What do multiple arrow functions mean in JavaScript?
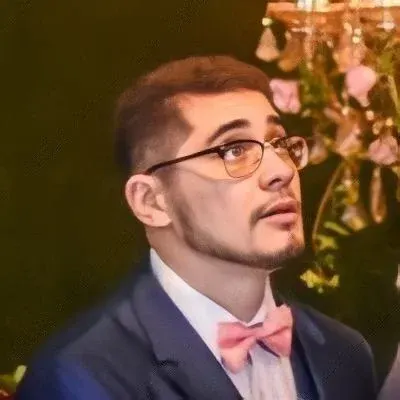
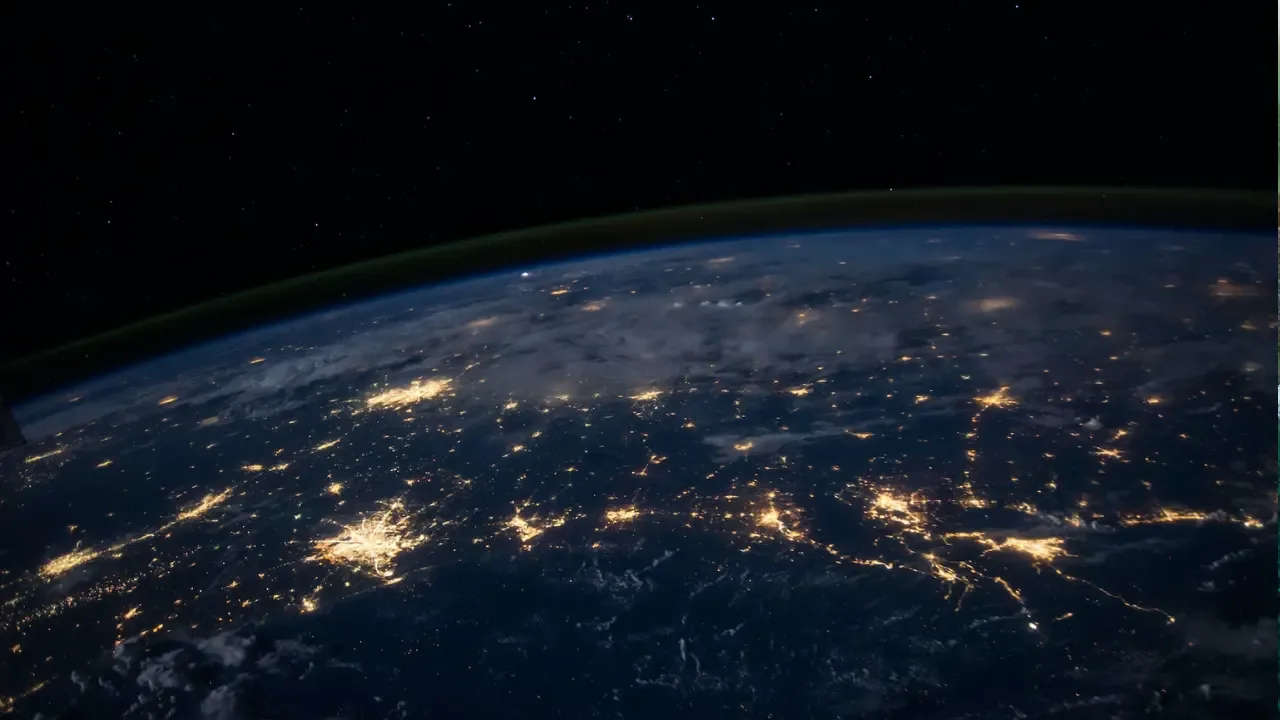
Understanding Multiple Arrow Functions in JavaScript 👨💻🏹
Have you ever come across code that utilizes multiple arrow functions in JavaScript, like the one mentioned above? It can be confusing at first, but fear not! In this blog post, we'll dive into the world of multiple arrow functions and demystify their purpose and functionality. So grab your coding hat and let's get started! 🎩💻
Why Do We Use Multiple Arrow Functions? 🤔
In JavaScript, arrow functions offer a concise syntax and lexical scoping compared to traditional function expressions. They're especially useful in frameworks like React, where we often encounter complex event handlers or callback functions.
The example you provided demonstrates the use of multiple arrow functions within a React component. Let's break it down:
handleChange = field => e => {
e.preventDefault();
/// Do something here
}
Understanding the Syntax 🧩
In this code snippet, handleChange
is being assigned a function that takes a field
parameter. Instead of returning a value directly, it returns another arrow function with e
as its parameter. This inner arrow function serves as the event handler for some event.
The outer arrow function is responsible for receiving the field
argument and returning the inner arrow function. This allows the event handler to have access to both the field
parameter and the event object (e
).
Practical Use Cases 🛠️
Now that we understand the syntax, let's explore some practical use cases where multiple arrow functions prove beneficial:
1. Event Handling 🎉
In React, event handlers often require additional parameters. By using multiple arrow functions, we can pass in these parameters explicitly while still having access to the event object. This simplifies the process of handling events and allows for cleaner code.
handleChange = field => e => {
e.preventDefault();
// Do something with field and event
}
2. Currying 📦
Currying is a functional programming technique that allows us to transform a function with multiple arguments into a sequence of functions, each taking one argument. Multiple arrow functions are excellent for implementing currying in JavaScript.
const add = x => y => x + y;
const addTwo = add(2);
console.log(addTwo(5)); // Output: 7
By creating two arrow function expressions, add
becomes a higher-order function that can be partially applied. We create an addTwo
function that adds 2
to any given value. This technique provides flexibility and reusability in our code.
Take It to the Next Level 🚀
Now that you've gained a solid understanding of multiple arrow functions in JavaScript, it's time to put this knowledge into action! Start experimenting with them in your own code and make your functions more efficient and expressive. 💪🚀
If you still have any questions or want to share your experiences with arrow functions, we'd love to hear from you in the comments below! Let's keep the conversation going. 🗣️💬
Happy coding! 🎉💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
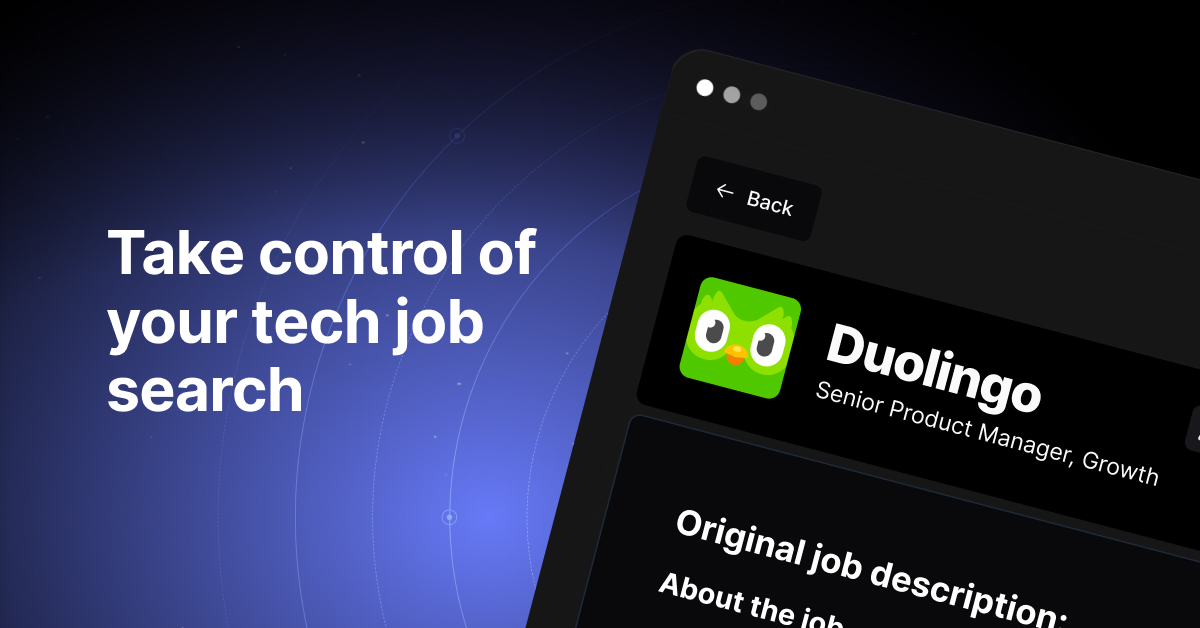