What is the correct way to check for string equality in JavaScript?
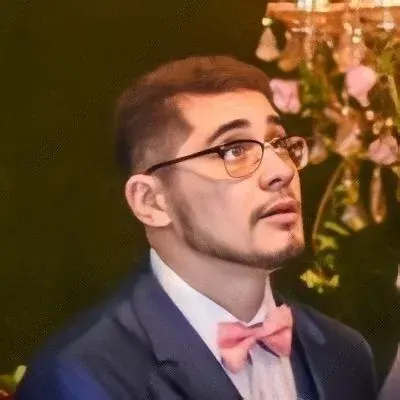
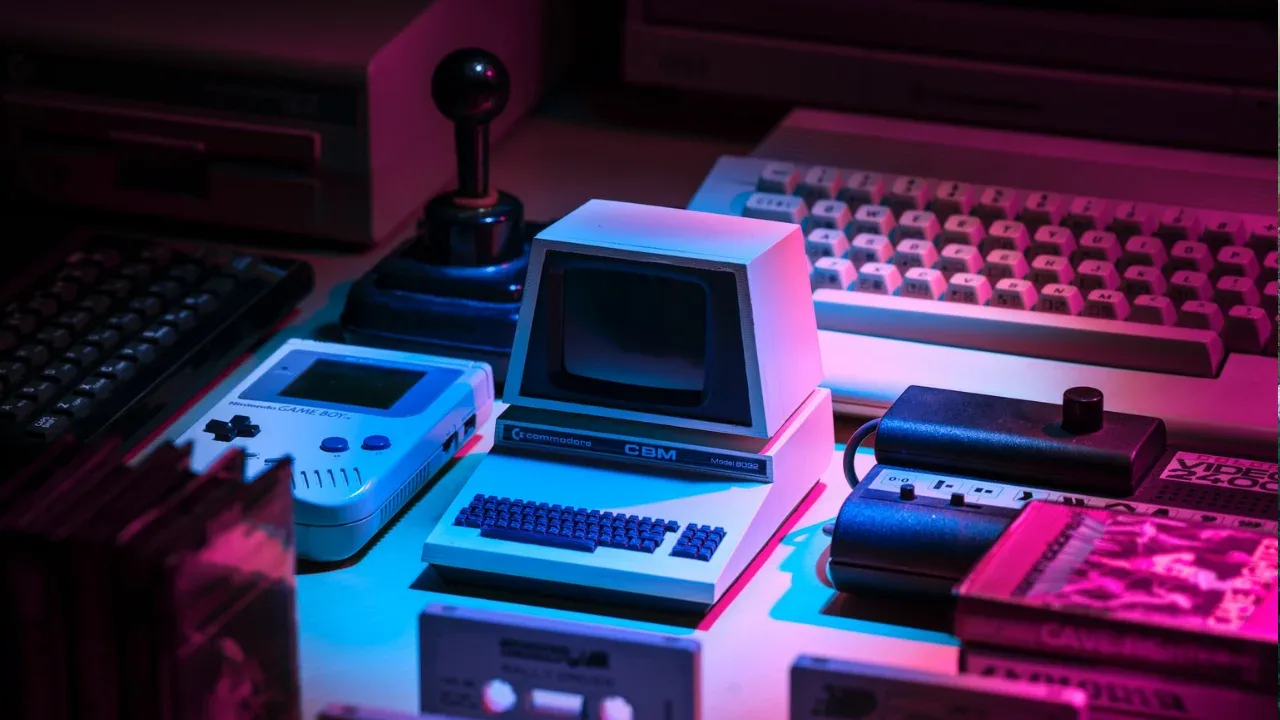
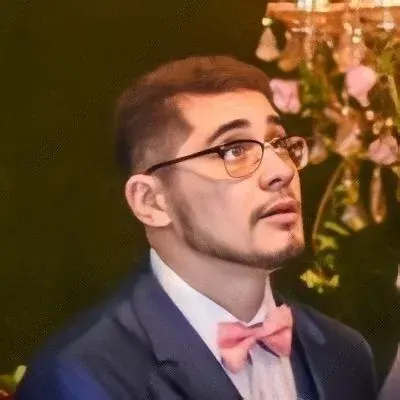
Easy Guide: How to Check for String Equality in JavaScript
š Hey there, JavaScript enthusiasts! šØāš» Welcome back to our tech blog. Today, we're going to tackle a common question that often confuses developers: What is the correct way to check for string equality in JavaScript? š¤š
The Common Issue: Inconsistent Comparison Results š
ā Before we dive into the solutions, let's address the problem. JavaScript provides us with multiple ways to check for string equality, such as the double equals (==
), triple equals (===
), and even the Object.is()
method. However, understanding how these comparison methods work is crucial to prevent unexpected results. š
š© The issue arises when using the double equals (==
) operator. It attempts type coercion to match the two operands, which can lead to inconsistent comparison results. Let's see an example:
console.log("5" == 5); // true
console.log("5" == [5]); // true
In both cases, the double equals (==
) operator returns true
, even though we have a string compared to a number and a string compared to an array. This can introduce hard-to-spot bugs into your code. š±š„
The Simple Solution: Triple Equals (===
)
š§ To avoid such inconsistencies, the best practice is to use the triple equals (===
) operator. This operator performs a strict equality check and does not coerce the types of the operands. Let's take a look:
console.log("5" === 5); // false
console.log("5" === [5]); // false
With the triple equals (===
), we get the expected results: both comparisons return false
. By using strict equality, we ensure that both the value and type of the operands are identical, guaranteeing accurate comparisons. šŖš
The Bonus Method: Object.is()
š "What about using the Object.is()
method?" you might ask. The Object.is()
method checks for strict equality, just like the triple equals (===
). However, there is a subtle difference when it comes to the treatment of certain special values such as NaN
and -0
.
console.log(Object.is(NaN, NaN)); // true
console.log(Object.is(-0, 0)); // false
In most cases, these differences won't impact your code. However, it's essential to be aware of how Object.is()
works to avoid any unexpected behavior in specific scenarios.
Call-to-Action: Your Turn to Engage! š¢š
š Now that we've shed some light on the correct way to check for string equality in JavaScript, it's time for you to practice and share your experiences. Let us know in the comments below which approach you prefer - triple equals (===
) or Object.is()
- and why! We'd love to hear your thoughts and insights. š¤š¬
š” Share this post with your fellow JavaScript developers so that they too can understand how to avoid comparison pitfalls and ensure accurate string equality checks. Together, we can elevate our coding practices and build more robust applications! šāØ
That's all for today, folks! We'll be back soon with more informative tech guides. Until then, happy coding! š»š