Watch multiple $scope attributes
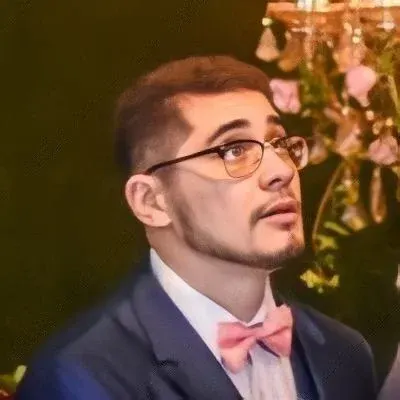
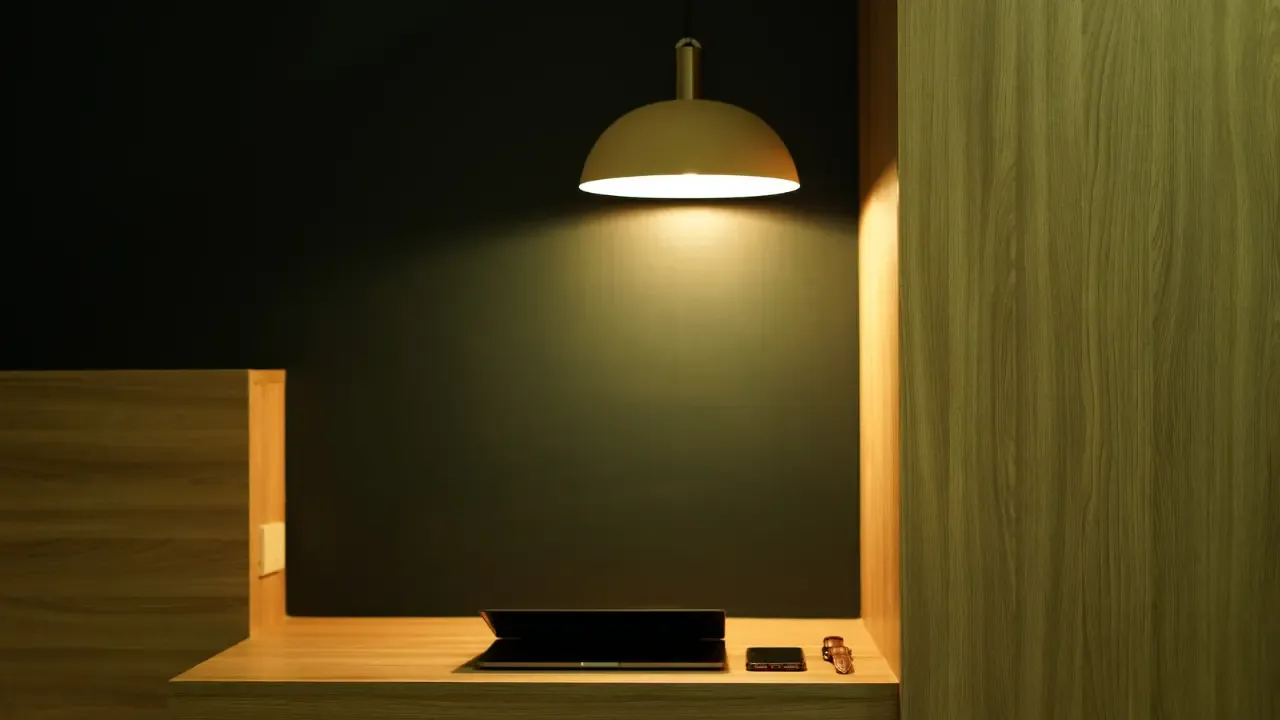
🤔 Watching Multiple $scope
Attributes? Here's How to Make it Work! 🙌
If you're a frontend developer working with AngularJS, you might have come across the need to watch multiple $scope
attributes for changes. Maybe you have a scenario where you need to react to changes in both item1
and item2
at the same time. But is it possible to achieve this with the $watch
function? 🤔
📝 Understanding the Problem
The code snippet you shared hints at the possibility of watching multiple $scope
attributes using $watch
. However, the syntax you provided is not correct. $watch
can watch only one attribute at a time. 😕 Don't worry! We're always up for a good challenge, and we have a couple of solutions up our sleeves. Let's dive in!
💡 Solution 1: Watching One Attribute with a Callback Function
A straightforward approach is to watch each attribute independently and provide a callback function that triggers when any of the attributes change. Here's how it can be done:
$scope.$watch('item1', function() {
// Do something when item1 changes
});
$scope.$watch('item2', function() {
// Do something when item2 changes
});
With this solution, you can perform separate actions when either item1
or item2
changes. However, if you have similar tasks to perform when both attributes change, it's not the most efficient solution.
💪 Solution 2: Using a Function to Observe Multiple Attributes
Sometimes, you want to consolidate the logic and react when multiple attributes are modified. For this scenario, you can take advantage of the $watchGroup
function. Here's how it works:
$scope.$watchGroup(['item1', 'item2'], function(newValues, oldValues) {
// newValues and oldValues are arrays containing the new and old values of the watched attributes respectively
// Perform actions based on the changes in item1 and item2
});
By using $watchGroup
, you can observe multiple attributes and execute a callback function when any of them change. This allows you to handle tasks that depend on both item1
and item2
in a single place.
🔥 Call-to-Action: Engage and Learn More
Now that you know how to watch multiple $scope
attributes, it's time to put this knowledge into practice! 🚀 Experiment with the solutions we provided and see how you can apply them to your own projects. Don't hesitate to share your experiences or ask questions in the comments section below. Let's learn and grow together as a community! 👩💻👨💻
Remember, frontend development can be challenging, but with the right mindset and a little guidance, every problem can be surmountable! 🌟
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
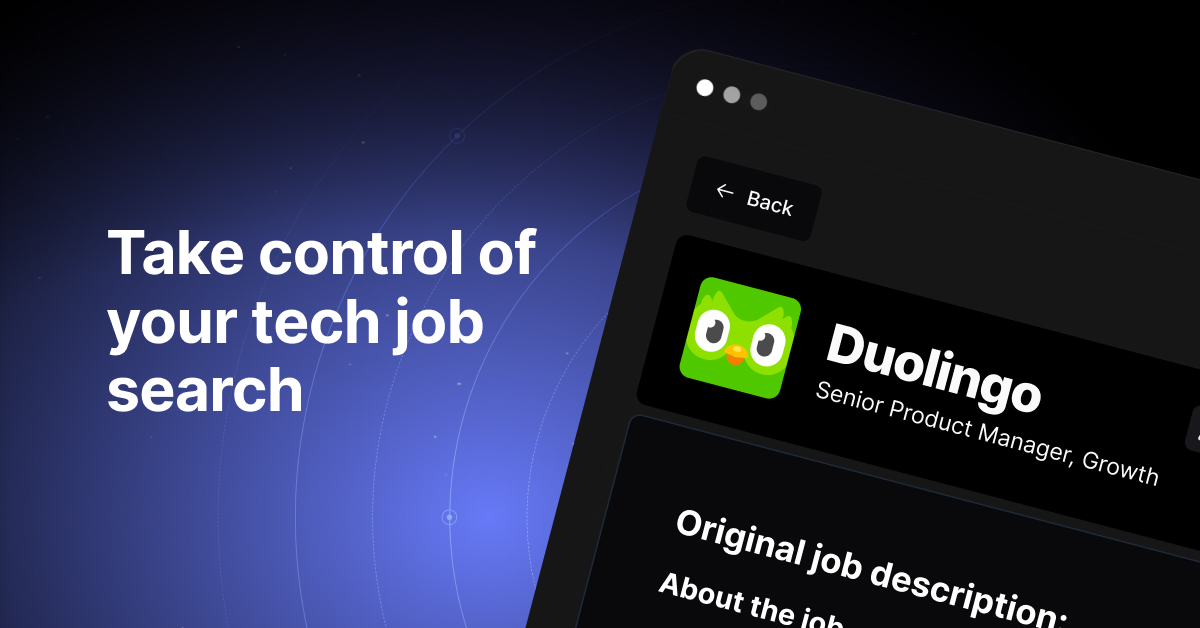