What is the difference between & vs @ and = in angularJS
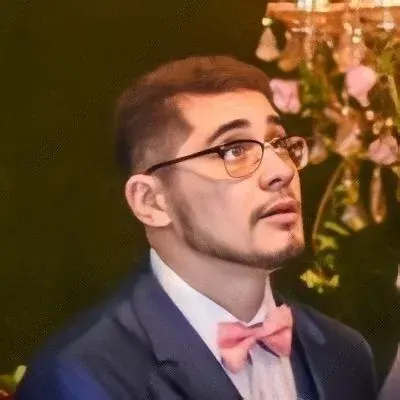
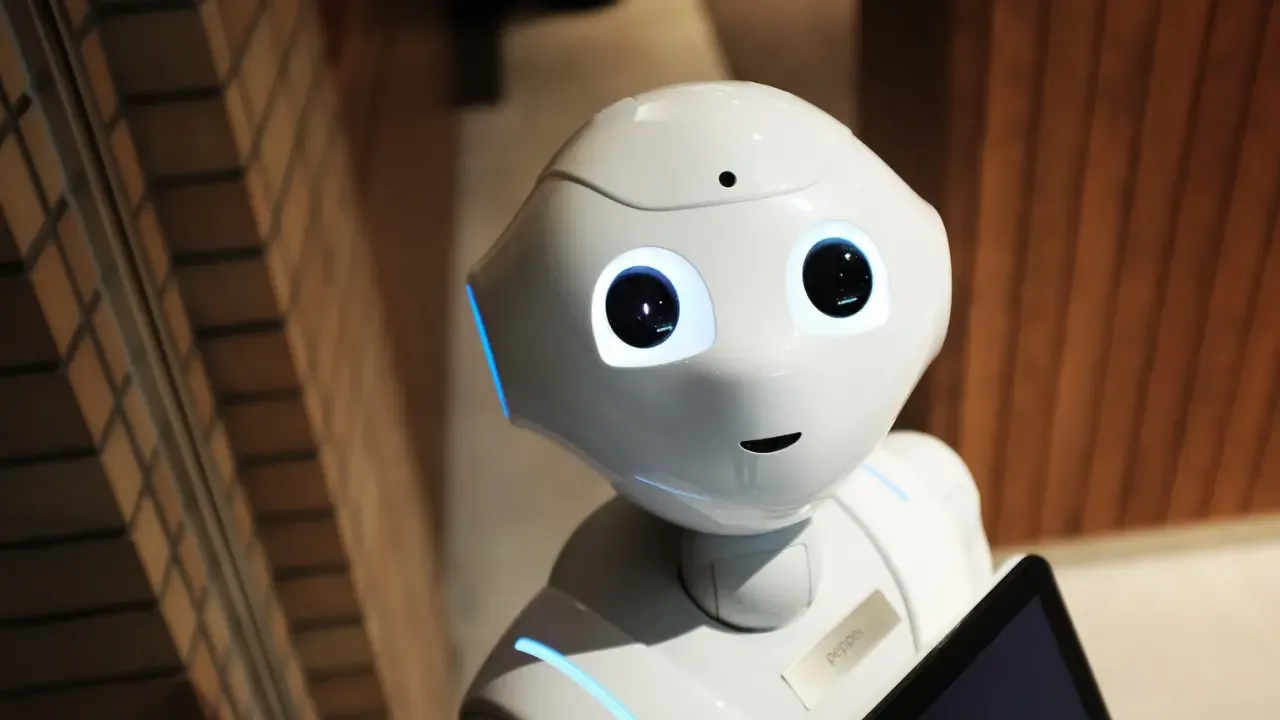
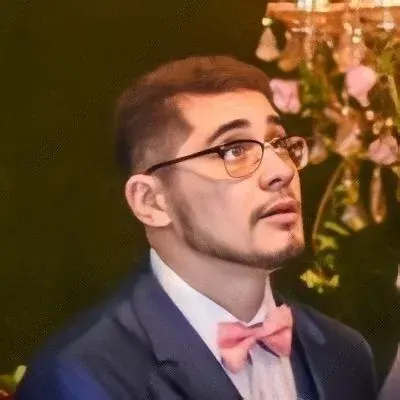
🌟 Unraveling the Mystery: Understanding the Difference Between &, @, and = in AngularJS 🌟
Are you new to AngularJS and feeling like a bewildered explorer lost in a labyrinth of confusing operators? Fear not! Today, we're going to shed some light on the difference between the &, @, and = operators, aiding you in conquering your angular woes!
Breaking Down the Operators
&: The Ampersand Operator
The & operator is used to bind an external function to a directive's scope. It allows us to invoke the external function within the directive, thereby enabling effective communication between the directive and its parent scope. 🤝
🚀 Example:
Let's say we have a directive called myDirective
and we want to pass a function from the parent scope to this directive. We can achieve this by using the & operator as follows:
<div ng-controller="MyController">
<my-directive my-function="doSomething()"></my-directive>
</div>
And in the directive definition, we can use the passed function like this:
app.directive('myDirective', function() {
return {
scope: {
myFunction: '&'
},
link: function(scope) {
// Invoking the passed function
scope.myFunction();
}
};
});
@: The At-Symbol Operator
The @ operator serves as a means of passing a simple value as a string from the parent scope to the directive scope. It makes the binding one-way, ensuring that any changes made in the parent scope do not affect the child scope and vice versa. 💌
🚀 Example:
Imagine we have a directive called myDirective
and we want to pass a string value from the parent scope to the directive. We can utilize the @ operator like this:
<div ng-controller="MyController">
<my-directive my-value="Hello, AngularJS!"></my-directive>
</div>
Within the directive definition, we can access this string value through the attribute:
app.directive('myDirective', function() {
return {
scope: {
myValue: '@'
},
link: function(scope) {
console.log(scope.myValue); // Output: 'Hello, AngularJS!'
}
};
});
=: The Equals Operator
The = operator is used for two-way binding, allowing changes made in the child scope to reflect in the parent scope, and vice versa. It establishes a direct link between the attribute value in the directive and the property in the parent scope. ↔️
🚀 Example:
Suppose we have a directive called myDirective
and we want to bind an object from the parent scope to the directive, so any modifications made to the object in the child scope automatically update the parent scope. We can make use of the = operator in the following way:
<div ng-controller="MyController">
<my-directive my-object="person"></my-directive>
</div>
And in the directive definition, we can access and modify the object directly:
app.directive('myDirective', function() {
return {
scope: {
myObject: '='
},
link: function(scope) {
console.log(scope.myObject.name); // Output: 'John'
scope.myObject.name = 'Jane'; // Modifying the object
console.log(scope.myObject.name); // Output: 'Jane'
}
};
});
🎉 Time to Empower Yourself with AngularJS Knowledge!
Now that we've unravelled the mysteries of the &, @, and = operators in AngularJS, it's time for you to dive in and apply this newfound knowledge in your projects. Remember, the & operator facilitates function communication, the @ operator is for simple string values, and the = operator enables two-way binding.
Feel free to experiment, explore, and create amazing AngularJS applications with confidence! 💪
Got any other AngularJS questions or need even more clarity on this topic? Drop a comment below and let's embark on a phenomenal AngularJS journey together! 🚀