Way to ng-repeat defined number of times instead of repeating over array?
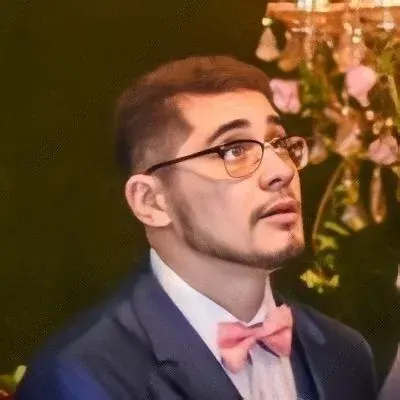
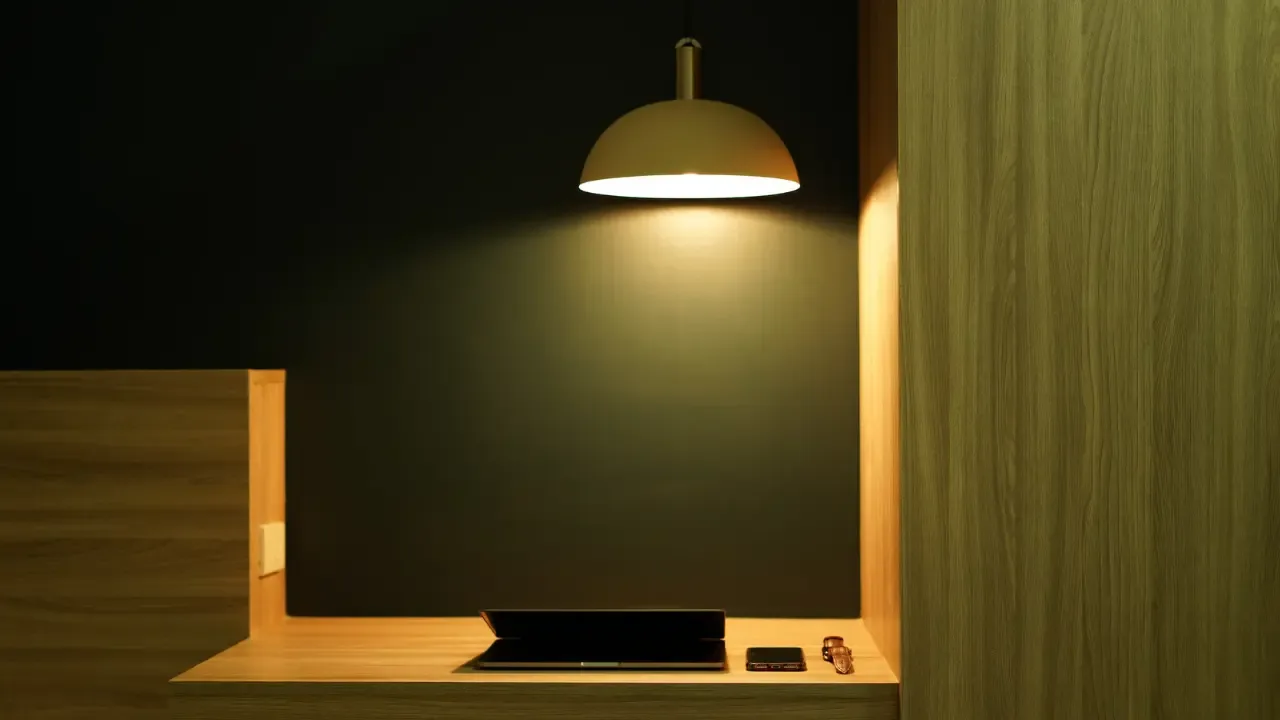
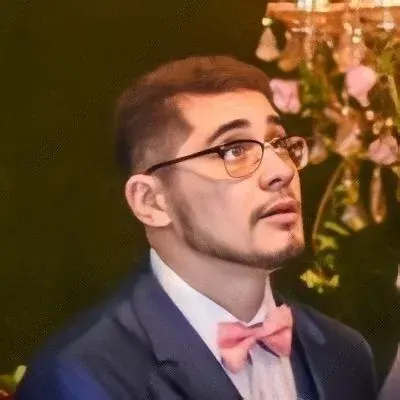
How to ng-repeat a Defined Number of Times Instead of Repeating Over an Array? 🔄
<p>🤔 Have you ever wondered if there's a way to use <code>ng-repeat</code> to display a list a specific number of times, without having to iterate over an array? Look no further – we have the solution for you!</p>
The Problem 😫
<p>Let's say you want to display a list, with each list item incrementing by 1, for a defined number of times. However, the traditional usage of <code>ng-repeat</code> requires an array to iterate over.</p>
The Desired Result 🎯
<p>Take a look at the desired result:</p>
<pre><code><ul> <li><span>1</span></li> <li><span>2</span></li> <li><span>3</span></li> <li><span>4</span></li> <li><span>5</span></li> </ul> </code></pre>
The Solution 💡
<p>To achieve this desired result, we can use the <code>ng-repeat</code> directive with a trick using the <code>Array</code> constructor and the <code>$index</code> property.</p>
<p>Here's how you can do it:</p>
<ul>
<li ng-repeat="i in Array(number).fill().map((_, index) => index + 1)">
<span>{{ i }}</span>
</li>
</ul>
<p>Explanation:</p> <ul> <li>We create an <code>Array</code> of a defined length by passing the <code>number</code> variable to the <code>Array</code> constructor.</li> <li>We then use the <code>fill()</code> method to populate the array with undefined values.</li> <li>Next, we use the <code>map()</code> method to incrementally fill the array with values from 1 up to the defined number. We achieve this by using an arrow function that takes the index as a parameter and returns the incremented value.</li> <li>Finally, we use <code>{{ i }}</code> to display each number as a list item.</li> </ul>
Your Turn! ✏️
<p>Now you have the power to repeat a specific number of times with ease. Go ahead and put this solution into practice in your Angular app!</p>
<p>Remember to get creative and adapt this solution to your specific needs. Share your results or any issues you encountered in the comments below. We'd love to hear from you! 😊</p>