What does "use strict" do in JavaScript, and what is the reasoning behind it?
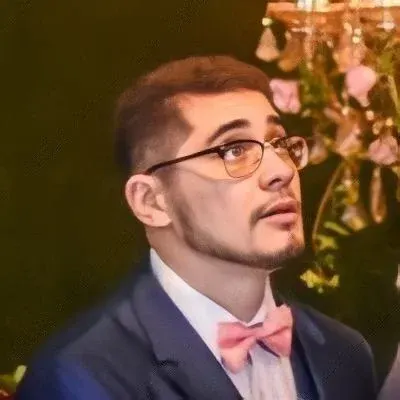
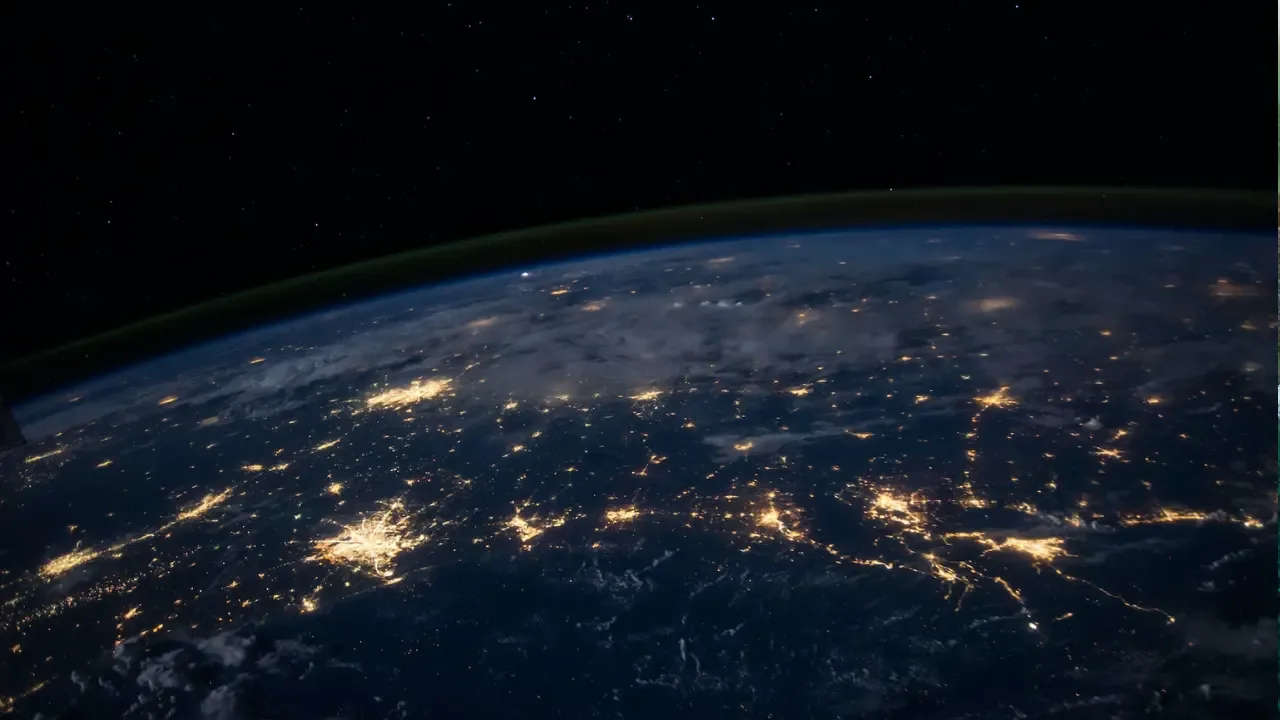
Understanding "use strict" in JavaScript
If you've ever encountered the error message "Missing 'use strict' statement" while running your JavaScript code through a linter or validator, you might have wondered what exactly this statement does and why it is necessary. In this blog post, we'll dive into the concept of "use strict" in JavaScript, explore its implications, and determine its relevance in the current browser landscape.
What is "use strict"?
"Use strict" is a special string literal that you can include at the top of your JavaScript code (usually within the global scope) to enable strict mode. When strict mode is enabled, the JavaScript interpreter applies stricter rules and enforces better coding practices, helping you write cleaner and more reliable code.
By default, JavaScript is a forgiving language that allows flexible syntax and provides automatic conversions for certain operations. However, these loose rules sometimes lead to unexpected behavior and can make it harder to catch errors. Strict mode aims to mitigate these issues by introducing additional constraints and throwing errors for potentially problematic code patterns.
What does strict mode imply?
Strict mode affects the entire script or a specific function, depending on where you include the "use strict" statement. Here are some key aspects of how strict mode alters the behavior of your JavaScript code:
Variable declaration: In strict mode, you must explicitly declare variables using
var
,let
, orconst
before using them. This helps prevent accidental global variable creation and enforces better scoping practices.Example:
// Without strict mode (non-strict): x = 42; // No explicit declaration, creates a global variable // With strict mode: "use strict"; let y = 42; // Explicit declaration using 'let'
No undeclared variables: In strict mode, referencing variables that have not been declared will result in an error. This catches typos and other potential bugs early on.
Example:
// Without strict mode (non-strict): z = 42; // No error, creates a global variable even with a typo // With strict mode: "use strict"; a = 42; // ReferenceError: a is not defined
Eliminates unsafe practices: Strict mode prohibits certain unsafe practices, such as deleting variables, functions, or function arguments. It also prevents the use of duplicate parameter names in functions.
Example:
// Without strict mode (non-strict): delete x; // No error, deletes x variable function sum(a, a) {} // No error, duplicate parameter names // With strict mode: "use strict"; delete y; // SyntaxError: Delete of an unqualified identifier function multiply(b, b) {} // SyntaxError: Duplicate parameter name not allowed
**Changes the behavior of
this
: In strict mode, the value ofthis
inside a function that is not a method or constructor will beundefined
, instead of referring to the global object.Example:
// Without strict mode (non-strict): function printThis() { console.log(this); } printThis(); // 'this' refers to the global object (e.g., 'window' in browsers) // With strict mode: "use strict"; function printThisStrict() { console.log(this); } printThisStrict(); // 'this' is 'undefined'
Is "use strict" still relevant?
Absolutely! The use of strict mode is highly recommended, even in modern JavaScript development. While strict mode may not catch all errors, it helps secure your code, reduces the likelihood of bugs, and promotes better coding practices. Additionally, strict mode ensures better compatibility with future versions of JavaScript, as new features may rely on stricter rules.
Enabling strict mode
To enable strict mode, simply add the statement "use strict";
at the beginning of your JavaScript file or function:
"use strict";
// Your code here...
Conclusion
"Use strict" in JavaScript enables strict mode, which imposes stricter rules and enhances coding practices. By explicitly declaring variables, catching undeclared variables early on, eliminating unsafe practices, and changing the behavior of this
, strict mode helps improve code quality and ensures better compatibility. So next time you encounter the "Missing 'use strict' statement" error, embrace it and make your JavaScript code more reliable.
Do you regularly use strict mode in your JavaScript projects? Share your experiences and best practices in the comments below! Let's code smarter together. 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
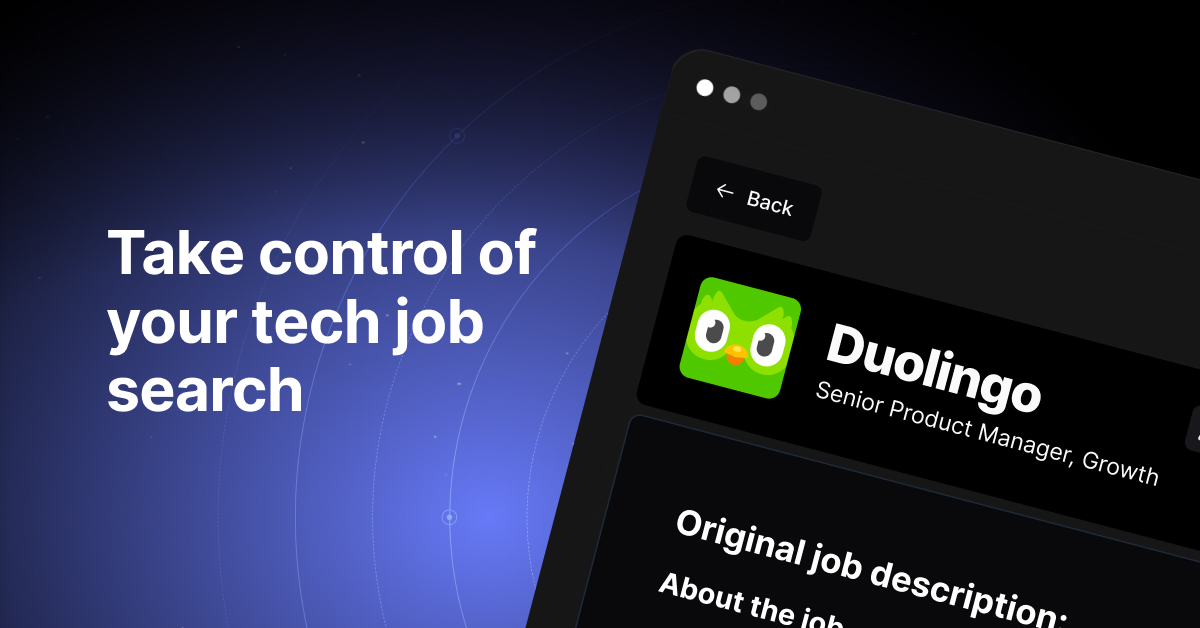