What is the difference between null and undefined in JavaScript?
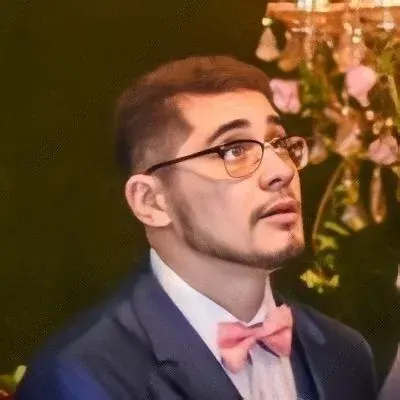
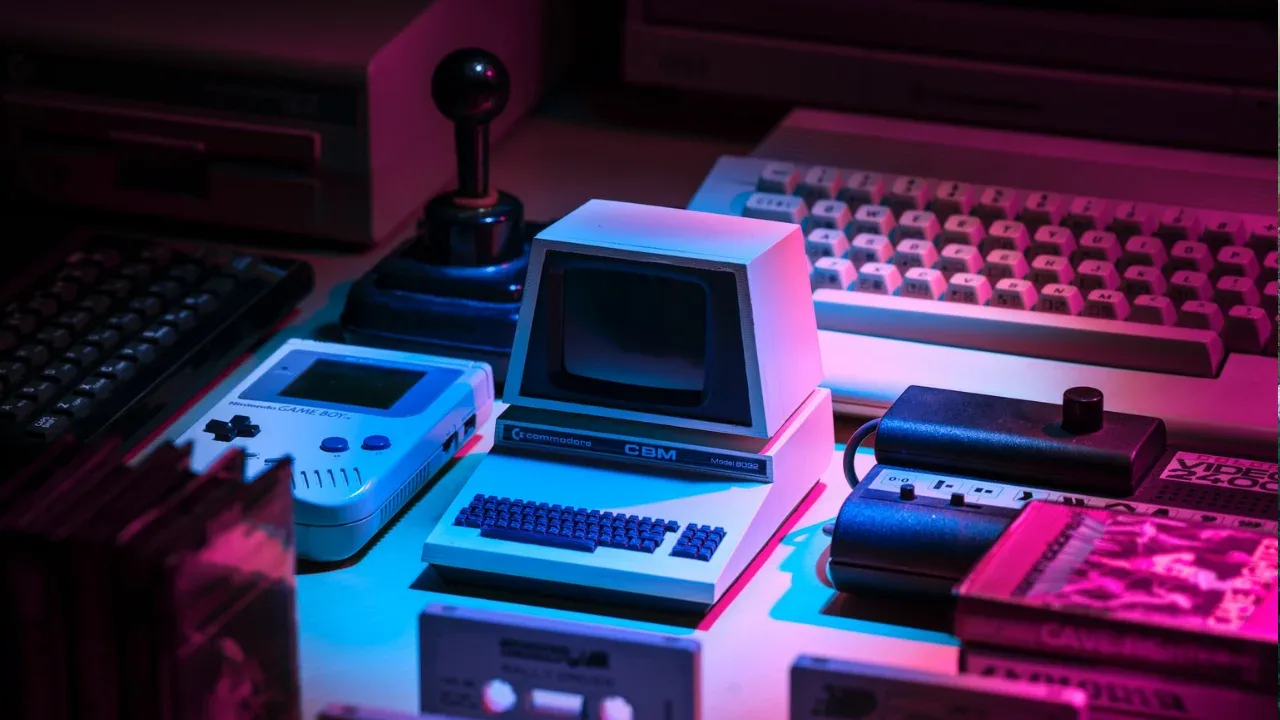
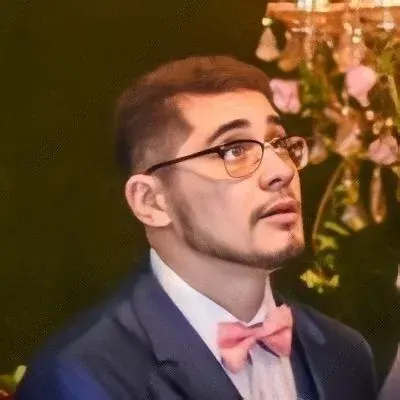
Understanding the Difference between π ββοΈ null and π€·ββοΈundefined in JavaScript
So, you want to know the difference between null
and undefined
in JavaScript? Well, my friend, you've come to the right place! π
These two terms may seem similar, but they have distinct meanings in the JavaScript language. Understanding their differences can make your code cleaner, more robust, and less prone to bugs. Let's dive right in, shall we? πͺ
π ββοΈ null: The Absence of Value
When you assign the value null
to a variable, you are explicitly stating that it has no value or object assigned to it. In other words, it represents the intentional absence of an object or value. So, if you come across a variable with a value of null
, it means that it has been purposely set to nothing.
Here's an example:
let car = null;
console.log(car); // Output: null
In the example above, the variable car
has been assigned the value null
, indicating that there is no car object or value present. Think of it as an empty container waiting to be filled. π
π€·ββοΈundefined: The Unknown or Missing Value
Unlike null
, undefined
represents the default value of a variable that has not been assigned any value yet. It indicates that a variable or object has been declared but has not been initialized with a value. Essentially, it is JavaScript's way of saying, "I don't know what the value is yet."
Take a look at this code snippet:
let username;
console.log(username); // Output: undefined
In this case, the variable username
has been declared but has no assigned value. Hence, it prints undefined
. It's like an empty box whose contents are still a mystery. π¦
π€ Understanding the Difference
Now that we know what null
and undefined
are, let's compare them side by side for clarity:
null
is an intentional absence of an object or value.undefined
is the default value of a variable that has not been assigned a value yet.You can explicitly assign
null
to a variable, whileundefined
is automatically assigned by JavaScript.
π‘ Dealing with Common Issues
Understanding the difference between null
and undefined
can help you avoid common pitfalls and make your code more robust. Here are a few common scenarios and how to handle them:
Checking for presence: When you want to check if a variable has been assigned a value or object, it's common to use
=== undefined
or!== undefined
. This ensures that the variable is not only present but also assigned a value.Assigning default values: You can use the logical OR operator (
||
) to assign a default value to a variable if it isnull
orundefined
. For example:let car = getCar() || getDefaultCar();
In this example,
getCar()
returns either a valid car object ornull
/undefined
. If it returns a falsy value, the||
operator will evaluate to the right-hand side and assign the return value ofgetDefaultCar()
.Avoiding type errors: Be cautious when comparing values that may be either
null
orundefined
. Using the strict equality operator (===
) will only returntrue
if the compared values are of the same type and have the same value. For example:let value = getValue(); if (value !== null && value !== undefined) { // Do something with the value }
By performing these checks, you ensure that the variable has a valid value before proceeding with any operations.
π£ Call to Action: Share Your Thoughts!
Now that you have a clear understanding of the difference between null
and undefined
, it's time to put your knowledge into action! π
I'd love to hear your thoughts on this topic. How has your experience been with handling null
and undefined
in your JavaScript projects? Have you encountered any common issues we haven't covered? Let's discuss in the comments section below! π¬
Remember, solidifying your understanding of these fundamental JavaScript concepts will empower you to write cleaner, more reliable code. Happy coding! π