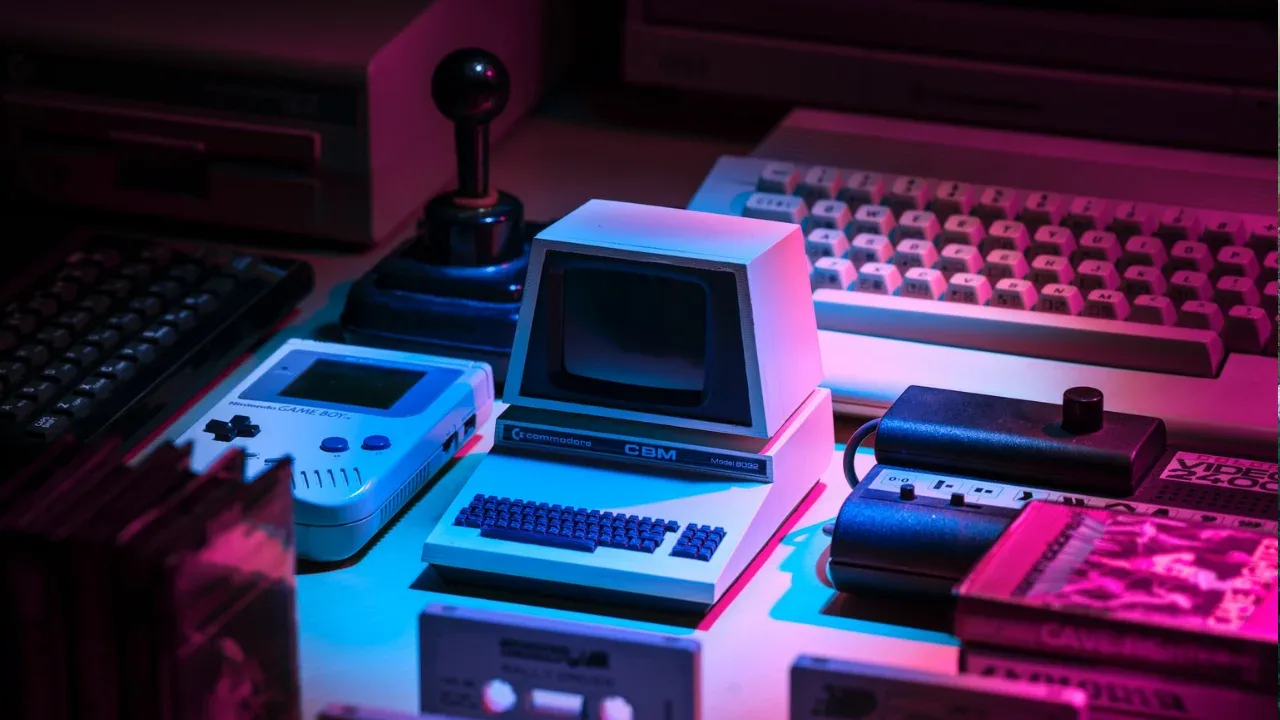
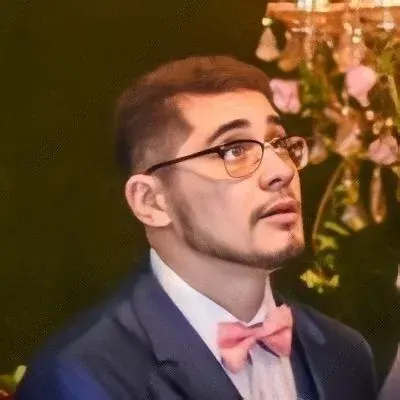
Why is the $$hashKey
added to my JSON.stringify
result? ๐
Are you puzzled by the mysterious appearance of $$hashKey
in your JSON.stringify
result? ๐ค Don't worry, you're not alone! Many developers encounter this unexpected property when serializing their JavaScript objects.
In this blog post, we'll shed some light on what $$hashKey
is and why it appears in your JSON.stringify
result. We'll also provide easy solutions to handle this issue and ensure a clean serialization. So, let's dive in! ๐ช
Understanding the $$hashKey
property ๐ง
The $$hashKey
property is not a standard part of JavaScript. It is actually a special property added by AngularJS, an immensely popular JavaScript framework. AngularJS uses $$hashKey
internally for tracking objects in its two-way data binding mechanism.
When you have an array of objects in AngularJS, each object is assigned a unique $$hashKey
property. This property helps AngularJS identify and track changes to individual objects efficiently. However, it is important to note that the $$hashKey
property is specific to AngularJS and has no meaning outside the framework.
Why does $$hashKey
appear in your JSON.stringify
result? ๐คทโโ๏ธ
The reason you see $$hashKey
in your JSON.stringify
result is that, by default, JSON.stringify
serializes all enumerable properties of an object, including those that are specific to AngularJS, such as $$hashKey
.
When you stringify an array of objects that have $$hashKey
properties using JSON.stringify
, these properties will be included in the resulting JSON string. This behavior is expected because JSON.stringify
doesn't have built-in knowledge about AngularJS-specific properties.
Easy solutions to remove $$hashKey
during serialization ๐
If you want to exclude the $$hashKey
property from your JSON.stringify
result, there are a few simple and straightforward solutions:
Solution 1: Manually remove $$hashKey
before serializing ๐งน
One way to handle this is by manually removing the $$hashKey
property from each object before calling JSON.stringify
. Here's an example:
const objects = [
{
"param_2": "Description 1",
"param_0": "Name 1",
"param_1": "VERSION 1",
"$$hashKey": "005"
},
// other objects...
];
const cleanObjects = objects.map(obj => {
const { $$hashKey, ...cleanObj } = obj;
return cleanObj;
});
const jsonString = JSON.stringify(cleanObjects);
console.log(jsonString);
In this solution, we use the map
function to iterate over each object in the array and create a new object without the $$hashKey
property. The resulting array of clean objects is then serialized using JSON.stringify
.
Solution 2: Utilize a reusable function to remove $$hashKey
๐งน
If you have multiple places in your codebase that require removing $$hashKey
properties, it can be helpful to create a reusable function. Here's an example of a generic function that removes $$hashKey
:
function removeHashKeys(objects) {
return objects.map(obj => {
const { $$hashKey, ...cleanObj } = obj;
return cleanObj;
});
}
// Usage:
const cleanObjects = removeHashKeys(objects);
const jsonString = JSON.stringify(cleanObjects);
console.log(jsonString);
By encapsulating the logic in a function, you can easily reuse it whenever necessary, making your code more modular and maintainable.
Call-to-action: Engage with the community! ๐
Now that you understand why $$hashKey
appears in your JSON.stringify
result and how to remove it, we encourage you to share your experience or ask any further questions in the comments below. Have you encountered any other unexpected behavior during object serialization? Let us know!
By engaging with the community, you not only enhance your own knowledge but also help fellow developers overcome similar challenges. Together, we can make the coding world a better place! ๐๐ป
So go ahead, share your thoughts, and let's start a fruitful conversation! Happy coding! ๐โจ