What is the best way to add options to a select from a JavaScript object with jQuery?
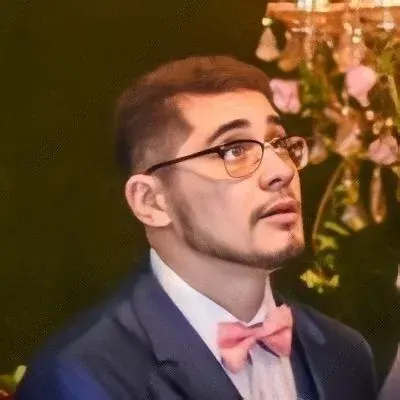
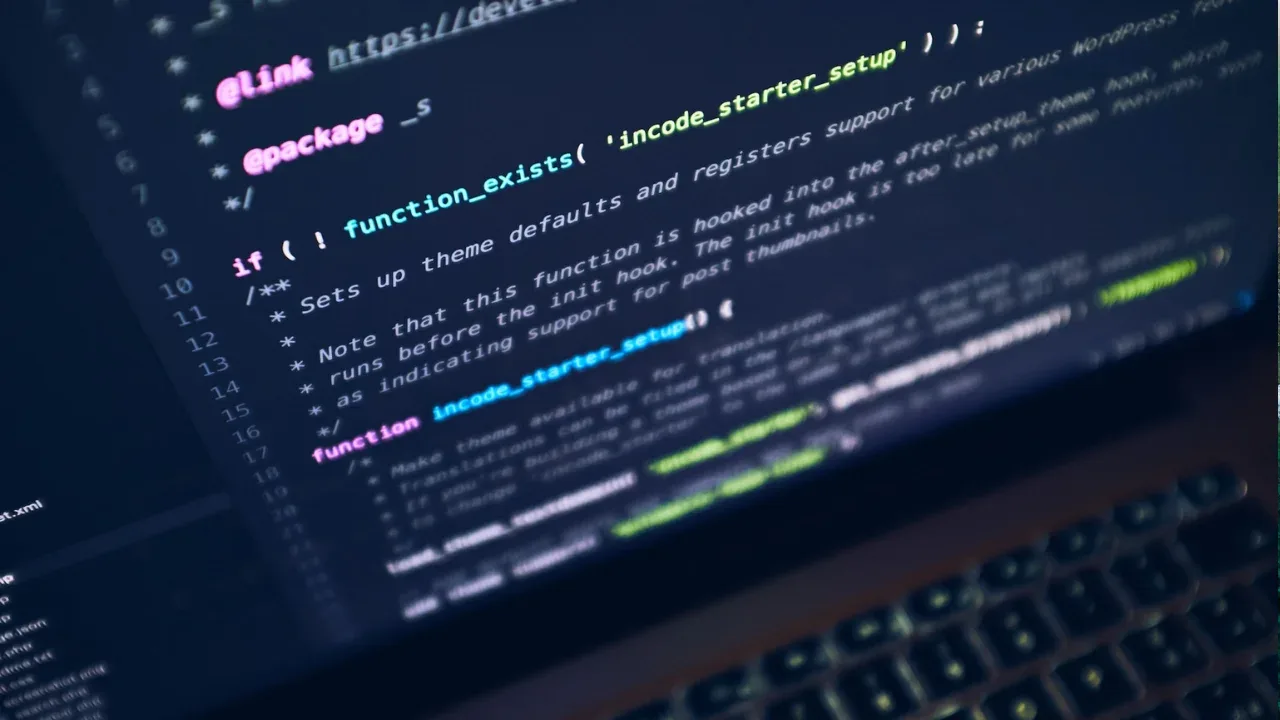
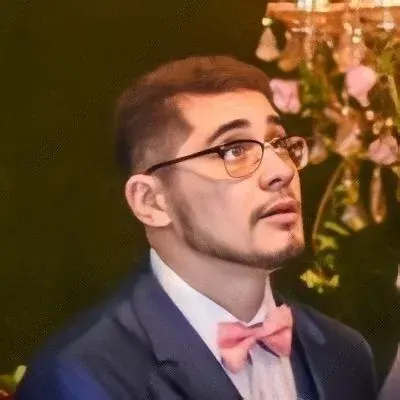
🌟 Easy and Effective Solution to Add Options to a Select Element using JavaScript Object and jQuery 🌟
If you've ever wondered how to add options to a <select>
element dynamically using a JavaScript object with jQuery, you're in the right place! In this blog post, I'll guide you through a simple and efficient solution to tackle this common issue. Let's dive in! 🏊♂️
Understanding the Problem 🤔
Consider the scenario where you have a JavaScript object called selectValues
that contains key-value pairs representing the options you want to add to your <select>
element. Here's an example:
selectValues = { "1": "test 1", "2": "test 2" };
Now, the challenge is to programmatically append these options to your <select>
element using jQuery, without the need for a plugin.
The Not-so-Optimal Approach 😕
The code snippet you shared is a common approach to solve this problem. However, it can be improved for better readability and simplicity. Let's take a look at it:
for (key in selectValues) {
if (typeof (selectValues[key] == 'string') {
$('#mySelect').append('<option value="' + key + '">' + selectValues[key] + '</option>');
}
}
While this code works, it can be a bit convoluted. Plus, it has a small error in the typeof
check (the closing parenthesis is placed incorrectly). Fear not! I have a cleaner and simpler solution for you! 🎉
A Clean and Simple Solution ✨
You can achieve the same outcome in a much neater way using the $.each()
function in jQuery. Here's how you can refactor your code:
$.each(selectValues, function(key, value) {
$('#mySelect')
.append($('<option>', { value : key })
.text(value));
});
Let's understand the key differences in this solution:
Instead of using a
for...in
loop, we utilize the$.each()
function provided by jQuery. It iterates over each key-value pair in theselectValues
object.Inside the iteration, we create a new
<option>
element using$(<option>)
and pass thevalue
key andtext
value as arguments. This creates an<option>
tag with the desired attribute and inner text.Finally, we append the newly created
<option>
element to the<select>
element with the id#mySelect
using the.append()
method.
A Call-to-Action for Your Input! 🙌
Now that you have a simple and effective solution to add options to a <select>
element using a JavaScript object and jQuery, I would love to hear from you! Do you have any other unique approaches or insights on this topic? Share your thoughts in the comments below! Let's learn from each other and make our code even better! 🚀
Remember, simplicity and readability are key when it comes to writing maintainable code. So next time you find yourself in need of dynamically populating options in a <select>
element, give this elegant solution a try!
Happy coding! 😄