What is the difference between call and apply?
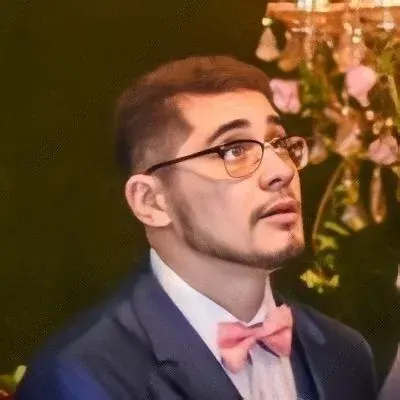
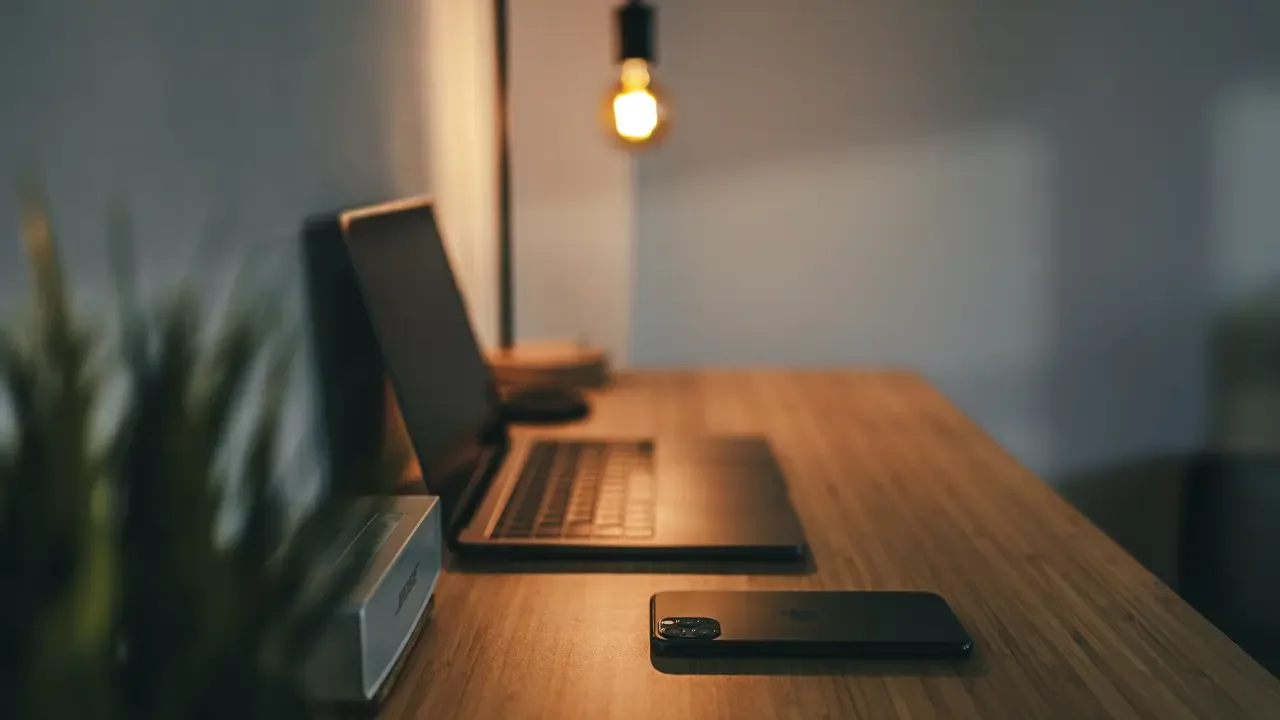
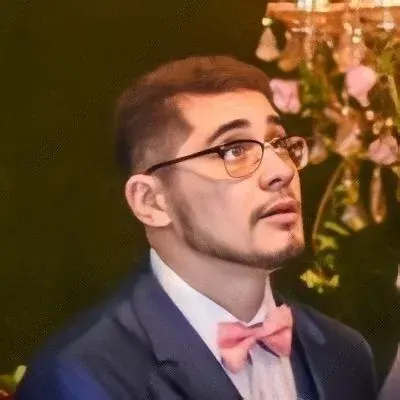
๐๐คCALL vs. APPLY: ๐งฉUnderstanding the Difference and When to Use Each ๐
Hey tech enthusiasts! ๐ In today's blog post, we're going to uncover the secrets behind the often-confusing duo of Function.prototype.apply()
and Function.prototype.call()
. ๐ค These two methods may seem similar, but trust me, there are some key differences you need to know about. Let's dive right in! ๐โโ๏ธ
๐ Similarities and Differences:
Both apply()
and call()
are used to invoke a function, but they differ in how arguments are passed to the function being called. ๐๐คทโโ๏ธ
๐น apply()
accepts an array-like object or an actual array as the second argument. Each element in the array represents an argument that will be passed to the function being called. For example:
const func = function(arg1, arg2) {
console.log(`${arg1} ${arg2}`);
};
const args = ['Hello', 'world!'];
func.apply(null, args); // Output: Hello world!
Note the use of null
as the first argument for apply()
. The first argument is the context in which the function should be called, but it's not relevant for this specific example so we can simply pass null
.
๐น On the other hand, call()
accepts multiple arguments directly, without wrapping them in an array. For example:
const func = function(arg1, arg2) {
console.log(`${arg1} ${arg2}`);
};
func.call(null, 'Hello', 'world!'); // Output: Hello world!
Just like with apply()
, the first argument for call()
is the context in which the function should be called.
๐ฏ Use Cases:
Now, you might be wondering when to use call()
over apply()
and vice versa. ๐ค Let me break it down for you:
๐น Use apply()
when you have an array-like object or an actual array containing the arguments you want to pass to the function. This is particularly useful when you don't know the number of arguments in advance or when the arguments are already gathered in an array-like structure. For example:
const numbers = [1, 2, 3, 4, 5];
const maxNumber = Math.max.apply(null, numbers); // Output: 5
In this case, apply()
allows us to pass the numbers
array directly as arguments to the Math.max()
function, which wouldn't be possible with call()
.
๐น On the other hand, use call()
when you know the number of arguments in advance and want to pass them individually. This is particularly useful when you have a fixed number of arguments or when each argument has a different meaning. For example:
const person = {
firstName: 'John',
lastName: 'Doe',
greet: function(greeting) {
console.log(`${greeting}, ${this.firstName} ${this.lastName}!`);
}
};
person.greet.call(person, 'Hola'); // Output: Hola, John Doe!
In this case, call()
allows us to pass the person
object as the context and the greeting as a separate argument.
๐ช Performance Considerations:
The performance difference between apply()
and call()
is negligible. Both methods provide similar results, but the choice between them depends on the specific use case, as we discussed earlier. So you can focus on choosing the right method without worrying about performance implications. ๐
๐ฃ Your Turn! ๐ฃ
Now that you know the difference between call()
and apply()
, it's time to put your newfound knowledge to the test! ๐ฌ Think of a scenario where you would use one method over the other and share it with us in the comments below. Let's start a discussion and learn from each other! ๐ฌ
That's it for today, folks! Stay tuned for more interesting tech insights and fun discussions. Don't forget to subscribe to our newsletter to never miss an update. Until next time, happy coding! ๐โจ