What does "export default" do in JSX?
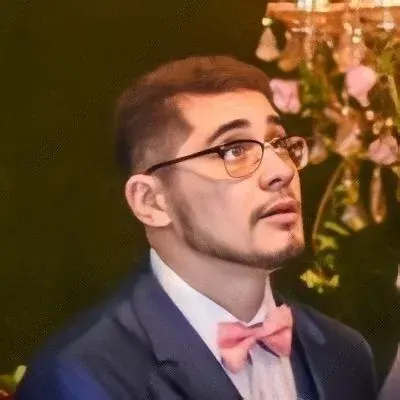
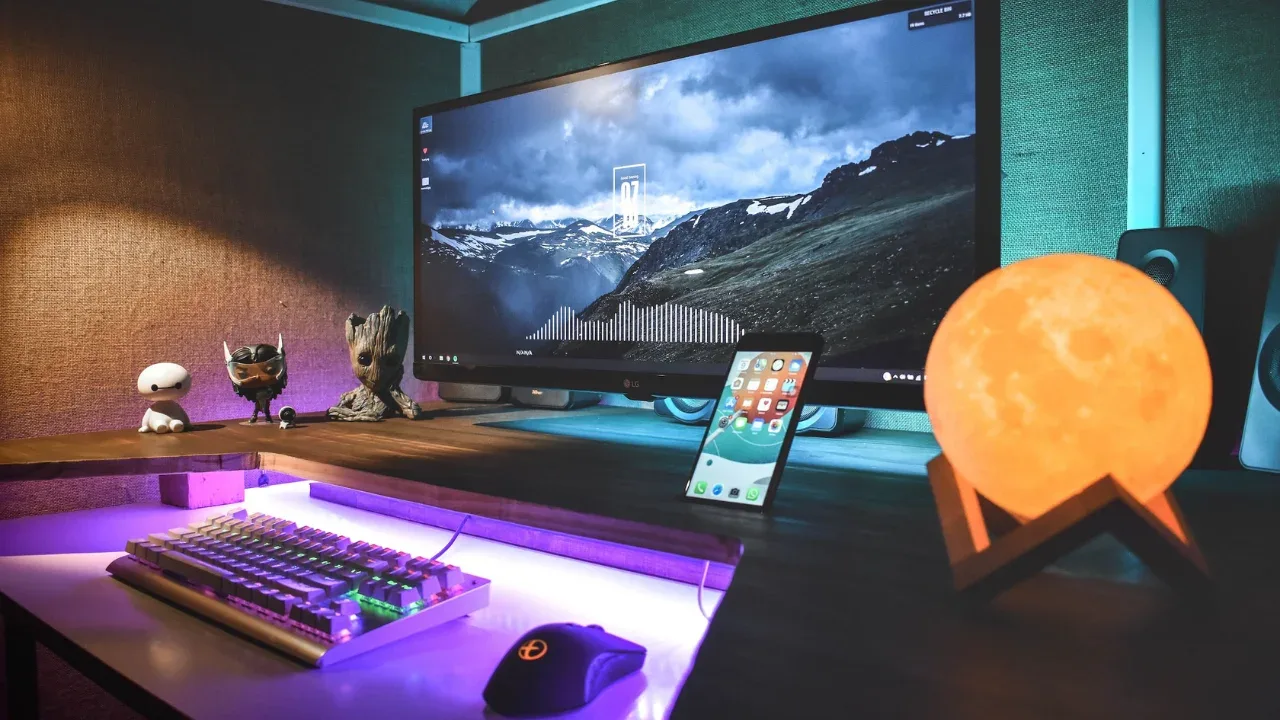
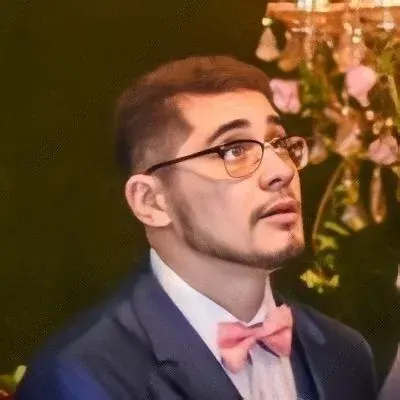
📝🔥 Tech Blog: Understanding "export default" in JSX
Have you ever come across the phrase "export default" in JSX and wondered what it does? 🤔 Don't worry, you're not alone! In this blog post, we'll dive into this concept, address common issues related to it, and provide easy solutions to help you understand and use it effectively. So, let's get started! 💪🚀
What is "export default"? 🤔
In the code snippet you provided, there's a class component called HelloWorld
, and it is being exported using the statement export default HelloWorld;
. So, what exactly does this mean?
In React, components are the building blocks of an application's UI. They allow you to create reusable and modular pieces of code. When you define a component in your JSX file, you often need to make it available for use in other files or components. This is where the "export default" statement comes into play.
The "export default" statement is used to export a single value (often a component) from a file. It tells the module system (e.g., Webpack or Babel) that this is the main entity that should be imported when using this file in other parts of your application.
In our example, the HelloWorld
component is being exported as the default export from the file hello-world.jsx
. This means that when this file is imported elsewhere, the imported value will be the HelloWorld
component itself.
Common Issues and Easy Solutions 💡
Let's address a couple of common issues related to "export default" and provide some easy solutions:
1. Issue: "export default" is not working when importing the component in another file.
Solution: Double-check the import statement in the consuming file. Ensure that you are using the correct file/path name and that you are importing the default export specifically. For example:
// Correct way to import the default export
import HelloWorld from './hello-world';
// Incorrect way (without specifying default export)
import { HelloWorld } from './hello-world';
2. Issue: Exporting multiple components from a file using "export default"
Solution: Since "export default" is used to export a single value, you cannot export multiple components using this statement alone. Instead, you can define multiple components and export them individually, like this:
// hello-world.jsx
export class FirstComponent extends React.Component { ... }
export class SecondComponent extends React.Component { ... }
Then, you can import them separately in other files using named imports.
📣 Now that you understand "export default" in JSX, you can confidently use it in your React applications! If you found this blog post helpful, don't forget to share it with your fellow developers. And remember, always keep exploring and expanding your React skills! Happy coding! 🎉💻
👉 What other React-related topics would you like us to cover? Share your thoughts in the comments below! Let's learn and grow together. 🌟