What is the difference between state and props in React?
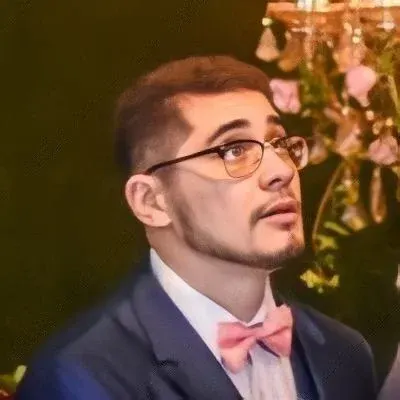

Understanding the Difference between State and Props in React
š Welcome to our tech blog! Today, we will be unraveling the mystery behind the difference between state and props in React. š¤ These concepts can often be confusing, but fear not! We'll break it down into simple terms and provide easy solutions to common issues that arise. Let's dive in! šŖ
What are Props?
Props, short for properties, are a component's configuration or options. They are like the ingredients you add to a recipe ā they provide data to a component from its parent component. š„ Unlike state, props are immutable, meaning they cannot be changed once set.
You might wonder, "Can props change if they are immutable?" Yes! Props can indeed change, but this change occurs at the parent component level. Think of props as being read-only for the current component, ensuring that the child component doesn't accidentally modify its props, preserving data integrity. š
What is State?
On the other hand, state represents the internal data of a component. It is like our emotions ā constantly changing. š©ļø Unlike props, state is mutable, meaning it can be changed within the component.
When a component's state changes, React automatically triggers a re-render, allowing the component to update and reflect the new state. This ability to manage and update state internally makes React components dynamic and interactive. š
When to Use Props and When to Use State?
Now that we understand the basics, let's answer the burning question: When should we use props and when should we use state?
š¹ Use props when you need to pass data from a parent component to its child component(s). This allows you to configure or customize the child component(s) based on the parent's data. Think of props as the communication channel between parent and child components.
š¹ Use state when you need to manage and update data within a component itself. State is perfect for handling user input, managing UI states, and any other data that changes within a component as it gets interacted with.
For example, imagine a "ToDoList" component that receives an array of tasks from its parent component via props. Each task will be rendered as a separate item in the list. Here, using props ensures that the list component always receives the latest tasks from its parent.
However, if we want to add the ability to mark a task as "complete" or "incomplete" within the "ToDoList" component, we would use state. By dynamically updating the state of each task, we can keep track of their completion status and render the visual changes accordingly.
The Power of Correctly Managing Props and State
Now that we've clarified the distinction between props and state, it's crucial to use them correctly to avoid potential issues. š«
šø Remember that props are immutable. Modifying the props directly within a child component is discouraged and can lead to unexpected behavior. If you need to change the data, do it at the parent component level and pass down the updated props.
šø For state, it's essential to follow React's best practices and update it correctly using setState()
. Modifying state directly with this.state
can cause inconsistencies and prevent React from triggering necessary re-renders. Keeping your state updates within setState()
will ensure a smooth and reliable React experience.
š” Pro Tip: If you're working with functional components, you can use React hooks like useState
and useEffect
to manage state and lifecycle.
Wrapping Up
To sum it all up, props and state play different roles in React, helping us build complex and interactive UIs. Props provide parent-to-child communication with immutable data, while state allows components to manage and update their internal data dynamically.
Remember to use props when passing data from a parent to a child component, and use state when managing and updating data within a component itself.
We hope this guide has shed some light on the difference between state and props in React! If you still have questions or need further clarification, drop a comment below, and we'd love to help you out. Happy Reacting! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
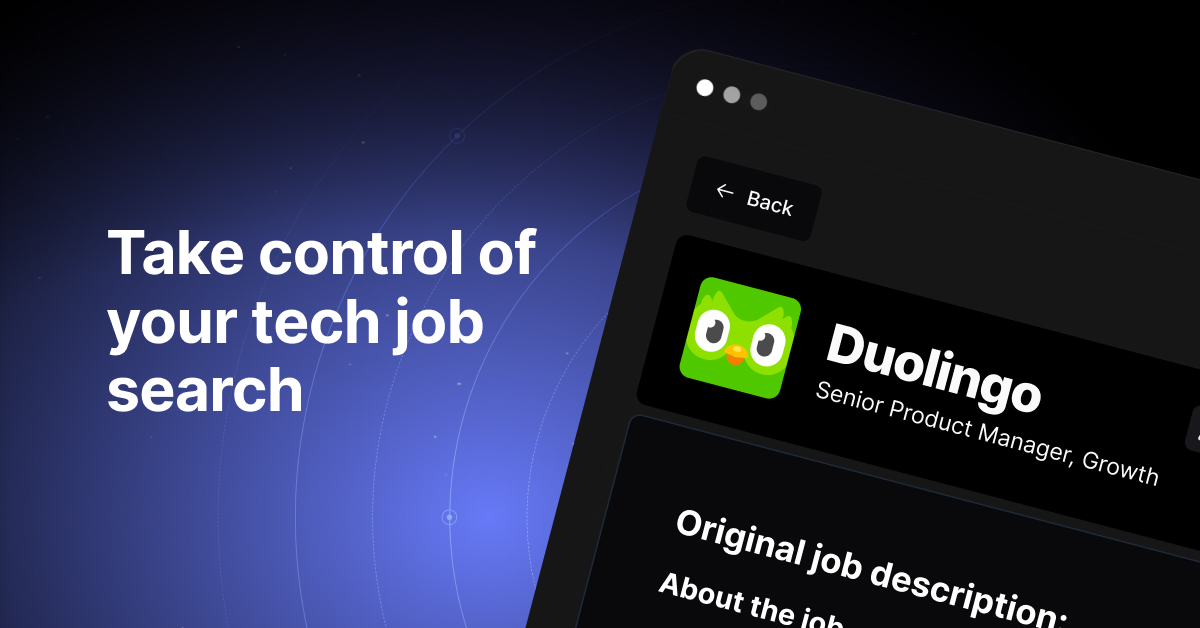